mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-07 03:36:16 +09:00
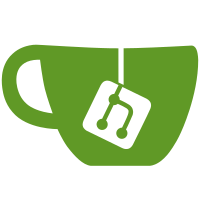
Generate a MAC address based on the cpuid available in the efuse block: Use the first 6 byte of the cpuid's SHA256 hash and set the locally administered bits. Also ensure that the multicast bit is cleared. The MAC address is only generated and set if there is no ethaddr present in the saved environment. This is based off of Klaus Goger's work in 8adc9d Signed-off-by: Rohan Garg <rohan.garg@collabora.com> Reviewed-by: Kever Yang <kever.yang@rock-chips.com>
128 lines
2.4 KiB
C
128 lines
2.4 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* (C) Copyright 2019 Rockchip Electronics Co., Ltd.
|
|
*/
|
|
#include <common.h>
|
|
#include <clk.h>
|
|
#include <dm.h>
|
|
#include <ram.h>
|
|
#include <syscon.h>
|
|
#include <asm/io.h>
|
|
#include <asm/arch-rockchip/boot_mode.h>
|
|
#include <asm/arch-rockchip/clock.h>
|
|
#include <asm/arch-rockchip/periph.h>
|
|
#include <asm/arch-rockchip/misc.h>
|
|
#include <power/regulator.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
__weak int rk_board_late_init(void)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
int board_late_init(void)
|
|
{
|
|
setup_boot_mode();
|
|
|
|
return rk_board_late_init();
|
|
}
|
|
|
|
int board_init(void)
|
|
{
|
|
int ret;
|
|
|
|
#ifdef CONFIG_DM_REGULATOR
|
|
ret = regulators_enable_boot_on(false);
|
|
if (ret)
|
|
debug("%s: Cannot enable boot on regulator\n", __func__);
|
|
#endif
|
|
|
|
return 0;
|
|
}
|
|
|
|
#if !defined(CONFIG_SYS_DCACHE_OFF) && !defined(CONFIG_ARM64)
|
|
void enable_caches(void)
|
|
{
|
|
/* Enable D-cache. I-cache is already enabled in start.S */
|
|
dcache_enable();
|
|
}
|
|
#endif
|
|
|
|
#if defined(CONFIG_USB_GADGET) && defined(CONFIG_USB_GADGET_DWC2_OTG)
|
|
#include <usb.h>
|
|
#include <usb/dwc2_udc.h>
|
|
|
|
static struct dwc2_plat_otg_data otg_data = {
|
|
.rx_fifo_sz = 512,
|
|
.np_tx_fifo_sz = 16,
|
|
.tx_fifo_sz = 128,
|
|
};
|
|
|
|
int board_usb_init(int index, enum usb_init_type init)
|
|
{
|
|
int node;
|
|
const char *mode;
|
|
bool matched = false;
|
|
const void *blob = gd->fdt_blob;
|
|
|
|
/* find the usb_otg node */
|
|
node = fdt_node_offset_by_compatible(blob, -1, "snps,dwc2");
|
|
|
|
while (node > 0) {
|
|
mode = fdt_getprop(blob, node, "dr_mode", NULL);
|
|
if (mode && strcmp(mode, "otg") == 0) {
|
|
matched = true;
|
|
break;
|
|
}
|
|
|
|
node = fdt_node_offset_by_compatible(blob, node, "snps,dwc2");
|
|
}
|
|
if (!matched) {
|
|
debug("Not found usb_otg device\n");
|
|
return -ENODEV;
|
|
}
|
|
otg_data.regs_otg = fdtdec_get_addr(blob, node, "reg");
|
|
|
|
return dwc2_udc_probe(&otg_data);
|
|
}
|
|
|
|
int board_usb_cleanup(int index, enum usb_init_type init)
|
|
{
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
#if CONFIG_IS_ENABLED(FASTBOOT)
|
|
int fastboot_set_reboot_flag(void)
|
|
{
|
|
printf("Setting reboot to fastboot flag ...\n");
|
|
/* Set boot mode to fastboot */
|
|
writel(BOOT_FASTBOOT, CONFIG_ROCKCHIP_BOOT_MODE_REG);
|
|
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
#ifdef CONFIG_MISC_INIT_R
|
|
__weak int misc_init_r(void)
|
|
{
|
|
const u32 cpuid_offset = 0x7;
|
|
const u32 cpuid_length = 0x10;
|
|
u8 cpuid[cpuid_length];
|
|
int ret;
|
|
|
|
ret = rockchip_cpuid_from_efuse(cpuid_offset, cpuid_length, cpuid);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = rockchip_cpuid_set(cpuid, cpuid_length);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = rockchip_setup_macaddr();
|
|
|
|
return ret;
|
|
}
|
|
#endif
|