mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
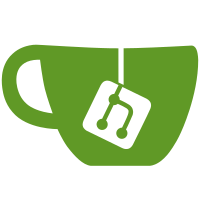
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
109 lines
2.7 KiB
C
109 lines
2.7 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* (C) Copyright 2000-2009
|
|
* Wolfgang Denk, DENX Software Engineering, wd@denx.de.
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <bootm.h>
|
|
#include <command.h>
|
|
#include <lmb.h>
|
|
#include <linux/compiler.h>
|
|
|
|
int __weak bootz_setup(ulong image, ulong *start, ulong *end)
|
|
{
|
|
/* Please define bootz_setup() for your platform */
|
|
|
|
puts("Your platform's zImage format isn't supported yet!\n");
|
|
return -1;
|
|
}
|
|
|
|
/*
|
|
* zImage booting support
|
|
*/
|
|
static int bootz_start(cmd_tbl_t *cmdtp, int flag, int argc,
|
|
char * const argv[], bootm_headers_t *images)
|
|
{
|
|
int ret;
|
|
ulong zi_start, zi_end;
|
|
|
|
ret = do_bootm_states(cmdtp, flag, argc, argv, BOOTM_STATE_START,
|
|
images, 1);
|
|
|
|
/* Setup Linux kernel zImage entry point */
|
|
if (!argc) {
|
|
images->ep = load_addr;
|
|
debug("* kernel: default image load address = 0x%08lx\n",
|
|
load_addr);
|
|
} else {
|
|
images->ep = simple_strtoul(argv[0], NULL, 16);
|
|
debug("* kernel: cmdline image address = 0x%08lx\n",
|
|
images->ep);
|
|
}
|
|
|
|
ret = bootz_setup(images->ep, &zi_start, &zi_end);
|
|
if (ret != 0)
|
|
return 1;
|
|
|
|
lmb_reserve(&images->lmb, images->ep, zi_end - zi_start);
|
|
|
|
/*
|
|
* Handle the BOOTM_STATE_FINDOTHER state ourselves as we do not
|
|
* have a header that provide this informaiton.
|
|
*/
|
|
if (bootm_find_images(flag, argc, argv))
|
|
return 1;
|
|
|
|
return 0;
|
|
}
|
|
|
|
int do_bootz(cmd_tbl_t *cmdtp, int flag, int argc, char * const argv[])
|
|
{
|
|
int ret;
|
|
|
|
/* Consume 'bootz' */
|
|
argc--; argv++;
|
|
|
|
if (bootz_start(cmdtp, flag, argc, argv, &images))
|
|
return 1;
|
|
|
|
/*
|
|
* We are doing the BOOTM_STATE_LOADOS state ourselves, so must
|
|
* disable interrupts ourselves
|
|
*/
|
|
bootm_disable_interrupts();
|
|
|
|
images.os.os = IH_OS_LINUX;
|
|
ret = do_bootm_states(cmdtp, flag, argc, argv,
|
|
#ifdef CONFIG_SYS_BOOT_RAMDISK_HIGH
|
|
BOOTM_STATE_RAMDISK |
|
|
#endif
|
|
BOOTM_STATE_OS_PREP | BOOTM_STATE_OS_FAKE_GO |
|
|
BOOTM_STATE_OS_GO,
|
|
&images, 1);
|
|
|
|
return ret;
|
|
}
|
|
|
|
#ifdef CONFIG_SYS_LONGHELP
|
|
static char bootz_help_text[] =
|
|
"[addr [initrd[:size]] [fdt]]\n"
|
|
" - boot Linux zImage stored in memory\n"
|
|
"\tThe argument 'initrd' is optional and specifies the address\n"
|
|
"\tof the initrd in memory. The optional argument ':size' allows\n"
|
|
"\tspecifying the size of RAW initrd.\n"
|
|
#if defined(CONFIG_OF_LIBFDT)
|
|
"\tWhen booting a Linux kernel which requires a flat device-tree\n"
|
|
"\ta third argument is required which is the address of the\n"
|
|
"\tdevice-tree blob. To boot that kernel without an initrd image,\n"
|
|
"\tuse a '-' for the second argument. If you do not pass a third\n"
|
|
"\ta bd_info struct will be passed instead\n"
|
|
#endif
|
|
"";
|
|
#endif
|
|
|
|
U_BOOT_CMD(
|
|
bootz, CONFIG_SYS_MAXARGS, 1, do_bootz,
|
|
"boot Linux zImage image from memory", bootz_help_text
|
|
);
|