mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-05 18:56:15 +09:00
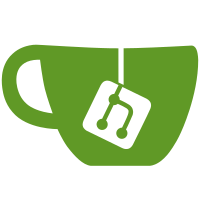
Add libavb lib (3rd party library from AOSP), that implements support of AVB 2.0. This library is used for integrity checking of Android partitions on eMMC. libavb was added as it is and minimal changes were introduced to reduce maintenance cost, because it will be deviated from AOSP upstream in the future. Changes: - license headers changed to conform SPDX-style - avb_crc32.c dropped - updates in avb_sysdeps_posix.c/avb_sysdeps.h For additional details check [1] AVB 2.0 README. [1] https://android.googlesource.com/platform/external/avb/+/master/README.md Signed-off-by: Igor Opaniuk <igor.opaniuk@linaro.org>
64 lines
1.1 KiB
C
64 lines
1.1 KiB
C
/*
|
|
* Copyright (C) 2016 The Android Open Source Project
|
|
*
|
|
* SPDX-License-Identifier: MIT
|
|
*/
|
|
|
|
#include <stdarg.h>
|
|
#include <stdlib.h>
|
|
|
|
#include "avb_sysdeps.h"
|
|
|
|
int avb_memcmp(const void* src1, const void* src2, size_t n) {
|
|
return memcmp(src1, src2, n);
|
|
}
|
|
|
|
void* avb_memcpy(void* dest, const void* src, size_t n) {
|
|
return memcpy(dest, src, n);
|
|
}
|
|
|
|
void* avb_memset(void* dest, const int c, size_t n) {
|
|
return memset(dest, c, n);
|
|
}
|
|
|
|
int avb_strcmp(const char* s1, const char* s2) {
|
|
return strcmp(s1, s2);
|
|
}
|
|
|
|
size_t avb_strlen(const char* str) {
|
|
return strlen(str);
|
|
}
|
|
|
|
uint32_t avb_div_by_10(uint64_t* dividend) {
|
|
uint32_t rem = (uint32_t)(*dividend % 10);
|
|
*dividend /= 10;
|
|
return rem;
|
|
}
|
|
|
|
void avb_abort(void) {
|
|
hang();
|
|
}
|
|
|
|
void avb_print(const char* message) {
|
|
printf("%s", message);
|
|
}
|
|
|
|
void avb_printv(const char* message, ...) {
|
|
va_list ap;
|
|
const char* m;
|
|
|
|
va_start(ap, message);
|
|
for (m = message; m != NULL; m = va_arg(ap, const char*)) {
|
|
printf("%s", m);
|
|
}
|
|
va_end(ap);
|
|
}
|
|
|
|
void* avb_malloc_(size_t size) {
|
|
return malloc(size);
|
|
}
|
|
|
|
void avb_free(void* ptr) {
|
|
free(ptr);
|
|
}
|