mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
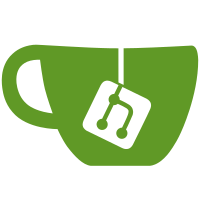
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
91 lines
2.7 KiB
C
91 lines
2.7 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Copyright (c) 2012, NVIDIA CORPORATION. All rights reserved.
|
|
*
|
|
* Inspired by cmd_ext_common.c, cmd_fat.c.
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <command.h>
|
|
#include <fs.h>
|
|
#include <efi_loader.h>
|
|
|
|
static int do_size_wrapper(cmd_tbl_t *cmdtp, int flag, int argc, char * const argv[])
|
|
{
|
|
return do_size(cmdtp, flag, argc, argv, FS_TYPE_ANY);
|
|
}
|
|
|
|
U_BOOT_CMD(
|
|
size, 4, 0, do_size_wrapper,
|
|
"determine a file's size",
|
|
"<interface> <dev[:part]> <filename>\n"
|
|
" - Find file 'filename' from 'dev' on 'interface'\n"
|
|
" and determine its size."
|
|
);
|
|
|
|
static int do_load_wrapper(cmd_tbl_t *cmdtp, int flag, int argc,
|
|
char * const argv[])
|
|
{
|
|
efi_set_bootdev(argv[1], (argc > 2) ? argv[2] : "",
|
|
(argc > 4) ? argv[4] : "");
|
|
return do_load(cmdtp, flag, argc, argv, FS_TYPE_ANY);
|
|
}
|
|
|
|
U_BOOT_CMD(
|
|
load, 7, 0, do_load_wrapper,
|
|
"load binary file from a filesystem",
|
|
"<interface> [<dev[:part]> [<addr> [<filename> [bytes [pos]]]]]\n"
|
|
" - Load binary file 'filename' from partition 'part' on device\n"
|
|
" type 'interface' instance 'dev' to address 'addr' in memory.\n"
|
|
" 'bytes' gives the size to load in bytes.\n"
|
|
" If 'bytes' is 0 or omitted, the file is read until the end.\n"
|
|
" 'pos' gives the file byte position to start reading from.\n"
|
|
" If 'pos' is 0 or omitted, the file is read from the start."
|
|
)
|
|
|
|
static int do_save_wrapper(cmd_tbl_t *cmdtp, int flag, int argc,
|
|
char * const argv[])
|
|
{
|
|
return do_save(cmdtp, flag, argc, argv, FS_TYPE_ANY);
|
|
}
|
|
|
|
U_BOOT_CMD(
|
|
save, 7, 0, do_save_wrapper,
|
|
"save file to a filesystem",
|
|
"<interface> <dev[:part]> <addr> <filename> bytes [pos]\n"
|
|
" - Save binary file 'filename' to partition 'part' on device\n"
|
|
" type 'interface' instance 'dev' from addr 'addr' in memory.\n"
|
|
" 'bytes' gives the size to save in bytes and is mandatory.\n"
|
|
" 'pos' gives the file byte position to start writing to.\n"
|
|
" If 'pos' is 0 or omitted, the file is written from the start."
|
|
)
|
|
|
|
static int do_ls_wrapper(cmd_tbl_t *cmdtp, int flag, int argc,
|
|
char * const argv[])
|
|
{
|
|
return do_ls(cmdtp, flag, argc, argv, FS_TYPE_ANY);
|
|
}
|
|
|
|
U_BOOT_CMD(
|
|
ls, 4, 1, do_ls_wrapper,
|
|
"list files in a directory (default /)",
|
|
"<interface> [<dev[:part]> [directory]]\n"
|
|
" - List files in directory 'directory' of partition 'part' on\n"
|
|
" device type 'interface' instance 'dev'."
|
|
)
|
|
|
|
static int do_fstype_wrapper(cmd_tbl_t *cmdtp, int flag, int argc,
|
|
char * const argv[])
|
|
{
|
|
return do_fs_type(cmdtp, flag, argc, argv);
|
|
}
|
|
|
|
U_BOOT_CMD(
|
|
fstype, 4, 1, do_fstype_wrapper,
|
|
"Look up a filesystem type",
|
|
"<interface> <dev>:<part>\n"
|
|
"- print filesystem type\n"
|
|
"fstype <interface> <dev>:<part> <varname>\n"
|
|
"- set environment variable to filesystem type\n"
|
|
);
|