mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-08-10 20:23:54 +09:00
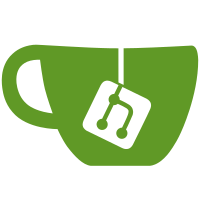
Add the CRC32 algorithm to the list of available hashes, and make the crc32 command use hash_command(). Add a new crc32_wd_buf() to make this possible, which puts its result in a buffer rather than returning it as a 32-bit value. Note: For some boards the hash command is not enabled, neither are sha1, sha256 or the verify option. In this case the full hash implementation adds about 500 bytes of overhead. So as a special case, we use #ifdef to select very simple bahaviour in that case. The justification for this is that it is currently a very common case (virtually all boards enable crc32 but only some enable more advanced features). Signed-off-by: Simon Glass <sjg@chromium.org>
75 lines
2.4 KiB
C
75 lines
2.4 KiB
C
/*
|
|
* Copyright (c) 2012 The Chromium OS Authors.
|
|
* See file CREDITS for list of people who contributed to this
|
|
* project.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston,
|
|
* MA 02111-1307 USA
|
|
*/
|
|
|
|
#ifndef _HASH_H
|
|
#define _HASH_H
|
|
|
|
#if defined(CONFIG_SHA1SUM_VERIFY) || defined(CONFIG_CRC32_VERIFY)
|
|
#define CONFIG_HASH_VERIFY
|
|
#endif
|
|
|
|
struct hash_algo {
|
|
const char *name; /* Name of algorithm */
|
|
int digest_size; /* Length of digest */
|
|
/**
|
|
* hash_func_ws: Generic hashing function
|
|
*
|
|
* This is the generic prototype for a hashing function. We only
|
|
* have the watchdog version at present.
|
|
*
|
|
* @input: Input buffer
|
|
* @ilen: Input buffer length
|
|
* @output: Checksum result (length depends on algorithm)
|
|
* @chunk_sz: Trigger watchdog after processing this many bytes
|
|
*/
|
|
void (*hash_func_ws)(const unsigned char *input, unsigned int ilen,
|
|
unsigned char *output, unsigned int chunk_sz);
|
|
int chunk_size; /* Watchdog chunk size */
|
|
};
|
|
|
|
/*
|
|
* Maximum digest size for all algorithms we support. Having this value
|
|
* avoids a malloc() or C99 local declaration in common/cmd_hash.c.
|
|
*/
|
|
#define HASH_MAX_DIGEST_SIZE 32
|
|
|
|
enum {
|
|
HASH_FLAG_VERIFY = 1 << 0, /* Enable verify mode */
|
|
HASH_FLAG_ENV = 1 << 1, /* Allow env vars */
|
|
};
|
|
|
|
/**
|
|
* hash_command: Process a hash command for a particular algorithm
|
|
*
|
|
* This common function is used to implement specific hash commands.
|
|
*
|
|
* @algo_name: Hash algorithm being used
|
|
* @flags: Flags value (HASH_FLAG_...)
|
|
* @cmdtp: Pointer to command table entry
|
|
* @flag: Some flags normally 0 (see CMD_FLAG_.. above)
|
|
* @argc: Number of arguments (arg 0 must be the command text)
|
|
* @argv: Arguments
|
|
*/
|
|
int hash_command(const char *algo_name, int flags, cmd_tbl_t *cmdtp, int flag,
|
|
int argc, char * const argv[]);
|
|
|
|
#endif
|