mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
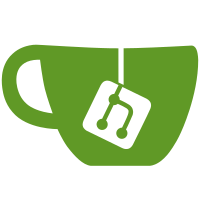
This patch basically adds two new commands for loadig secure images. 1. zynq rsa adds support to load secure image which can be both authenticated or encrypted or both authenticated and encrypted image in xilinx bootimage(BOOT.bin) format. 2. zynq aes command adds support to decrypt and load encrypted image back to DDR as per destination address. The image has to be encrypted using xilinx bootgen tool and to get only the encrypted image from tool use -split option while invoking bootgen. Signed-off-by: Siva Durga Prasad Paladugu <siva.durga.paladugu@xilinx.com> Signed-off-by: Michal Simek <michal.simek@xilinx.com>
79 lines
2.3 KiB
C
79 lines
2.3 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* Copyright 2014 Freescale Semiconductor, Inc.
|
|
*/
|
|
|
|
#ifndef _RSA_MOD_EXP_H
|
|
#define _RSA_MOD_EXP_H
|
|
|
|
#include <errno.h>
|
|
#include <image.h>
|
|
|
|
/**
|
|
* struct key_prop - holder for a public key properties
|
|
*
|
|
* The struct has pointers to modulus (Typically called N),
|
|
* The inverse, R^2, exponent. These can be typecasted and
|
|
* used as byte arrays or converted to the required format
|
|
* as per requirement of RSA implementation.
|
|
*/
|
|
struct key_prop {
|
|
const void *rr; /* R^2 can be treated as byte array */
|
|
const void *modulus; /* modulus as byte array */
|
|
const void *public_exponent; /* public exponent as byte array */
|
|
uint32_t n0inv; /* -1 / modulus[0] mod 2^32 */
|
|
int num_bits; /* Key length in bits */
|
|
uint32_t exp_len; /* Exponent length in number of uint8_t */
|
|
};
|
|
|
|
/**
|
|
* rsa_mod_exp_sw() - Perform RSA Modular Exponentiation in sw
|
|
*
|
|
* Operation: out[] = sig ^ exponent % modulus
|
|
*
|
|
* @sig: RSA PKCS1.5 signature
|
|
* @sig_len: Length of signature in number of bytes
|
|
* @node: Node with RSA key elements like modulus, exponent, R^2, n0inv
|
|
* @out: Result in form of byte array of len equal to sig_len
|
|
*/
|
|
int rsa_mod_exp_sw(const uint8_t *sig, uint32_t sig_len,
|
|
struct key_prop *node, uint8_t *out);
|
|
|
|
int rsa_mod_exp(struct udevice *dev, const uint8_t *sig, uint32_t sig_len,
|
|
struct key_prop *node, uint8_t *out);
|
|
|
|
#if defined(CONFIG_CMD_ZYNQ_RSA)
|
|
int zynq_pow_mod(u32 *keyptr, u32 *inout);
|
|
#endif
|
|
|
|
/**
|
|
* struct struct mod_exp_ops - Driver model for RSA Modular Exponentiation
|
|
* operations
|
|
*
|
|
* The uclass interface is implemented by all crypto devices which use
|
|
* driver model.
|
|
*/
|
|
struct mod_exp_ops {
|
|
/**
|
|
* Perform Modular Exponentiation
|
|
*
|
|
* Operation: out[] = sig ^ exponent % modulus
|
|
*
|
|
* @dev: RSA Device
|
|
* @sig: RSA PKCS1.5 signature
|
|
* @sig_len: Length of signature in number of bytes
|
|
* @node: Node with RSA key elements like modulus, exponent,
|
|
* R^2, n0inv
|
|
* @out: Result in form of byte array of len equal to sig_len
|
|
*
|
|
* This function computes exponentiation over the signature.
|
|
* Returns: 0 if exponentiation is successful, or a negative value
|
|
* if it wasn't.
|
|
*/
|
|
int (*mod_exp)(struct udevice *dev, const uint8_t *sig,
|
|
uint32_t sig_len, struct key_prop *node,
|
|
uint8_t *outp);
|
|
};
|
|
|
|
#endif
|