mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
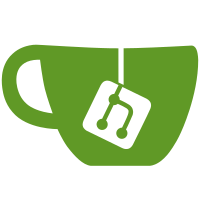
In cmd/fpga.c the commands should return enum command_ret_t, e.g. CMD_RET_USAGE, CMD_RET_SUCCESS, or CMD_RET_FAILURE. What they actually do is passing a return value from different 'fpga_' functions. Passing on a return value of -1 from a called function leads to printing out usage text. In case of actually correct usage with correctly specified parameters but some fail at runtime printing out that usage text is distracting. The reason is most 'fpga_' functions return either FPGA_SUCCESS or FPGA_FAIL, the latter was equal to -1 which is the same value as CMD_RET_USAGE. So just passing on FPGA_FAIL lead to printing out usage. We should only return CMD_RET_USAGE in cases, where the user sent wrong input. Every other case should return CMD_RET_SUCCESS or CMD_RET_FAILURE, and not simply pass an error code. Simply changing FPGA_FAIL from -1 to 1 gets the job done. Suggested-by: Michal Simek <michal.simek@xilinx.com> Signed-off-by: Alexander Dahl <ada@thorsis.com>
80 lines
2.1 KiB
C
80 lines
2.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* (C) Copyright 2002
|
|
* Rich Ireland, Enterasys Networks, rireland@enterasys.com.
|
|
*/
|
|
|
|
#include <linux/types.h> /* for ulong typedef */
|
|
|
|
#ifndef _FPGA_H_
|
|
#define _FPGA_H_
|
|
|
|
#ifndef CONFIG_MAX_FPGA_DEVICES
|
|
#define CONFIG_MAX_FPGA_DEVICES 5
|
|
#endif
|
|
|
|
/* fpga_xxxx function return value definitions */
|
|
#define FPGA_SUCCESS 0
|
|
#define FPGA_FAIL 1
|
|
|
|
/* device numbers must be non-negative */
|
|
#define FPGA_INVALID_DEVICE -1
|
|
|
|
#define FPGA_ENC_USR_KEY 1
|
|
#define FPGA_NO_ENC_OR_NO_AUTH 2
|
|
|
|
/* root data type defintions */
|
|
typedef enum { /* typedef fpga_type */
|
|
fpga_min_type, /* range check value */
|
|
fpga_xilinx, /* Xilinx Family) */
|
|
fpga_altera, /* unimplemented */
|
|
fpga_lattice, /* Lattice family */
|
|
fpga_undefined /* invalid range check value */
|
|
} fpga_type; /* end, typedef fpga_type */
|
|
|
|
typedef struct { /* typedef fpga_desc */
|
|
fpga_type devtype; /* switch value to select sub-functions */
|
|
void *devdesc; /* real device descriptor */
|
|
} fpga_desc; /* end, typedef fpga_desc */
|
|
|
|
typedef struct { /* typedef fpga_desc */
|
|
unsigned int blocksize;
|
|
char *interface;
|
|
char *dev_part;
|
|
const char *filename;
|
|
int fstype;
|
|
} fpga_fs_info;
|
|
|
|
struct fpga_secure_info {
|
|
u8 *userkey_addr;
|
|
u8 authflag;
|
|
u8 encflag;
|
|
};
|
|
|
|
typedef enum {
|
|
BIT_FULL = 0,
|
|
BIT_PARTIAL,
|
|
BIT_NONE = 0xFF,
|
|
} bitstream_type;
|
|
|
|
/* root function definitions */
|
|
void fpga_init(void);
|
|
int fpga_add(fpga_type devtype, void *desc);
|
|
int fpga_count(void);
|
|
const fpga_desc *const fpga_get_desc(int devnum);
|
|
int fpga_is_partial_data(int devnum, size_t img_len);
|
|
int fpga_load(int devnum, const void *buf, size_t bsize,
|
|
bitstream_type bstype);
|
|
int fpga_fsload(int devnum, const void *buf, size_t size,
|
|
fpga_fs_info *fpga_fsinfo);
|
|
int fpga_loads(int devnum, const void *buf, size_t size,
|
|
struct fpga_secure_info *fpga_sec_info);
|
|
int fpga_loadbitstream(int devnum, char *fpgadata, size_t size,
|
|
bitstream_type bstype);
|
|
int fpga_dump(int devnum, const void *buf, size_t bsize);
|
|
int fpga_info(int devnum);
|
|
const fpga_desc *const fpga_validate(int devnum, const void *buf,
|
|
size_t bsize, char *fn);
|
|
|
|
#endif /* _FPGA_H_ */
|