mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
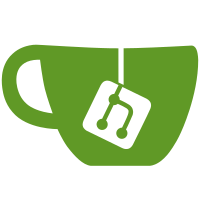
Currently on Armada-37xx the mem_map structure is statically defined to map first 2 GB of memory as RAM region, and system registers and PCIe region device region. This is insufficient for when there is more RAM or when for example the PCIe windows is mapped to another address by the CPU Address Decoder. In the case when the board has 4 GB RAM, on some boards the ARM Trusted Firmware can move the PCIe window to another address, in order to maximize possible usable RAM. Also the dram_init and dram_init_banksize looks for information in device-tree, and therefore different device trees are needed for boards with different RAM sizes. Therefore we add code that looks at how the ARM Trusted Firmware has configured the CPU Address Decoder windows, and then we update the mem_map structure and compute gd->ram_size and gd->bd->bi_dram bank base addresses and sizes accordingly. Signed-off-by: Marek Behún <marek.behun@nic.cz> Reviewed-by: Stefan Roese <sr@denx.de>
111 lines
2.3 KiB
C
111 lines
2.3 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright (C) 2016 Stefan Roese <sr@denx.de>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <dm.h>
|
|
#include <fdtdec.h>
|
|
#include <init.h>
|
|
#include <linux/libfdt.h>
|
|
#include <linux/sizes.h>
|
|
#include <pci.h>
|
|
#include <asm/io.h>
|
|
#include <asm/system.h>
|
|
#include <asm/arch/cpu.h>
|
|
#include <asm/arch/soc.h>
|
|
#include <asm/armv8/mmu.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
/*
|
|
* Not all memory is mapped in the MMU. So we need to restrict the
|
|
* memory size so that U-Boot does not try to access it. Also, the
|
|
* internal registers are located at 0xf000.0000 - 0xffff.ffff.
|
|
* Currently only 2GiB are mapped for system memory. This is what
|
|
* we pass to the U-Boot subsystem here.
|
|
*/
|
|
#define USABLE_RAM_SIZE 0x80000000
|
|
|
|
ulong board_get_usable_ram_top(ulong total_size)
|
|
{
|
|
if (gd->ram_size > USABLE_RAM_SIZE)
|
|
return USABLE_RAM_SIZE;
|
|
|
|
return gd->ram_size;
|
|
}
|
|
|
|
/*
|
|
* On ARMv8, MBus is not configured in U-Boot. To enable compilation
|
|
* of the already implemented drivers, lets add a dummy version of
|
|
* this function so that linking does not fail.
|
|
*/
|
|
const struct mbus_dram_target_info *mvebu_mbus_dram_info(void)
|
|
{
|
|
return NULL;
|
|
}
|
|
|
|
__weak int dram_init_banksize(void)
|
|
{
|
|
if (CONFIG_IS_ENABLED(ARMADA_8K))
|
|
return a8k_dram_init_banksize();
|
|
else if (CONFIG_IS_ENABLED(ARMADA_3700))
|
|
return a3700_dram_init_banksize();
|
|
else
|
|
return fdtdec_setup_memory_banksize();
|
|
}
|
|
|
|
__weak int dram_init(void)
|
|
{
|
|
if (CONFIG_IS_ENABLED(ARMADA_8K)) {
|
|
gd->ram_size = a8k_dram_scan_ap_sz();
|
|
if (gd->ram_size != 0)
|
|
return 0;
|
|
}
|
|
|
|
if (CONFIG_IS_ENABLED(ARMADA_3700))
|
|
return a3700_dram_init();
|
|
|
|
if (fdtdec_setup_mem_size_base() != 0)
|
|
return -EINVAL;
|
|
|
|
return 0;
|
|
}
|
|
|
|
int arch_cpu_init(void)
|
|
{
|
|
/* Nothing to do (yet) */
|
|
return 0;
|
|
}
|
|
|
|
int arch_early_init_r(void)
|
|
{
|
|
struct udevice *dev;
|
|
int ret;
|
|
int i;
|
|
|
|
/*
|
|
* Loop over all MISC uclass drivers to call the comphy code
|
|
* and init all CP110 devices enabled in the DT
|
|
*/
|
|
i = 0;
|
|
while (1) {
|
|
/* Call the comphy code via the MISC uclass driver */
|
|
ret = uclass_get_device(UCLASS_MISC, i++, &dev);
|
|
|
|
/* We're done, once no further CP110 device is found */
|
|
if (ret)
|
|
break;
|
|
}
|
|
|
|
/* Cause the SATA device to do its early init */
|
|
uclass_first_device(UCLASS_AHCI, &dev);
|
|
|
|
#ifdef CONFIG_DM_PCI
|
|
/* Trigger PCIe devices detection */
|
|
pci_init();
|
|
#endif
|
|
|
|
return 0;
|
|
}
|