mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-30 23:03:45 +09:00
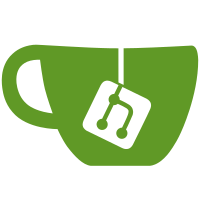
When disabled the PIT runs until it reaches the CPIV value. The Linux PIT driver stops the PIT and waits until it stopped. This can take over 100ms. Simply stopping in u-boot isn't sufficient as the PIT will still be running when Linux is waiting until it stopped. So, we stop it in u-boot by setting the compare value to a value slightly greater than the current running counter to make the PIT stopped in short time. Signed-off-by: Alexander Stein <alexander.stein@systec-electronic.com>
101 lines
2.6 KiB
C
101 lines
2.6 KiB
C
/*
|
|
* (C) Copyright 2010
|
|
* Reinhard Meyer, reinhard.meyer@emk-elektronik.de
|
|
* (C) Copyright 2009
|
|
* Jean-Christophe PLAGNIOL-VILLARD <plagnioj@jcrosoft.com>
|
|
*
|
|
* See file CREDITS for list of people who contributed to this
|
|
* project.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston,
|
|
* MA 02111-1307 USA
|
|
*/
|
|
|
|
#include <common.h>
|
|
|
|
#include <asm/arch/hardware.h>
|
|
#include <asm/arch/at91_pmc.h>
|
|
#include <asm/arch/at91_pit.h>
|
|
#include <asm/arch/at91_gpbr.h>
|
|
#include <asm/arch/clk.h>
|
|
#include <asm/arch/io.h>
|
|
|
|
#ifndef CONFIG_SYS_AT91_MAIN_CLOCK
|
|
#define CONFIG_SYS_AT91_MAIN_CLOCK 0
|
|
#endif
|
|
|
|
int arch_cpu_init(void)
|
|
{
|
|
return at91_clock_init(CONFIG_SYS_AT91_MAIN_CLOCK);
|
|
}
|
|
|
|
void arch_preboot_os(void)
|
|
{
|
|
ulong cpiv;
|
|
at91_pit_t *pit = (at91_pit_t *) AT91_PIT_BASE;
|
|
|
|
cpiv = AT91_PIT_MR_PIV_MASK(readl(&pit->piir));
|
|
|
|
/*
|
|
* Disable PITC
|
|
* Add 0x1000 to current counter to stop it faster
|
|
* without waiting for wrapping back to 0
|
|
*/
|
|
writel(cpiv + 0x1000, &pit->mr);
|
|
}
|
|
|
|
#if defined(CONFIG_DISPLAY_CPUINFO)
|
|
int print_cpuinfo(void)
|
|
{
|
|
char buf[32];
|
|
|
|
printf("CPU: %s\n", CONFIG_SYS_AT91_CPU_NAME);
|
|
printf("Crystal frequency: %8s MHz\n",
|
|
strmhz(buf, get_main_clk_rate()));
|
|
printf("CPU clock : %8s MHz\n",
|
|
strmhz(buf, get_cpu_clk_rate()));
|
|
printf("Master clock : %8s MHz\n",
|
|
strmhz(buf, get_mck_clk_rate()));
|
|
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
#ifdef CONFIG_BOOTCOUNT_LIMIT
|
|
/*
|
|
* We combine the BOOTCOUNT_MAGIC and bootcount in one 32-bit register.
|
|
* This is done so we need to use only one of the four GPBR registers.
|
|
*/
|
|
void bootcount_store (ulong a)
|
|
{
|
|
at91_gpbr_t *gpbr = (at91_gpbr_t *) AT91_GPR_BASE;
|
|
|
|
writel((BOOTCOUNT_MAGIC & 0xffff0000) | (a & 0x0000ffff),
|
|
&gpbr->reg[AT91_GPBR_INDEX_BOOTCOUNT]);
|
|
}
|
|
|
|
ulong bootcount_load (void)
|
|
{
|
|
at91_gpbr_t *gpbr = (at91_gpbr_t *) AT91_GPR_BASE;
|
|
|
|
ulong val = readl(&gpbr->reg[AT91_GPBR_INDEX_BOOTCOUNT]);
|
|
if ((val & 0xffff0000) != (BOOTCOUNT_MAGIC & 0xffff0000))
|
|
return 0;
|
|
else
|
|
return val & 0x0000ffff;
|
|
}
|
|
|
|
#endif /* CONFIG_BOOTCOUNT_LIMIT */
|