mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-08-01 07:43:45 +09:00
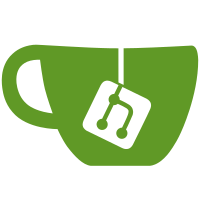
CONFIG_ENV_VARS_UBOOT_CONFIG, CONFIG_ENV_VARS_UBOOT_RUNTIME_CONFIG and CONFIG_BOARD_LATE_INIT is already set. Adding support to detect the board. These variables are used by findfdt. Signed-off-by: Sekhar Nori <nsekhar@ti.com> Signed-off-by: Lokesh Vutla <lokeshvutla@ti.com>
120 lines
2.5 KiB
C
120 lines
2.5 KiB
C
/*
|
|
* board.c
|
|
*
|
|
* Board functions for TI AM43XX based boards
|
|
*
|
|
* Copyright (C) 2013, Texas Instruments, Incorporated - http://www.ti.com/
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <i2c.h>
|
|
#include <asm/errno.h>
|
|
#include <spl.h>
|
|
#include <asm/arch/clock.h>
|
|
#include <asm/arch/sys_proto.h>
|
|
#include <asm/arch/mux.h>
|
|
#include "board.h"
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
/*
|
|
* Read header information from EEPROM into global structure.
|
|
*/
|
|
static int read_eeprom(struct am43xx_board_id *header)
|
|
{
|
|
/* Check if baseboard eeprom is available */
|
|
if (i2c_probe(CONFIG_SYS_I2C_EEPROM_ADDR)) {
|
|
printf("Could not probe the EEPROM at 0x%x\n",
|
|
CONFIG_SYS_I2C_EEPROM_ADDR);
|
|
return -ENODEV;
|
|
}
|
|
|
|
/* read the eeprom using i2c */
|
|
if (i2c_read(CONFIG_SYS_I2C_EEPROM_ADDR, 0, 2, (uchar *)header,
|
|
sizeof(struct am43xx_board_id))) {
|
|
printf("Could not read the EEPROM\n");
|
|
return -EIO;
|
|
}
|
|
|
|
if (header->magic != 0xEE3355AA) {
|
|
/*
|
|
* read the eeprom using i2c again,
|
|
* but use only a 1 byte address
|
|
*/
|
|
if (i2c_read(CONFIG_SYS_I2C_EEPROM_ADDR, 0, 1, (uchar *)header,
|
|
sizeof(struct am43xx_board_id))) {
|
|
printf("Could not read the EEPROM at 0x%x\n",
|
|
CONFIG_SYS_I2C_EEPROM_ADDR);
|
|
return -EIO;
|
|
}
|
|
|
|
if (header->magic != 0xEE3355AA) {
|
|
printf("Incorrect magic number (0x%x) in EEPROM\n",
|
|
header->magic);
|
|
return -EINVAL;
|
|
}
|
|
}
|
|
|
|
strncpy(am43xx_board_name, (char *)header->name, sizeof(header->name));
|
|
am43xx_board_name[sizeof(header->name)] = 0;
|
|
|
|
return 0;
|
|
}
|
|
|
|
#ifdef CONFIG_SPL_BUILD
|
|
|
|
const struct dpll_params dpll_ddr = {
|
|
-1, -1, -1, -1, -1, -1, -1};
|
|
|
|
const struct dpll_params *get_dpll_ddr_params(void)
|
|
{
|
|
return &dpll_ddr;
|
|
}
|
|
|
|
void set_uart_mux_conf(void)
|
|
{
|
|
enable_uart0_pin_mux();
|
|
}
|
|
|
|
void set_mux_conf_regs(void)
|
|
{
|
|
enable_board_pin_mux();
|
|
}
|
|
|
|
void sdram_init(void)
|
|
{
|
|
}
|
|
#endif
|
|
|
|
int board_init(void)
|
|
{
|
|
gd->bd->bi_boot_params = CONFIG_SYS_SDRAM_BASE + 0x100;
|
|
|
|
return 0;
|
|
}
|
|
|
|
#ifdef CONFIG_BOARD_LATE_INIT
|
|
int board_late_init(void)
|
|
{
|
|
#ifdef CONFIG_ENV_VARS_UBOOT_RUNTIME_CONFIG
|
|
char safe_string[HDR_NAME_LEN + 1];
|
|
struct am43xx_board_id header;
|
|
|
|
if (read_eeprom(&header) < 0)
|
|
puts("Could not get board ID.\n");
|
|
|
|
/* Now set variables based on the header. */
|
|
strncpy(safe_string, (char *)header.name, sizeof(header.name));
|
|
safe_string[sizeof(header.name)] = 0;
|
|
setenv("board_name", safe_string);
|
|
|
|
strncpy(safe_string, (char *)header.version, sizeof(header.version));
|
|
safe_string[sizeof(header.version)] = 0;
|
|
setenv("board_rev", safe_string);
|
|
#endif
|
|
return 0;
|
|
}
|
|
#endif
|