mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-18 17:18:01 +09:00
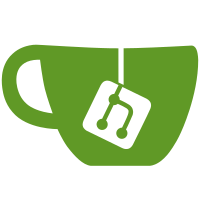
This introduces OMAP3 support for the common omap boot code, as well as a major cleanup of the common omap boot code. First, the omap_boot_parameters structure becomes platform-specific, since its definition differs a bit across omap platforms. The offsets are removed as well since it is U-Boot's coding style to use structures for mapping such kind of data (in the sense that it is similar to registers). It is correct to assume that romcode structure encoding is the same as U-Boot, given the description of these structures in the TRMs. The original address provided by the bootrom is passed to the U-Boot binary instead of a duplicate of the structure stored in global data. This allows to have only the relevant (boot device and mode) information stored in global data. It is also expected that the address where the bootrom stores that information is not overridden by the U-Boot SPL or U-Boot. The save_omap_boot_params is expected to handle all special cases where the data provided by the bootrom cannot be used as-is, so that spl_boot_device and spl_boot_mode only return the data from global data. All of this is only relevant when the U-Boot SPL is used. In cases it is not, save_boot_params should fallback to its weak (or board-specific) definition. save_omap_boot_params should not be called in that context either. Signed-off-by: Paul Kocialkowski <contact@paulk.fr>
73 lines
1.9 KiB
C
73 lines
1.9 KiB
C
/*
|
|
* (C) Copyright 2014
|
|
* Texas Instruments, <www.ti.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
#ifndef _TI_COMMON_SYS_PROTO_H_
|
|
#define _TI_COMMON_SYS_PROTO_H_
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
#ifdef CONFIG_OMAP_COMMON
|
|
#define TI_ARMV7_DRAM_ADDR_SPACE_START 0x80000000
|
|
#define TI_ARMV7_DRAM_ADDR_SPACE_END 0xFFFFFFFF
|
|
|
|
#define OMAP_INIT_CONTEXT_SPL 0
|
|
#define OMAP_INIT_CONTEXT_UBOOT_FROM_NOR 1
|
|
#define OMAP_INIT_CONTEXT_UBOOT_AFTER_SPL 2
|
|
#define OMAP_INIT_CONTEXT_UBOOT_AFTER_CH 3
|
|
|
|
static inline u32 running_from_sdram(void)
|
|
{
|
|
u32 pc;
|
|
asm volatile ("mov %0, pc" : "=r" (pc));
|
|
return ((pc >= TI_ARMV7_DRAM_ADDR_SPACE_START) &&
|
|
(pc < TI_ARMV7_DRAM_ADDR_SPACE_END));
|
|
}
|
|
|
|
static inline u8 uboot_loaded_by_spl(void)
|
|
{
|
|
/*
|
|
* u-boot can be running from sdram either because of configuration
|
|
* Header or by SPL. If because of CH, then the romcode sets the
|
|
* CHSETTINGS executed bit to true in the boot parameter structure that
|
|
* it passes to the bootloader.This parameter is stored in the ch_flags
|
|
* variable by both SPL and u-boot.Check out for CHSETTINGS, which is a
|
|
* mandatory section if CH is present.
|
|
*/
|
|
if (gd->arch.omap_ch_flags & CH_FLAGS_CHSETTINGS)
|
|
return 0;
|
|
else
|
|
return running_from_sdram();
|
|
}
|
|
|
|
/*
|
|
* The basic hardware init of OMAP(s_init()) can happen in 4
|
|
* different contexts:
|
|
* 1. SPL running from SRAM
|
|
* 2. U-Boot running from FLASH
|
|
* 3. Non-XIP U-Boot loaded to SDRAM by SPL
|
|
* 4. Non-XIP U-Boot loaded to SDRAM by ROM code using the
|
|
* Configuration Header feature
|
|
*
|
|
* This function finds this context.
|
|
* Defining as inline may help in compiling out unused functions in SPL
|
|
*/
|
|
static inline u32 omap_hw_init_context(void)
|
|
{
|
|
#ifdef CONFIG_SPL_BUILD
|
|
return OMAP_INIT_CONTEXT_SPL;
|
|
#else
|
|
if (uboot_loaded_by_spl())
|
|
return OMAP_INIT_CONTEXT_UBOOT_AFTER_SPL;
|
|
else if (running_from_sdram())
|
|
return OMAP_INIT_CONTEXT_UBOOT_AFTER_CH;
|
|
else
|
|
return OMAP_INIT_CONTEXT_UBOOT_FROM_NOR;
|
|
#endif
|
|
}
|
|
#endif
|
|
|
|
#endif
|