mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
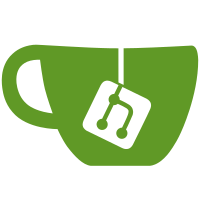
This construct is quite long-winded. In earlier days it made some sense since auto-allocation was a strange concept. But with driver model now used pretty universally, we can shorten this to 'auto'. This reduces verbosity and makes it easier to read. Coincidentally it also ensures that every declaration is on one line, thus making dtoc's job easier. Signed-off-by: Simon Glass <sjg@chromium.org>
93 lines
1.8 KiB
C
93 lines
1.8 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright (C) 2018 Álvaro Fernández Rojas <noltari@gmail.com>
|
|
*
|
|
* Derived from linux/arch/mips/bcm63xx/usb-common.c:
|
|
* Copyright 2008 Maxime Bizon <mbizon@freebox.fr>
|
|
* Copyright 2013 Florian Fainelli <florian@openwrt.org>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <clk.h>
|
|
#include <dm.h>
|
|
#include <generic-phy.h>
|
|
#include <log.h>
|
|
#include <malloc.h>
|
|
#include <reset.h>
|
|
#include <asm/io.h>
|
|
#include <dm/device.h>
|
|
#include <linux/bitops.h>
|
|
|
|
#define USBH_SETUP_PORT1_EN BIT(0)
|
|
|
|
struct bcm6348_usbh_priv {
|
|
void __iomem *regs;
|
|
};
|
|
|
|
static int bcm6348_usbh_init(struct phy *phy)
|
|
{
|
|
struct bcm6348_usbh_priv *priv = dev_get_priv(phy->dev);
|
|
|
|
writel_be(USBH_SETUP_PORT1_EN, priv->regs);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static struct phy_ops bcm6348_usbh_ops = {
|
|
.init = bcm6348_usbh_init,
|
|
};
|
|
|
|
static const struct udevice_id bcm6348_usbh_ids[] = {
|
|
{ .compatible = "brcm,bcm6348-usbh" },
|
|
{ /* sentinel */ }
|
|
};
|
|
|
|
static int bcm6348_usbh_probe(struct udevice *dev)
|
|
{
|
|
struct bcm6348_usbh_priv *priv = dev_get_priv(dev);
|
|
struct reset_ctl rst_ctl;
|
|
struct clk clk;
|
|
int ret;
|
|
|
|
priv->regs = dev_remap_addr(dev);
|
|
if (!priv->regs)
|
|
return -EINVAL;
|
|
|
|
/* enable usbh clock */
|
|
ret = clk_get_by_name(dev, "usbh", &clk);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
ret = clk_enable(&clk);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
ret = clk_free(&clk);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
/* perform reset */
|
|
ret = reset_get_by_index(dev, 0, &rst_ctl);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
ret = reset_deassert(&rst_ctl);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
ret = reset_free(&rst_ctl);
|
|
if (ret < 0)
|
|
return ret;
|
|
|
|
return 0;
|
|
}
|
|
|
|
U_BOOT_DRIVER(bcm6348_usbh) = {
|
|
.name = "bcm6348-usbh",
|
|
.id = UCLASS_PHY,
|
|
.of_match = bcm6348_usbh_ids,
|
|
.ops = &bcm6348_usbh_ops,
|
|
.priv_auto = sizeof(struct bcm6348_usbh_priv),
|
|
.probe = bcm6348_usbh_probe,
|
|
};
|