mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
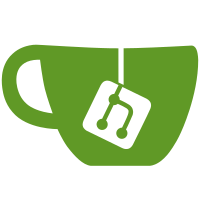
Rewrite interrupt handling functionality for the i386 port. Separated functionality into separate CPU and Architecture components. It appears as if the i386 interrupt handler functionality was intended to allow multiple handlers to be installed for a given interrupt. Unfortunately, this functionality was not fully implemented and also had the problem that irq_free_handler() does not allow the passing of the handler function pointer and therefore could never be used to free specific handlers that had been installed for a given IRQ. There were also various issues with array bounds not being fully tested. I had two objectives in mind for the new implementation: 1) Keep the implementation as similar as possible to existing implementations. To that end, I have used the leon2/3 implementations as the reference 2) Seperate CPU and Architecture specific elements. All specific i386 interrupt functionality is now in cpu/i386/ with the high level API and architecture specific code in lib_i386. Functionality specific to the PC/AT architecture (i.e. cascaded i8259 PICs) has been further split out into an individual file to allow for the implementation of the PIC architecture of the SC520 CPU (supports more IRQs) Signed-off-by: Graeme Russ <graeme.russ at gmail.com>
74 lines
2.0 KiB
C
74 lines
2.0 KiB
C
/*
|
|
* (C) Copyright 2009
|
|
* Graeme Russ, graeme.russ@gmail.com
|
|
*
|
|
* (C) Copyright 2002
|
|
* Daniel Engström, Omicron Ceti AB, daniel@omicron.se
|
|
*
|
|
* See file CREDITS for list of people who contributed to this
|
|
* project.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston,
|
|
* MA 02111-1307 USA
|
|
*/
|
|
|
|
#ifndef __ASM_INTERRUPT_H_
|
|
#define __ASM_INTERRUPT_H_ 1
|
|
|
|
/* cpu/i386/interrupts.c */
|
|
void set_vector(u8 intnum, void *routine);
|
|
|
|
/* lib_i386/interupts.c */
|
|
void disable_irq(int irq);
|
|
void enable_irq(int irq);
|
|
|
|
/* Architecture specific functions */
|
|
void mask_irq(int irq);
|
|
void unmask_irq(int irq);
|
|
void specific_eoi(int irq);
|
|
|
|
extern char exception_stack[];
|
|
|
|
#define __isr__ void __attribute__ ((regparm(0)))
|
|
|
|
#define DECLARE_INTERRUPT(x) \
|
|
asm(".globl irq_"#x"\n" \
|
|
"irq_"#x":\n" \
|
|
"pusha \n" \
|
|
"pushl $"#x"\n" \
|
|
"pushl $irq_return\n" \
|
|
"jmp do_irq\n"); \
|
|
__isr__ irq_##x(void)
|
|
|
|
#define DECLARE_EXCEPTION(x, f) \
|
|
asm(".globl exp_"#x"\n" \
|
|
"exp_"#x":\n" \
|
|
"pusha \n" \
|
|
"movl %esp, %ebx\n" \
|
|
"movl $exception_stack, %eax\n" \
|
|
"movl %eax, %esp \n" \
|
|
"pushl %ebx\n" \
|
|
"movl 32(%esp), %ebx\n" \
|
|
"xorl %edx, %edx\n" \
|
|
"movw 36(%esp), %dx\n" \
|
|
"pushl %edx\n" \
|
|
"pushl %ebx\n" \
|
|
"pushl $"#x"\n" \
|
|
"pushl $exp_return\n" \
|
|
"jmp "#f"\n"); \
|
|
__isr__ exp_##x(void)
|
|
|
|
#endif
|