mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-07 19:56:18 +09:00
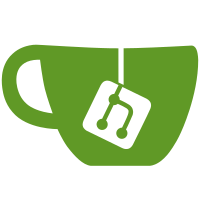
At present the FDTs are keyed by their default filename (not their actual filename). It seems easier to key by the entry type, since this is always the same for each FDT type. To do this, add a new Entry method called GetFdtEtype(). This is necessary since some entry types contain a device tree which are not the simple three entry types 'u-boot-dtb', 'u-boot-spl' or 'u-boot-tpl'. The code already returns a dict for GetFdt(). Update the value of that dict to include the filename so that existing code can work. Signed-off-by: Simon Glass <sjg@chromium.org>
98 lines
3.2 KiB
Python
98 lines
3.2 KiB
Python
# SPDX-License-Identifier: GPL-2.0+
|
|
# Copyright (c) 2016 Google, Inc
|
|
# Written by Simon Glass <sjg@chromium.org>
|
|
#
|
|
# Test for the Entry class
|
|
|
|
import collections
|
|
import os
|
|
import sys
|
|
import unittest
|
|
|
|
import entry
|
|
import fdt
|
|
import fdt_util
|
|
import tools
|
|
|
|
class TestEntry(unittest.TestCase):
|
|
def setUp(self):
|
|
tools.PrepareOutputDir(None)
|
|
|
|
def tearDown(self):
|
|
tools.FinaliseOutputDir()
|
|
|
|
def GetNode(self):
|
|
binman_dir = os.path.dirname(os.path.realpath(sys.argv[0]))
|
|
fname = fdt_util.EnsureCompiled(
|
|
os.path.join(binman_dir,('test/005_simple.dts')))
|
|
dtb = fdt.FdtScan(fname)
|
|
return dtb.GetNode('/binman/u-boot')
|
|
|
|
def _ReloadEntry(self):
|
|
global entry
|
|
if entry:
|
|
if sys.version_info[0] >= 3:
|
|
import importlib
|
|
importlib.reload(entry)
|
|
else:
|
|
reload(entry)
|
|
else:
|
|
import entry
|
|
|
|
def test1EntryNoImportLib(self):
|
|
"""Test that we can import Entry subclassess successfully"""
|
|
sys.modules['importlib'] = None
|
|
global entry
|
|
self._ReloadEntry()
|
|
entry.Entry.Create(None, self.GetNode(), 'u-boot')
|
|
self.assertFalse(entry.have_importlib)
|
|
|
|
def test2EntryImportLib(self):
|
|
del sys.modules['importlib']
|
|
global entry
|
|
self._ReloadEntry()
|
|
entry.Entry.Create(None, self.GetNode(), 'u-boot-spl')
|
|
self.assertTrue(entry.have_importlib)
|
|
|
|
def testEntryContents(self):
|
|
"""Test the Entry bass class"""
|
|
import entry
|
|
base_entry = entry.Entry(None, None, None, read_node=False)
|
|
self.assertEqual(True, base_entry.ObtainContents())
|
|
|
|
def testUnknownEntry(self):
|
|
"""Test that unknown entry types are detected"""
|
|
Node = collections.namedtuple('Node', ['name', 'path'])
|
|
node = Node('invalid-name', 'invalid-path')
|
|
with self.assertRaises(ValueError) as e:
|
|
entry.Entry.Create(None, node, node.name)
|
|
self.assertIn("Unknown entry type 'invalid-name' in node "
|
|
"'invalid-path'", str(e.exception))
|
|
|
|
def testUniqueName(self):
|
|
"""Test Entry.GetUniqueName"""
|
|
Node = collections.namedtuple('Node', ['name', 'parent'])
|
|
base_node = Node('root', None)
|
|
base_entry = entry.Entry(None, None, base_node, read_node=False)
|
|
self.assertEqual('root', base_entry.GetUniqueName())
|
|
sub_node = Node('subnode', base_node)
|
|
sub_entry = entry.Entry(None, None, sub_node, read_node=False)
|
|
self.assertEqual('root.subnode', sub_entry.GetUniqueName())
|
|
|
|
def testGetDefaultFilename(self):
|
|
"""Trivial test for this base class function"""
|
|
base_entry = entry.Entry(None, None, None, read_node=False)
|
|
self.assertIsNone(base_entry.GetDefaultFilename())
|
|
|
|
def testBlobFdt(self):
|
|
"""Test the GetFdtEtype() method of the blob-dtb entries"""
|
|
base = entry.Entry.Create(None, self.GetNode(), 'blob-dtb')
|
|
self.assertIsNone(base.GetFdtEtype())
|
|
|
|
dtb = entry.Entry.Create(None, self.GetNode(), 'u-boot-dtb')
|
|
self.assertEqual('u-boot-dtb', dtb.GetFdtEtype())
|
|
|
|
|
|
if __name__ == "__main__":
|
|
unittest.main()
|