mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
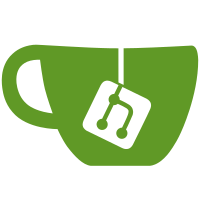
Currently the FSP execution environment GDT is setup by U-Boot in arch/x86/cpu/start16.S, which works pretty well. But if we try to move the FspInitEntry call a little bit later to better fit into U-Boot's initialization sequence, FSP will fail to bring up the AP due to #GP fault as AP's GDT is duplicated from BSP whose GDT is now moved into CAR, and unfortunately FSP calls AP initialization after it disables the CAR. So basically the BSP's GDT still refers to the one in the CAR, whose content is no longer available, so when AP starts up and loads its segment register, it blows up. To resolve this, we load GDT before calling into FspInitEntry. The GDT is the same one used in arch/x86/cpu/start16.S, which is in the ROM and exists forever. Signed-off-by: Bin Meng <bmeng.cn@gmail.com> Tested-by: Andrew Bradford <andrew.bradford@kodakalaris.com> Tested-by: Simon Glass <sjg@chromium.org> Acked-by: Simon Glass <sjg@chromium.org>
132 lines
2.9 KiB
ArmAsm
132 lines
2.9 KiB
ArmAsm
/*
|
|
* U-Boot - x86 Startup Code
|
|
*
|
|
* (C) Copyright 2008-2011
|
|
* Graeme Russ, <graeme.russ@gmail.com>
|
|
*
|
|
* (C) Copyright 2002,2003
|
|
* Daniel Engström, Omicron Ceti AB, <daniel@omicron.se>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <asm/global_data.h>
|
|
#include <asm/processor-flags.h>
|
|
|
|
#define BOOT_SEG 0xffff0000 /* linear segment of boot code */
|
|
#define a32 .byte 0x67;
|
|
#define o32 .byte 0x66;
|
|
|
|
.section .start16, "ax"
|
|
.code16
|
|
.globl start16
|
|
start16:
|
|
/* Save BIST */
|
|
movl %eax, %ecx
|
|
|
|
/* Set the Cold Boot / Hard Reset flag */
|
|
movl $GD_FLG_COLD_BOOT, %ebx
|
|
|
|
xorl %eax, %eax
|
|
movl %eax, %cr3 /* Invalidate TLB */
|
|
|
|
/* Turn off cache (this might require a 486-class CPU) */
|
|
movl %cr0, %eax
|
|
orl $(X86_CR0_NW | X86_CR0_CD), %eax
|
|
movl %eax, %cr0
|
|
wbinvd
|
|
|
|
/* load the temporary Global Descriptor Table */
|
|
o32 cs lidt idt_ptr
|
|
o32 cs lgdt gdt_ptr
|
|
|
|
/* Now, we enter protected mode */
|
|
movl %cr0, %eax
|
|
orl $X86_CR0_PE, %eax
|
|
movl %eax, %cr0
|
|
|
|
/* Flush the prefetch queue */
|
|
jmp ff
|
|
ff:
|
|
|
|
/* Finally restore BIST and jump to the 32-bit initialization code */
|
|
movw $code32start, %ax
|
|
movw %ax, %bp
|
|
movl %ecx, %eax
|
|
o32 cs ljmp *(%bp)
|
|
|
|
/* 48-bit far pointer */
|
|
code32start:
|
|
.long _start /* offset */
|
|
.word 0x10 /* segment */
|
|
|
|
idt_ptr:
|
|
.word 0 /* limit */
|
|
.long 0 /* base */
|
|
|
|
/*
|
|
* The following Global Descriptor Table is just enough to get us into
|
|
* 'Flat Protected Mode' - It will be discarded as soon as the final
|
|
* GDT is setup in a safe location in RAM
|
|
*/
|
|
gdt_ptr:
|
|
.word 0x1f /* limit (31 bytes = 4 GDT entries - 1) */
|
|
.long BOOT_SEG + gdt_rom /* base */
|
|
|
|
/* Some CPUs are picky about GDT alignment... */
|
|
.align 16
|
|
.globl gdt_rom
|
|
gdt_rom:
|
|
/*
|
|
* The GDT table ...
|
|
*
|
|
* Selector Type
|
|
* 0x00 NULL
|
|
* 0x08 Unused
|
|
* 0x10 32bit code
|
|
* 0x18 32bit data/stack
|
|
*/
|
|
/* The NULL Desciptor - Mandatory */
|
|
.word 0x0000 /* limit_low */
|
|
.word 0x0000 /* base_low */
|
|
.byte 0x00 /* base_middle */
|
|
.byte 0x00 /* access */
|
|
.byte 0x00 /* flags + limit_high */
|
|
.byte 0x00 /* base_high */
|
|
|
|
/* Unused Desciptor - (matches Linux) */
|
|
.word 0x0000 /* limit_low */
|
|
.word 0x0000 /* base_low */
|
|
.byte 0x00 /* base_middle */
|
|
.byte 0x00 /* access */
|
|
.byte 0x00 /* flags + limit_high */
|
|
.byte 0x00 /* base_high */
|
|
|
|
/*
|
|
* The Code Segment Descriptor:
|
|
* - Base = 0x00000000
|
|
* - Size = 4GB
|
|
* - Access = Present, Ring 0, Exec (Code), Readable
|
|
* - Flags = 4kB Granularity, 32-bit
|
|
*/
|
|
.word 0xffff /* limit_low */
|
|
.word 0x0000 /* base_low */
|
|
.byte 0x00 /* base_middle */
|
|
.byte 0x9b /* access */
|
|
.byte 0xcf /* flags + limit_high */
|
|
.byte 0x00 /* base_high */
|
|
|
|
/*
|
|
* The Data Segment Descriptor:
|
|
* - Base = 0x00000000
|
|
* - Size = 4GB
|
|
* - Access = Present, Ring 0, Non-Exec (Data), Writable
|
|
* - Flags = 4kB Granularity, 32-bit
|
|
*/
|
|
.word 0xffff /* limit_low */
|
|
.word 0x0000 /* base_low */
|
|
.byte 0x00 /* base_middle */
|
|
.byte 0x93 /* access */
|
|
.byte 0xcf /* flags + limit_high */
|
|
.byte 0x00 /* base_high */
|