mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
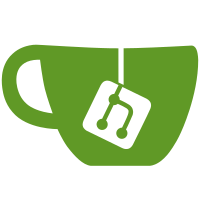
Add a dm_ prefix to driver model I2C functions so that we can keep the old ones around. This is a little unfortunate, but on reflection it is too difficult to change the API. We can undo this rename when most boards and drivers are converted to use driver model for I2C. Signed-off-by: Simon Glass <sjg@chromium.org>
112 lines
2.9 KiB
C
112 lines
2.9 KiB
C
/*
|
|
* Copyright (c) 2010-2013, NVIDIA CORPORATION. All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify it
|
|
* under the terms and conditions of the GNU General Public License,
|
|
* version 2, as published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope it will be useful, but WITHOUT
|
|
* ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or
|
|
* FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for
|
|
* more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program. If not, see <http://www.gnu.org/licenses/>.
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <dm.h>
|
|
#include <asm/arch/pinmux.h>
|
|
#include <asm/arch/gp_padctrl.h>
|
|
#include "pinmux-config-dalmore.h"
|
|
#include <i2c.h>
|
|
|
|
#define BAT_I2C_ADDRESS 0x48 /* TPS65090 charger */
|
|
#define PMU_I2C_ADDRESS 0x58 /* TPS65913 PMU */
|
|
|
|
/*
|
|
* Routine: pinmux_init
|
|
* Description: Do individual peripheral pinmux configs
|
|
*/
|
|
void pinmux_init(void)
|
|
{
|
|
pinmux_config_pingrp_table(tegra114_pinmux_set_nontristate,
|
|
ARRAY_SIZE(tegra114_pinmux_set_nontristate));
|
|
|
|
pinmux_config_pingrp_table(tegra114_pinmux_common,
|
|
ARRAY_SIZE(tegra114_pinmux_common));
|
|
|
|
pinmux_config_pingrp_table(unused_pins_lowpower,
|
|
ARRAY_SIZE(unused_pins_lowpower));
|
|
|
|
/* Initialize any non-default pad configs (APB_MISC_GP regs) */
|
|
pinmux_config_drvgrp_table(dalmore_padctrl,
|
|
ARRAY_SIZE(dalmore_padctrl));
|
|
}
|
|
|
|
#if defined(CONFIG_TEGRA_MMC)
|
|
/*
|
|
* Do I2C/PMU writes to bring up SD card bus power
|
|
*
|
|
*/
|
|
void board_sdmmc_voltage_init(void)
|
|
{
|
|
struct udevice *dev;
|
|
uchar reg, data_buffer[1];
|
|
int ret;
|
|
|
|
ret = i2c_get_chip_for_busnum(0, PMU_I2C_ADDRESS, &dev);
|
|
if (ret) {
|
|
debug("%s: Cannot find PMIC I2C chip\n", __func__);
|
|
return;
|
|
}
|
|
|
|
/* TPS65913: LDO9_VOLTAGE = 3.3V */
|
|
data_buffer[0] = 0x31;
|
|
reg = 0x61;
|
|
|
|
ret = dm_i2c_write(dev, reg, data_buffer, 1);
|
|
if (ret)
|
|
printf("%s: PMU i2c_write %02X<-%02X returned %d\n",
|
|
__func__, reg, data_buffer[0], ret);
|
|
|
|
/* TPS65913: LDO9_CTRL = Active */
|
|
data_buffer[0] = 0x01;
|
|
reg = 0x60;
|
|
|
|
ret = dm_i2c_write(dev, reg, data_buffer, 1);
|
|
if (ret)
|
|
printf("%s: PMU i2c_write %02X<-%02X returned %d\n",
|
|
__func__, reg, data_buffer[0], ret);
|
|
|
|
/* TPS65090: FET6_CTRL = enable output auto discharge, enable FET6 */
|
|
data_buffer[0] = 0x03;
|
|
reg = 0x14;
|
|
|
|
ret = i2c_get_chip_for_busnum(0, BAT_I2C_ADDRESS, &dev);
|
|
if (ret) {
|
|
debug("%s: Cannot find charger I2C chip\n", __func__);
|
|
return;
|
|
}
|
|
ret = dm_i2c_write(dev, reg, data_buffer, 1);
|
|
if (ret)
|
|
printf("%s: BAT i2c_write %02X<-%02X returned %d\n",
|
|
__func__, reg, data_buffer[0], ret);
|
|
}
|
|
|
|
/*
|
|
* Routine: pin_mux_mmc
|
|
* Description: setup the MMC muxes, power rails, etc.
|
|
*/
|
|
void pin_mux_mmc(void)
|
|
{
|
|
/*
|
|
* NOTE: We don't do mmc-specific pin muxes here.
|
|
* They were done globally in pinmux_init().
|
|
*/
|
|
|
|
/* Bring up the SDIO3 power rail */
|
|
board_sdmmc_voltage_init();
|
|
}
|
|
#endif /* MMC */
|