mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-06 03:06:16 +09:00
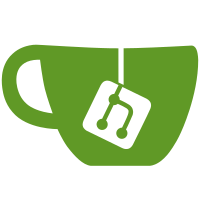
We are about to add support for the Amlogic AXG SoC. While very close to the Gx SoC family, we will need to handle a few thing which are different in this SoC. Rework the meson arch directory to prepare for this. Signed-off-by: Jerome Brunet <jbrunet@baylibre.com> Signed-off-by: Neil Armstrong <narmstrong@baylibre.com>
56 lines
1.1 KiB
C
56 lines
1.1 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* (C) Copyright 2016 Beniamino Galvani <b.galvani@gmail.com>
|
|
*
|
|
* Secure monitor calls.
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <linux/kernel.h>
|
|
|
|
#define FN_GET_SHARE_MEM_INPUT_BASE 0x82000020
|
|
#define FN_GET_SHARE_MEM_OUTPUT_BASE 0x82000021
|
|
#define FN_EFUSE_READ 0x82000030
|
|
#define FN_EFUSE_WRITE 0x82000031
|
|
|
|
static void *shmem_input;
|
|
static void *shmem_output;
|
|
|
|
static void meson_init_shmem(void)
|
|
{
|
|
struct pt_regs regs;
|
|
|
|
if (shmem_input && shmem_output)
|
|
return;
|
|
|
|
regs.regs[0] = FN_GET_SHARE_MEM_INPUT_BASE;
|
|
smc_call(®s);
|
|
shmem_input = (void *)regs.regs[0];
|
|
|
|
regs.regs[0] = FN_GET_SHARE_MEM_OUTPUT_BASE;
|
|
smc_call(®s);
|
|
shmem_output = (void *)regs.regs[0];
|
|
|
|
debug("Secure Monitor shmem: 0x%p 0x%p\n", shmem_input, shmem_output);
|
|
}
|
|
|
|
ssize_t meson_sm_read_efuse(uintptr_t offset, void *buffer, size_t size)
|
|
{
|
|
struct pt_regs regs;
|
|
|
|
meson_init_shmem();
|
|
|
|
regs.regs[0] = FN_EFUSE_READ;
|
|
regs.regs[1] = offset;
|
|
regs.regs[2] = size;
|
|
|
|
smc_call(®s);
|
|
|
|
if (regs.regs[0] == 0)
|
|
return -1;
|
|
|
|
memcpy(buffer, shmem_output, min(size, regs.regs[0]));
|
|
|
|
return regs.regs[0];
|
|
}
|