mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
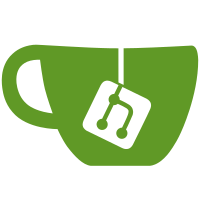
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
421 lines
13 KiB
C
421 lines
13 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* (C) Copyright 2015 Google, Inc
|
|
*
|
|
* Dhrystone is widely available in the public domain. A GPL license is
|
|
* chosen for U-Boot.
|
|
*/
|
|
|
|
/*****************************************************************************
|
|
* The BYTE UNIX Benchmarks - Release 3
|
|
* Module: dhry_1.c SID: 3.4 5/15/91 19:30:21
|
|
*
|
|
*****************************************************************************
|
|
* Bug reports, patches, comments, suggestions should be sent to:
|
|
*
|
|
* Ben Smith, Rick Grehan or Tom Yager
|
|
* ben@bytepb.byte.com rick_g@bytepb.byte.com tyager@bytepb.byte.com
|
|
*
|
|
*****************************************************************************
|
|
*
|
|
* *** WARNING **** With BYTE's modifications applied, results obtained with
|
|
* ******* this version of the Dhrystone program may not be applicable
|
|
* to other versions.
|
|
*
|
|
* Modification Log:
|
|
* 10/22/97 - code cleanup to remove ANSI C compiler warnings
|
|
* Andy Kahn <kahn@zk3.dec.com>
|
|
*
|
|
* Adapted from:
|
|
*
|
|
* "DHRYSTONE" Benchmark Program
|
|
* -----------------------------
|
|
*
|
|
* Version: C, Version 2.1
|
|
*
|
|
* File: dhry_1.c (part 2 of 3)
|
|
*
|
|
* Date: May 25, 1988
|
|
*
|
|
* Author: Reinhold P. Weicker
|
|
*
|
|
***************************************************************************/
|
|
char SCCSid[] = "@(#) @(#)dhry_1.c:3.4 -- 5/15/91 19:30:21";
|
|
|
|
#include <common.h>
|
|
#include <malloc.h>
|
|
|
|
#include "dhry.h"
|
|
|
|
unsigned long Run_Index;
|
|
|
|
void report(void)
|
|
{
|
|
printf("%ld loops\n", Run_Index);
|
|
}
|
|
|
|
/* Global Variables: */
|
|
|
|
Rec_Pointer Ptr_Glob,
|
|
Next_Ptr_Glob;
|
|
int Int_Glob;
|
|
Boolean Bool_Glob;
|
|
char Ch_1_Glob,
|
|
Ch_2_Glob;
|
|
int Arr_1_Glob [50];
|
|
int Arr_2_Glob [50] [50];
|
|
|
|
Enumeration Func_1 (Capital_Letter Ch_1_Par_Val, Capital_Letter Ch_2_Par_Val);
|
|
/* forward declaration necessary since Enumeration may not simply be int */
|
|
|
|
#ifndef REG
|
|
Boolean Reg = false;
|
|
#define REG
|
|
/* REG becomes defined as empty */
|
|
/* i.e. no register variables */
|
|
#else
|
|
Boolean Reg = true;
|
|
#endif
|
|
|
|
/* variables for time measurement: */
|
|
|
|
#ifdef TIMES
|
|
#define Too_Small_Time 120
|
|
/* Measurements should last at least about 2 seconds */
|
|
#endif
|
|
#ifdef TIME
|
|
extern long time();
|
|
/* see library function "time" */
|
|
#define Too_Small_Time 2
|
|
/* Measurements should last at least 2 seconds */
|
|
#endif
|
|
|
|
long Begin_Time,
|
|
End_Time,
|
|
User_Time;
|
|
|
|
/* end of variables for time measurement */
|
|
|
|
void Proc_1 (REG Rec_Pointer Ptr_Val_Par);
|
|
void Proc_2 (One_Fifty *Int_Par_Ref);
|
|
void Proc_3 (Rec_Pointer *Ptr_Ref_Par);
|
|
void Proc_4 (void);
|
|
void Proc_5 (void);
|
|
|
|
|
|
extern Boolean Func_2(Str_30, Str_30);
|
|
extern void Proc_6(Enumeration, Enumeration *);
|
|
extern void Proc_7(One_Fifty, One_Fifty, One_Fifty *);
|
|
extern void Proc_8(Arr_1_Dim, Arr_2_Dim, int, int);
|
|
|
|
void dhry(int Number_Of_Runs)
|
|
/* main program, corresponds to procedures */
|
|
/* Main and Proc_0 in the Ada version */
|
|
{
|
|
One_Fifty Int_1_Loc;
|
|
REG One_Fifty Int_2_Loc;
|
|
One_Fifty Int_3_Loc;
|
|
REG char Ch_Index;
|
|
Enumeration Enum_Loc;
|
|
Str_30 Str_1_Loc;
|
|
Str_30 Str_2_Loc;
|
|
|
|
/* Initializations */
|
|
|
|
Next_Ptr_Glob = (Rec_Pointer) malloc (sizeof (Rec_Type));
|
|
Ptr_Glob = (Rec_Pointer) malloc (sizeof (Rec_Type));
|
|
|
|
Ptr_Glob->Ptr_Comp = Next_Ptr_Glob;
|
|
Ptr_Glob->Discr = Ident_1;
|
|
Ptr_Glob->variant.var_1.Enum_Comp = Ident_3;
|
|
Ptr_Glob->variant.var_1.Int_Comp = 40;
|
|
strcpy (Ptr_Glob->variant.var_1.Str_Comp,
|
|
"DHRYSTONE PROGRAM, SOME STRING");
|
|
strcpy (Str_1_Loc, "DHRYSTONE PROGRAM, 1'ST STRING");
|
|
|
|
Arr_2_Glob [8][7] = 10;
|
|
/* Was missing in published program. Without this statement, */
|
|
/* Arr_2_Glob [8][7] would have an undefined value. */
|
|
/* Warning: With 16-Bit processors and Number_Of_Runs > 32000, */
|
|
/* overflow may occur for this array element. */
|
|
|
|
#ifdef PRATTLE
|
|
printf ("\n");
|
|
printf ("Dhrystone Benchmark, Version 2.1 (Language: C)\n");
|
|
printf ("\n");
|
|
if (Reg)
|
|
{
|
|
printf ("Program compiled with 'register' attribute\n");
|
|
printf ("\n");
|
|
}
|
|
else
|
|
{
|
|
printf ("Program compiled without 'register' attribute\n");
|
|
printf ("\n");
|
|
}
|
|
printf ("Please give the number of runs through the benchmark: ");
|
|
{
|
|
int n;
|
|
scanf ("%d", &n);
|
|
Number_Of_Runs = n;
|
|
}
|
|
printf ("\n");
|
|
|
|
printf ("Execution starts, %d runs through Dhrystone\n", Number_Of_Runs);
|
|
#endif /* PRATTLE */
|
|
|
|
Run_Index = 0;
|
|
|
|
/***************/
|
|
/* Start timer */
|
|
/***************/
|
|
|
|
#ifdef SELF_TIMED
|
|
#ifdef TIMES
|
|
times (&time_info);
|
|
Begin_Time = (long) time_info.tms_utime;
|
|
#endif
|
|
#ifdef TIME
|
|
Begin_Time = time ( (long *) 0);
|
|
#endif
|
|
#endif /* SELF_TIMED */
|
|
|
|
for (Run_Index = 1; Run_Index < Number_Of_Runs; ++Run_Index)
|
|
{
|
|
|
|
Proc_5();
|
|
Proc_4();
|
|
/* Ch_1_Glob == 'A', Ch_2_Glob == 'B', Bool_Glob == true */
|
|
Int_1_Loc = 2;
|
|
Int_2_Loc = 3;
|
|
strcpy (Str_2_Loc, "DHRYSTONE PROGRAM, 2'ND STRING");
|
|
Enum_Loc = Ident_2;
|
|
Bool_Glob = ! Func_2 (Str_1_Loc, Str_2_Loc);
|
|
/* Bool_Glob == 1 */
|
|
while (Int_1_Loc < Int_2_Loc) /* loop body executed once */
|
|
{
|
|
Int_3_Loc = 5 * Int_1_Loc - Int_2_Loc;
|
|
/* Int_3_Loc == 7 */
|
|
Proc_7 (Int_1_Loc, Int_2_Loc, &Int_3_Loc);
|
|
/* Int_3_Loc == 7 */
|
|
Int_1_Loc += 1;
|
|
} /* while */
|
|
/* Int_1_Loc == 3, Int_2_Loc == 3, Int_3_Loc == 7 */
|
|
Proc_8 (Arr_1_Glob, Arr_2_Glob, Int_1_Loc, Int_3_Loc);
|
|
/* Int_Glob == 5 */
|
|
Proc_1 (Ptr_Glob);
|
|
for (Ch_Index = 'A'; Ch_Index <= Ch_2_Glob; ++Ch_Index)
|
|
/* loop body executed twice */
|
|
{
|
|
if (Enum_Loc == Func_1 (Ch_Index, 'C'))
|
|
/* then, not executed */
|
|
{
|
|
Proc_6 (Ident_1, &Enum_Loc);
|
|
strcpy (Str_2_Loc, "DHRYSTONE PROGRAM, 3'RD STRING");
|
|
Int_2_Loc = Run_Index;
|
|
Int_Glob = Run_Index;
|
|
}
|
|
}
|
|
/* Int_1_Loc == 3, Int_2_Loc == 3, Int_3_Loc == 7 */
|
|
Int_2_Loc = Int_2_Loc * Int_1_Loc;
|
|
Int_1_Loc = Int_2_Loc / Int_3_Loc;
|
|
Int_2_Loc = 7 * (Int_2_Loc - Int_3_Loc) - Int_1_Loc;
|
|
/* Int_1_Loc == 1, Int_2_Loc == 13, Int_3_Loc == 7 */
|
|
Proc_2 (&Int_1_Loc);
|
|
/* Int_1_Loc == 5 */
|
|
|
|
} /* loop "for Run_Index" */
|
|
|
|
/**************/
|
|
/* Stop timer */
|
|
/**************/
|
|
#ifdef SELF_TIMED
|
|
#ifdef TIMES
|
|
times (&time_info);
|
|
End_Time = (long) time_info.tms_utime;
|
|
#endif
|
|
#ifdef TIME
|
|
End_Time = time ( (long *) 0);
|
|
#endif
|
|
#endif /* SELF_TIMED */
|
|
|
|
/* BYTE version never executes this stuff */
|
|
#ifdef SELF_TIMED
|
|
printf ("Execution ends\n");
|
|
printf ("\n");
|
|
printf ("Final values of the variables used in the benchmark:\n");
|
|
printf ("\n");
|
|
printf ("Int_Glob: %d\n", Int_Glob);
|
|
printf (" should be: %d\n", 5);
|
|
printf ("Bool_Glob: %d\n", Bool_Glob);
|
|
printf (" should be: %d\n", 1);
|
|
printf ("Ch_1_Glob: %c\n", Ch_1_Glob);
|
|
printf (" should be: %c\n", 'A');
|
|
printf ("Ch_2_Glob: %c\n", Ch_2_Glob);
|
|
printf (" should be: %c\n", 'B');
|
|
printf ("Arr_1_Glob[8]: %d\n", Arr_1_Glob[8]);
|
|
printf (" should be: %d\n", 7);
|
|
printf ("Arr_2_Glob[8][7]: %d\n", Arr_2_Glob[8][7]);
|
|
printf (" should be: Number_Of_Runs + 10\n");
|
|
printf ("Ptr_Glob->\n");
|
|
printf (" Ptr_Comp: %d\n", (int) Ptr_Glob->Ptr_Comp);
|
|
printf (" should be: (implementation-dependent)\n");
|
|
printf (" Discr: %d\n", Ptr_Glob->Discr);
|
|
printf (" should be: %d\n", 0);
|
|
printf (" Enum_Comp: %d\n", Ptr_Glob->variant.var_1.Enum_Comp);
|
|
printf (" should be: %d\n", 2);
|
|
printf (" Int_Comp: %d\n", Ptr_Glob->variant.var_1.Int_Comp);
|
|
printf (" should be: %d\n", 17);
|
|
printf (" Str_Comp: %s\n", Ptr_Glob->variant.var_1.Str_Comp);
|
|
printf (" should be: DHRYSTONE PROGRAM, SOME STRING\n");
|
|
printf ("Next_Ptr_Glob->\n");
|
|
printf (" Ptr_Comp: %d\n", (int) Next_Ptr_Glob->Ptr_Comp);
|
|
printf (" should be: (implementation-dependent), same as above\n");
|
|
printf (" Discr: %d\n", Next_Ptr_Glob->Discr);
|
|
printf (" should be: %d\n", 0);
|
|
printf (" Enum_Comp: %d\n", Next_Ptr_Glob->variant.var_1.Enum_Comp);
|
|
printf (" should be: %d\n", 1);
|
|
printf (" Int_Comp: %d\n", Next_Ptr_Glob->variant.var_1.Int_Comp);
|
|
printf (" should be: %d\n", 18);
|
|
printf (" Str_Comp: %s\n",
|
|
Next_Ptr_Glob->variant.var_1.Str_Comp);
|
|
printf (" should be: DHRYSTONE PROGRAM, SOME STRING\n");
|
|
printf ("Int_1_Loc: %d\n", Int_1_Loc);
|
|
printf (" should be: %d\n", 5);
|
|
printf ("Int_2_Loc: %d\n", Int_2_Loc);
|
|
printf (" should be: %d\n", 13);
|
|
printf ("Int_3_Loc: %d\n", Int_3_Loc);
|
|
printf (" should be: %d\n", 7);
|
|
printf ("Enum_Loc: %d\n", Enum_Loc);
|
|
printf (" should be: %d\n", 1);
|
|
printf ("Str_1_Loc: %s\n", Str_1_Loc);
|
|
printf (" should be: DHRYSTONE PROGRAM, 1'ST STRING\n");
|
|
printf ("Str_2_Loc: %s\n", Str_2_Loc);
|
|
printf (" should be: DHRYSTONE PROGRAM, 2'ND STRING\n");
|
|
printf ("\n");
|
|
|
|
User_Time = End_Time - Begin_Time;
|
|
|
|
if (User_Time < Too_Small_Time)
|
|
{
|
|
printf ("Measured time too small to obtain meaningful results\n");
|
|
printf ("Please increase number of runs\n");
|
|
printf ("\n");
|
|
}
|
|
else
|
|
{
|
|
#ifdef TIME
|
|
Microseconds = (float) User_Time * Mic_secs_Per_Second
|
|
/ (float) Number_Of_Runs;
|
|
Dhrystones_Per_Second = (float) Number_Of_Runs / (float) User_Time;
|
|
#else
|
|
Microseconds = (float) User_Time * Mic_secs_Per_Second
|
|
/ ((float) HZ * ((float) Number_Of_Runs));
|
|
Dhrystones_Per_Second = ((float) HZ * (float) Number_Of_Runs)
|
|
/ (float) User_Time;
|
|
#endif
|
|
printf ("Microseconds for one run through Dhrystone: ");
|
|
printf ("%6.1f \n", Microseconds);
|
|
printf ("Dhrystones per Second: ");
|
|
printf ("%6.1f \n", Dhrystones_Per_Second);
|
|
printf ("\n");
|
|
}
|
|
#endif /* SELF_TIMED */
|
|
}
|
|
|
|
|
|
void Proc_1 (REG Rec_Pointer Ptr_Val_Par)
|
|
/* executed once */
|
|
{
|
|
REG Rec_Pointer Next_Record = Ptr_Val_Par->Ptr_Comp;
|
|
/* == Ptr_Glob_Next */
|
|
/* Local variable, initialized with Ptr_Val_Par->Ptr_Comp, */
|
|
/* corresponds to "rename" in Ada, "with" in Pascal */
|
|
|
|
structassign (*Ptr_Val_Par->Ptr_Comp, *Ptr_Glob);
|
|
Ptr_Val_Par->variant.var_1.Int_Comp = 5;
|
|
Next_Record->variant.var_1.Int_Comp
|
|
= Ptr_Val_Par->variant.var_1.Int_Comp;
|
|
Next_Record->Ptr_Comp = Ptr_Val_Par->Ptr_Comp;
|
|
Proc_3 (&Next_Record->Ptr_Comp);
|
|
/* Ptr_Val_Par->Ptr_Comp->Ptr_Comp
|
|
== Ptr_Glob->Ptr_Comp */
|
|
if (Next_Record->Discr == Ident_1)
|
|
/* then, executed */
|
|
{
|
|
Next_Record->variant.var_1.Int_Comp = 6;
|
|
Proc_6 (Ptr_Val_Par->variant.var_1.Enum_Comp,
|
|
&Next_Record->variant.var_1.Enum_Comp);
|
|
Next_Record->Ptr_Comp = Ptr_Glob->Ptr_Comp;
|
|
Proc_7 (Next_Record->variant.var_1.Int_Comp, 10,
|
|
&Next_Record->variant.var_1.Int_Comp);
|
|
}
|
|
else /* not executed */
|
|
structassign (*Ptr_Val_Par, *Ptr_Val_Par->Ptr_Comp);
|
|
} /* Proc_1 */
|
|
|
|
|
|
void Proc_2 (One_Fifty *Int_Par_Ref)
|
|
/* executed once */
|
|
/* *Int_Par_Ref == 1, becomes 4 */
|
|
{
|
|
One_Fifty Int_Loc;
|
|
Enumeration Enum_Loc;
|
|
|
|
Enum_Loc = 0;
|
|
|
|
Int_Loc = *Int_Par_Ref + 10;
|
|
do /* executed once */
|
|
if (Ch_1_Glob == 'A')
|
|
/* then, executed */
|
|
{
|
|
Int_Loc -= 1;
|
|
*Int_Par_Ref = Int_Loc - Int_Glob;
|
|
Enum_Loc = Ident_1;
|
|
} /* if */
|
|
while (Enum_Loc != Ident_1); /* true */
|
|
} /* Proc_2 */
|
|
|
|
|
|
void Proc_3 (Rec_Pointer *Ptr_Ref_Par)
|
|
/* executed once */
|
|
/* Ptr_Ref_Par becomes Ptr_Glob */
|
|
{
|
|
if (Ptr_Glob != Null)
|
|
/* then, executed */
|
|
*Ptr_Ref_Par = Ptr_Glob->Ptr_Comp;
|
|
Proc_7 (10, Int_Glob, &Ptr_Glob->variant.var_1.Int_Comp);
|
|
} /* Proc_3 */
|
|
|
|
|
|
void Proc_4 (void) /* without parameters */
|
|
/* executed once */
|
|
{
|
|
Boolean Bool_Loc;
|
|
|
|
Bool_Loc = Ch_1_Glob == 'A';
|
|
Bool_Glob = Bool_Loc | Bool_Glob;
|
|
Ch_2_Glob = 'B';
|
|
} /* Proc_4 */
|
|
|
|
void Proc_5 (void) /* without parameters */
|
|
/*******/
|
|
/* executed once */
|
|
{
|
|
Ch_1_Glob = 'A';
|
|
Bool_Glob = false;
|
|
} /* Proc_5 */
|
|
|
|
|
|
/* Procedure for the assignment of structures, */
|
|
/* if the C compiler doesn't support this feature */
|
|
#ifdef NOSTRUCTASSIGN
|
|
memcpy (d, s, l)
|
|
register char *d;
|
|
register char *s;
|
|
register int l;
|
|
{
|
|
while (l--) *d++ = *s++;
|
|
}
|
|
#endif
|