mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-12 14:16:17 +09:00
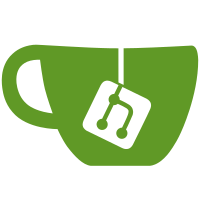
Commit 3ff46cc4
fixed exception vectors setting in
the general ARM case, by either copying the exception
and indirect vector tables to normal (0x00000000) or
high (0xFFFF0000) vectors address, or setting VBAR to
U-Boot's base if applicable.
i.MX27 SoC is ARM926E-JS, thus has only normal and
high options, but does not provide RAM at 0xFFFF0000
and has only ROM at 0x00000000; it is therefore not
possible to move or change its exception vectors.
Besides, i.MX27 ROM code does provide an indirect
vectors table but at a non-standard address and with
the reset and reserved vectors missing.
Turn the current vector relocation code into a weak
routine called after relocate_code from crt0, and add
strong version for i.MX27.
Series-Cc: Heiko Schocher <hs@denx.de>
Signed-off-by: Albert ARIBAUD <albert.u.boot@aribaud.net>
Reviewed-by: Stefano Babic <sbabic@denx.de>
Tested-by: Stefano Babic <sbabic@denx.de>
Tested-by: Philippe Reynes <tremyfr@gmail.com>
Tested-by: Philippe Reynes <tremyfr@yahoo.fr>
118 lines
3.0 KiB
ArmAsm
118 lines
3.0 KiB
ArmAsm
/*
|
|
* relocate - common relocation function for ARM U-Boot
|
|
*
|
|
* Copyright (c) 2013 Albert ARIBAUD <albert.u.boot@aribaud.net>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <asm-offsets.h>
|
|
#include <config.h>
|
|
#include <linux/linkage.h>
|
|
|
|
/*
|
|
* Default/weak exception vectors relocation routine
|
|
*
|
|
* This routine covers the standard ARM cases: normal (0x00000000),
|
|
* high (0xffff0000) and VBAR. SoCs which do not comply with any of
|
|
* the standard cases must provide their own, strong, version.
|
|
*/
|
|
|
|
.section .text.relocate_vectors,"ax",%progbits
|
|
.weak relocate_vectors
|
|
|
|
ENTRY(relocate_vectors)
|
|
|
|
#ifdef CONFIG_HAS_VBAR
|
|
/*
|
|
* If the ARM processor has the security extensions,
|
|
* use VBAR to relocate the exception vectors.
|
|
*/
|
|
ldr r0, [r9, #GD_RELOCADDR] /* r0 = gd->relocaddr */
|
|
mcr p15, 0, r0, c12, c0, 0 /* Set VBAR */
|
|
#else
|
|
/*
|
|
* Copy the relocated exception vectors to the
|
|
* correct address
|
|
* CP15 c1 V bit gives us the location of the vectors:
|
|
* 0x00000000 or 0xFFFF0000.
|
|
*/
|
|
ldr r0, [r9, #GD_RELOCADDR] /* r0 = gd->relocaddr */
|
|
mrc p15, 0, r2, c1, c0, 0 /* V bit (bit[13]) in CP15 c1 */
|
|
ands r2, r2, #(1 << 13)
|
|
ldreq r1, =0x00000000 /* If V=0 */
|
|
ldrne r1, =0xFFFF0000 /* If V=1 */
|
|
ldmia r0!, {r2-r8,r10}
|
|
stmia r1!, {r2-r8,r10}
|
|
ldmia r0!, {r2-r8,r10}
|
|
stmia r1!, {r2-r8,r10}
|
|
#endif
|
|
bx lr
|
|
|
|
ENDPROC(relocate_vectors)
|
|
|
|
/*
|
|
* void relocate_code(addr_moni)
|
|
*
|
|
* This function relocates the monitor code.
|
|
*
|
|
* NOTE:
|
|
* To prevent the code below from containing references with an R_ARM_ABS32
|
|
* relocation record type, we never refer to linker-defined symbols directly.
|
|
* Instead, we declare literals which contain their relative location with
|
|
* respect to relocate_code, and at run time, add relocate_code back to them.
|
|
*/
|
|
|
|
ENTRY(relocate_code)
|
|
ldr r1, =__image_copy_start /* r1 <- SRC &__image_copy_start */
|
|
subs r4, r0, r1 /* r4 <- relocation offset */
|
|
beq relocate_done /* skip relocation */
|
|
ldr r2, =__image_copy_end /* r2 <- SRC &__image_copy_end */
|
|
|
|
copy_loop:
|
|
ldmia r1!, {r10-r11} /* copy from source address [r1] */
|
|
stmia r0!, {r10-r11} /* copy to target address [r0] */
|
|
cmp r1, r2 /* until source end address [r2] */
|
|
blo copy_loop
|
|
|
|
/*
|
|
* fix .rel.dyn relocations
|
|
*/
|
|
ldr r2, =__rel_dyn_start /* r2 <- SRC &__rel_dyn_start */
|
|
ldr r3, =__rel_dyn_end /* r3 <- SRC &__rel_dyn_end */
|
|
fixloop:
|
|
ldmia r2!, {r0-r1} /* (r0,r1) <- (SRC location,fixup) */
|
|
and r1, r1, #0xff
|
|
cmp r1, #23 /* relative fixup? */
|
|
bne fixnext
|
|
|
|
/* relative fix: increase location by offset */
|
|
add r0, r0, r4
|
|
ldr r1, [r0]
|
|
add r1, r1, r4
|
|
str r1, [r0]
|
|
fixnext:
|
|
cmp r2, r3
|
|
blo fixloop
|
|
|
|
relocate_done:
|
|
|
|
#ifdef __XSCALE__
|
|
/*
|
|
* On xscale, icache must be invalidated and write buffers drained,
|
|
* even with cache disabled - 4.2.7 of xscale core developer's manual
|
|
*/
|
|
mcr p15, 0, r0, c7, c7, 0 /* invalidate icache */
|
|
mcr p15, 0, r0, c7, c10, 4 /* drain write buffer */
|
|
#endif
|
|
|
|
/* ARMv4- don't know bx lr but the assembler fails to see that */
|
|
|
|
#ifdef __ARM_ARCH_4__
|
|
mov pc, lr
|
|
#else
|
|
bx lr
|
|
#endif
|
|
|
|
ENDPROC(relocate_code)
|