mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
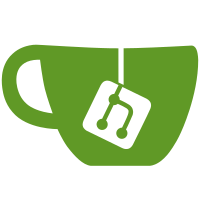
When multiple log() calls are used which don't end in newline, the log prefix is prepended multiple times in the same line. This makes the output look strange. Fix this by detecting when the previous log record did not end in newline. In that case, setting a flag. Drop the unused BUFFSIZE in the test while we are here. As an example implementation, update log_console to check the flag and produce the expected output. Signed-off-by: Simon Glass <sjg@chromium.org>
54 lines
1.3 KiB
C
54 lines
1.3 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Logging support
|
|
*
|
|
* Copyright (c) 2017 Google, Inc
|
|
* Written by Simon Glass <sjg@chromium.org>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <log.h>
|
|
#include <asm/global_data.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
static int log_console_emit(struct log_device *ldev, struct log_rec *rec)
|
|
{
|
|
int fmt = gd->log_fmt;
|
|
bool add_space = false;
|
|
|
|
/*
|
|
* The output format is designed to give someone a fighting chance of
|
|
* figuring out which field is which:
|
|
* - level is in CAPS
|
|
* - cat is lower case and ends with comma
|
|
* - file normally has a .c extension and ends with a colon
|
|
* - line is integer and ends with a -
|
|
* - function is an identifier and ends with ()
|
|
* - message has a space before it unless it is on its own
|
|
*/
|
|
if (!(rec->flags & LOGRECF_CONT) && fmt != BIT(LOGF_MSG)) {
|
|
add_space = true;
|
|
if (fmt & BIT(LOGF_LEVEL))
|
|
printf("%s.", log_get_level_name(rec->level));
|
|
if (fmt & BIT(LOGF_CAT))
|
|
printf("%s,", log_get_cat_name(rec->cat));
|
|
if (fmt & BIT(LOGF_FILE))
|
|
printf("%s:", rec->file);
|
|
if (fmt & BIT(LOGF_LINE))
|
|
printf("%d-", rec->line);
|
|
if (fmt & BIT(LOGF_FUNC))
|
|
printf("%s()", rec->func);
|
|
}
|
|
if (fmt & BIT(LOGF_MSG))
|
|
printf("%s%s", add_space ? " " : "", rec->msg);
|
|
|
|
return 0;
|
|
}
|
|
|
|
LOG_DRIVER(console) = {
|
|
.name = "console",
|
|
.emit = log_console_emit,
|
|
.flags = LOGDF_ENABLE,
|
|
};
|