mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
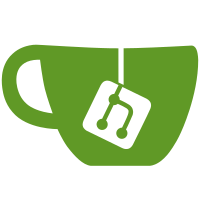
In some cases it is useful to include a U-Boot environment region in an image. This allows the board to start up with an environment ready to go. Add a new entry type for this. The input is a text file containing the environment entries, one per line, in the format: var=value Signed-off-by: Simon Glass <sjg@chromium.org> Acked-by: Andy Shevchenko <andriy.shevchenko@linux.intel.com>
43 lines
1.3 KiB
Python
43 lines
1.3 KiB
Python
# SPDX-License-Identifier: GPL-2.0+
|
|
# Copyright (c) 2018 Google, Inc
|
|
# Written by Simon Glass <sjg@chromium.org>
|
|
#
|
|
|
|
import struct
|
|
import zlib
|
|
|
|
from binman.etype.blob import Entry_blob
|
|
from dtoc import fdt_util
|
|
from patman import tools
|
|
|
|
class Entry_u_boot_env(Entry_blob):
|
|
"""An entry which contains a U-Boot environment
|
|
|
|
Properties / Entry arguments:
|
|
- filename: File containing the environment text, with each line in the
|
|
form var=value
|
|
"""
|
|
def __init__(self, section, etype, node):
|
|
super().__init__(section, etype, node)
|
|
|
|
def ReadNode(self):
|
|
super().ReadNode()
|
|
if self.size is None:
|
|
self.Raise("'u-boot-env' entry must have a size property")
|
|
self.fill_value = fdt_util.GetByte(self._node, 'fill-byte', 0)
|
|
|
|
def ReadBlobContents(self):
|
|
indata = tools.ReadFile(self._pathname)
|
|
data = b''
|
|
for line in indata.splitlines():
|
|
data += line + b'\0'
|
|
data += b'\0';
|
|
pad = self.size - len(data) - 5
|
|
if pad < 0:
|
|
self.Raise("'u-boot-env' entry too small to hold data (need %#x more bytes)" % -pad)
|
|
data += tools.GetBytes(self.fill_value, pad)
|
|
crc = zlib.crc32(data)
|
|
buf = struct.pack('<I', crc) + b'\x01' + data
|
|
self.SetContents(buf)
|
|
return True
|