mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
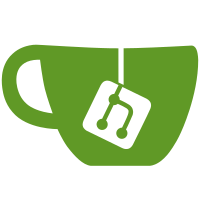
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
110 lines
2.7 KiB
C
110 lines
2.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* Copyright 2008-2014 Freescale Semiconductor, Inc.
|
|
*
|
|
*/
|
|
|
|
#ifndef __JR_H
|
|
#define __JR_H
|
|
|
|
#include <linux/compiler.h>
|
|
|
|
#define JR_SIZE 4
|
|
/* Timeout currently defined as 90 sec */
|
|
#define CONFIG_SEC_DEQ_TIMEOUT 90000000U
|
|
|
|
#define DEFAULT_JR_ID 0
|
|
#define DEFAULT_JR_LIODN 0
|
|
#define DEFAULT_IRQ 0 /* Interrupts not to be configured */
|
|
|
|
#define MCFGR_SWRST ((uint32_t)(1)<<31) /* Software Reset */
|
|
#define MCFGR_DMA_RST ((uint32_t)(1)<<28) /* DMA Reset */
|
|
#define MCFGR_PS_SHIFT 16
|
|
#define MCFGR_AWCACHE_SHIFT 8
|
|
#define MCFGR_AWCACHE_MASK (0xf << MCFGR_AWCACHE_SHIFT)
|
|
#define MCFGR_ARCACHE_SHIFT 12
|
|
#define MCFGR_ARCACHE_MASK (0xf << MCFGR_ARCACHE_SHIFT)
|
|
|
|
#define JR_INTMASK 0x00000001
|
|
#define JRCR_RESET 0x01
|
|
#define JRINT_ERR_HALT_INPROGRESS 0x4
|
|
#define JRINT_ERR_HALT_MASK 0xc
|
|
#define JRNSLIODN_SHIFT 16
|
|
#define JRNSLIODN_MASK 0x0fff0000
|
|
#define JRSLIODN_SHIFT 0
|
|
#define JRSLIODN_MASK 0x00000fff
|
|
#define JROWN_NS 0x00000008
|
|
#define JRMID_NS 0x00000001
|
|
|
|
#define JQ_DEQ_ERR -1
|
|
#define JQ_DEQ_TO_ERR -2
|
|
#define JQ_ENQ_ERR -3
|
|
|
|
#define RNG4_MAX_HANDLES 2
|
|
|
|
struct op_ring {
|
|
phys_addr_t desc;
|
|
uint32_t status;
|
|
} __packed;
|
|
|
|
struct jr_info {
|
|
void (*callback)(uint32_t status, void *arg);
|
|
phys_addr_t desc_phys_addr;
|
|
uint32_t desc_len;
|
|
uint32_t op_done;
|
|
void *arg;
|
|
};
|
|
|
|
struct jobring {
|
|
int jq_id;
|
|
int irq;
|
|
int liodn;
|
|
/* Head is the index where software would enq the descriptor in
|
|
* the i/p ring
|
|
*/
|
|
int head;
|
|
/* Tail index would be used by s/w ehile enqueuing to determine if
|
|
* there is any space left in the s/w maintained i/p rings
|
|
*/
|
|
/* Also in case of deq tail will be incremented only in case of
|
|
* in-order job completion
|
|
*/
|
|
int tail;
|
|
/* Read index of the output ring. It may not match with tail in case
|
|
* of out of order completetion
|
|
*/
|
|
int read_idx;
|
|
/* Write index to input ring. Would be always equal to head */
|
|
int write_idx;
|
|
/* Size of the rings. */
|
|
int size;
|
|
/* Op ring size aligned to cache line size */
|
|
int op_size;
|
|
/* The ip and output rings have to be accessed by SEC. So the
|
|
* pointers will ahve to point to the housekeeping region provided
|
|
* by SEC
|
|
*/
|
|
/*Circular Ring of i/p descriptors */
|
|
dma_addr_t *input_ring;
|
|
/* Circular Ring of o/p descriptors */
|
|
/* Circula Ring containing info regarding descriptors in i/p
|
|
* and o/p ring
|
|
*/
|
|
/* This ring can be on the stack */
|
|
struct jr_info info[JR_SIZE];
|
|
struct op_ring *output_ring;
|
|
/* Offset in CCSR to the SEC engine to which this JR belongs */
|
|
uint32_t sec_offset;
|
|
|
|
};
|
|
|
|
struct result {
|
|
int done;
|
|
uint32_t status;
|
|
};
|
|
|
|
void caam_jr_strstatus(u32 status);
|
|
int run_descriptor_jr(uint32_t *desc);
|
|
|
|
#endif
|