mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-09 04:36:16 +09:00
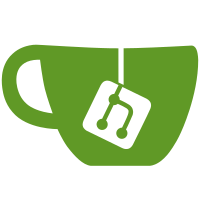
Convert cache flush to use dm cpu data. The original cache flush functions are written in assembly and use CONFIG_SYS_{I,D}CACHE_SIZE... macros. It is difficult to convert to use cache configuration in dm cpu data which is extracted from device tree. The cacheflush.c of Linux nios2 arch uses cpuinfo structure, which is very close to our dm cpu data. So we copy and modify it to arch/nios2/lib/cache.c to replace the old cache.S. Signed-off-by: Thomas Chou <thomas@wytron.com.tw>
58 lines
1.7 KiB
C
58 lines
1.7 KiB
C
/*
|
|
* (C) Copyright 2003, Psyent Corporation <www.psyent.com>
|
|
* Scott McNutt <smcnutt@psyent.com>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
|
|
#define NIOS_MAGIC 0x534f494e /* enable command line and initrd passing */
|
|
|
|
int do_bootm_linux(int flag, int argc, char * const argv[], bootm_headers_t *images)
|
|
{
|
|
void (*kernel)(int, int, int, char *) = (void *)images->ep;
|
|
char *commandline = getenv("bootargs");
|
|
ulong initrd_start = images->rd_start;
|
|
ulong initrd_end = images->rd_end;
|
|
char *of_flat_tree = NULL;
|
|
#if defined(CONFIG_OF_LIBFDT)
|
|
/* did generic code already find a device tree? */
|
|
if (images->ft_len)
|
|
of_flat_tree = images->ft_addr;
|
|
#endif
|
|
if (!of_flat_tree && argc > 1)
|
|
of_flat_tree = (char *)simple_strtoul(argv[1], NULL, 16);
|
|
if (of_flat_tree)
|
|
initrd_end = (ulong)of_flat_tree;
|
|
|
|
/*
|
|
* allow the PREP bootm subcommand, it is required for bootm to work
|
|
*/
|
|
if (flag & BOOTM_STATE_OS_PREP)
|
|
return 0;
|
|
|
|
if ((flag != 0) && (flag != BOOTM_STATE_OS_GO))
|
|
return 1;
|
|
|
|
/* flushes data and instruction caches before calling the kernel */
|
|
disable_interrupts();
|
|
flush_dcache_all();
|
|
|
|
debug("bootargs=%s @ 0x%lx\n", commandline, (ulong)&commandline);
|
|
debug("initrd=0x%lx-0x%lx\n", (ulong)initrd_start, (ulong)initrd_end);
|
|
/* kernel parameters passing
|
|
* r4 : NIOS magic
|
|
* r5 : initrd start
|
|
* r6 : initrd end or fdt
|
|
* r7 : kernel command line
|
|
* fdt is passed to kernel via r6, the same as initrd_end. fdt will be
|
|
* verified with fdt magic. when both initrd and fdt are used at the
|
|
* same time, fdt must follow immediately after initrd.
|
|
*/
|
|
kernel(NIOS_MAGIC, initrd_start, initrd_end, commandline);
|
|
/* does not return */
|
|
|
|
return 1;
|
|
}
|