mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-08-02 16:23:46 +09:00
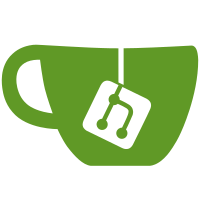
The get_sw_write_prot API is used to get the write-protected bits of flash by reading the status register and other wards it's API for reading register bits. 1) This kind of requirement can be achieved using existing flash operations and flash locking API calls instead of making a separate flash API. 2) Technically there is no real hardware user for this API to use in the source tree. 3) Having a flash operations API for simple register read bits also make difficult to extend the flash operations. 4) Instead of touching generic code, it is possible to have this functionality inside spinor operations in the form of flash hooks or fixups for associated flash chips. Considering all these points, this patch drops the get_sw_write_prot and associated code bases. Cc: Simon Glass <sjg@chromium.org> Cc: Vignesh R <vigneshr@ti.com> Signed-off-by: Jagan Teki <jagan@amarulasolutions.com>
93 lines
2.6 KiB
C
93 lines
2.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* SPI flash internal definitions
|
|
*
|
|
* Copyright (C) 2008 Atmel Corporation
|
|
* Copyright (C) 2013 Jagannadha Sutradharudu Teki, Xilinx Inc.
|
|
*/
|
|
|
|
#ifndef _SF_INTERNAL_H_
|
|
#define _SF_INTERNAL_H_
|
|
|
|
#include <linux/bitops.h>
|
|
#include <linux/types.h>
|
|
#include <linux/compiler.h>
|
|
|
|
#define SPI_NOR_MAX_ID_LEN 6
|
|
#define SPI_NOR_MAX_ADDR_WIDTH 4
|
|
|
|
struct flash_info {
|
|
#if !CONFIG_IS_ENABLED(SPI_FLASH_TINY)
|
|
char *name;
|
|
#endif
|
|
|
|
/*
|
|
* This array stores the ID bytes.
|
|
* The first three bytes are the JEDIC ID.
|
|
* JEDEC ID zero means "no ID" (mostly older chips).
|
|
*/
|
|
u8 id[SPI_NOR_MAX_ID_LEN];
|
|
u8 id_len;
|
|
|
|
/* The size listed here is what works with SPINOR_OP_SE, which isn't
|
|
* necessarily called a "sector" by the vendor.
|
|
*/
|
|
unsigned int sector_size;
|
|
u16 n_sectors;
|
|
|
|
u16 page_size;
|
|
u16 addr_width;
|
|
|
|
u32 flags;
|
|
#define SECT_4K BIT(0) /* SPINOR_OP_BE_4K works uniformly */
|
|
#define SPI_NOR_NO_ERASE BIT(1) /* No erase command needed */
|
|
#define SST_WRITE BIT(2) /* use SST byte programming */
|
|
#define SPI_NOR_NO_FR BIT(3) /* Can't do fastread */
|
|
#define SECT_4K_PMC BIT(4) /* SPINOR_OP_BE_4K_PMC works uniformly */
|
|
#define SPI_NOR_DUAL_READ BIT(5) /* Flash supports Dual Read */
|
|
#define SPI_NOR_QUAD_READ BIT(6) /* Flash supports Quad Read */
|
|
#define USE_FSR BIT(7) /* use flag status register */
|
|
#define SPI_NOR_HAS_LOCK BIT(8) /* Flash supports lock/unlock via SR */
|
|
#define SPI_NOR_HAS_TB BIT(9) /*
|
|
* Flash SR has Top/Bottom (TB) protect
|
|
* bit. Must be used with
|
|
* SPI_NOR_HAS_LOCK.
|
|
*/
|
|
#define SPI_S3AN BIT(10) /*
|
|
* Xilinx Spartan 3AN In-System Flash
|
|
* (MFR cannot be used for probing
|
|
* because it has the same value as
|
|
* ATMEL flashes)
|
|
*/
|
|
#define SPI_NOR_4B_OPCODES BIT(11) /*
|
|
* Use dedicated 4byte address op codes
|
|
* to support memory size above 128Mib.
|
|
*/
|
|
#define NO_CHIP_ERASE BIT(12) /* Chip does not support chip erase */
|
|
#define SPI_NOR_SKIP_SFDP BIT(13) /* Skip parsing of SFDP tables */
|
|
#define USE_CLSR BIT(14) /* use CLSR command */
|
|
#define SPI_NOR_HAS_SST26LOCK BIT(15) /* Flash supports lock/unlock via BPR */
|
|
#define SPI_NOR_OCTAL_READ BIT(16) /* Flash supports Octal Read */
|
|
};
|
|
|
|
extern const struct flash_info spi_nor_ids[];
|
|
|
|
#define JEDEC_MFR(info) ((info)->id[0])
|
|
#define JEDEC_ID(info) (((info)->id[1]) << 8 | ((info)->id[2]))
|
|
|
|
#if CONFIG_IS_ENABLED(SPI_FLASH_MTD)
|
|
int spi_flash_mtd_register(struct spi_flash *flash);
|
|
void spi_flash_mtd_unregister(void);
|
|
#else
|
|
static inline int spi_flash_mtd_register(struct spi_flash *flash)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
static inline void spi_flash_mtd_unregister(void)
|
|
{
|
|
}
|
|
#endif
|
|
|
|
#endif /* _SF_INTERNAL_H_ */
|