mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-06 19:26:16 +09:00
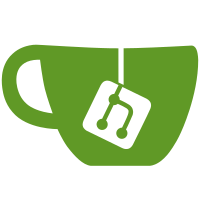
Make spl_*_load_image() functions return a value instead of hanging if a problem is encountered. This enables main spl code to make the decision whether to hang or not, thus preparing it to support alternative boot devices. Some boot devices (namely nand and spi) do not hang on error. Instead, they return normally and SPL proceeds to boot the contents of the load address. This is considered a bug and is rectified by hanging on error for these devices as well. Signed-off-by: Nikita Kiryanov <nikita@compulab.co.il> Cc: Igor Grinberg <grinberg@compulab.co.il> Cc: Tom Rini <trini@konsulko.com> Cc: Simon Glass <sjg@chromium.org> Cc: Ian Campbell <ijc@hellion.org.uk> Cc: Hans De Goede <hdegoede@redhat.com> Cc: Albert Aribaud <albert.u.boot@aribaud.net> Cc: Jagan Teki <jteki@openedev.com> Reviewed-by: Tom Rini <trini@konsulko.com> Reviewed-by: Simon Glass <sjg@chromium.org>
91 lines
2.1 KiB
C
91 lines
2.1 KiB
C
/*
|
|
* Copyright (C) 2011 OMICRON electronics GmbH
|
|
*
|
|
* based on drivers/mtd/nand/nand_spl_load.c
|
|
*
|
|
* Copyright (C) 2011
|
|
* Heiko Schocher, DENX Software Engineering, hs@denx.de.
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <spi.h>
|
|
#include <spi_flash.h>
|
|
#include <errno.h>
|
|
#include <spl.h>
|
|
|
|
#ifdef CONFIG_SPL_OS_BOOT
|
|
/*
|
|
* Load the kernel, check for a valid header we can parse, and if found load
|
|
* the kernel and then device tree.
|
|
*/
|
|
static int spi_load_image_os(struct spi_flash *flash,
|
|
struct image_header *header)
|
|
{
|
|
/* Read for a header, parse or error out. */
|
|
spi_flash_read(flash, CONFIG_SYS_SPI_KERNEL_OFFS, 0x40,
|
|
(void *)header);
|
|
|
|
if (image_get_magic(header) != IH_MAGIC)
|
|
return -1;
|
|
|
|
spl_parse_image_header(header);
|
|
|
|
spi_flash_read(flash, CONFIG_SYS_SPI_KERNEL_OFFS,
|
|
spl_image.size, (void *)spl_image.load_addr);
|
|
|
|
/* Read device tree. */
|
|
spi_flash_read(flash, CONFIG_SYS_SPI_ARGS_OFFS,
|
|
CONFIG_SYS_SPI_ARGS_SIZE,
|
|
(void *)CONFIG_SYS_SPL_ARGS_ADDR);
|
|
|
|
return 0;
|
|
}
|
|
#endif
|
|
|
|
/*
|
|
* The main entry for SPI booting. It's necessary that SDRAM is already
|
|
* configured and available since this code loads the main U-Boot image
|
|
* from SPI into SDRAM and starts it from there.
|
|
*/
|
|
int spl_spi_load_image(void)
|
|
{
|
|
int err = 0;
|
|
struct spi_flash *flash;
|
|
struct image_header *header;
|
|
|
|
/*
|
|
* Load U-Boot image from SPI flash into RAM
|
|
*/
|
|
|
|
flash = spi_flash_probe(CONFIG_SF_DEFAULT_BUS,
|
|
CONFIG_SF_DEFAULT_CS,
|
|
CONFIG_SF_DEFAULT_SPEED,
|
|
CONFIG_SF_DEFAULT_MODE);
|
|
if (!flash) {
|
|
puts("SPI probe failed.\n");
|
|
return -ENODEV;
|
|
}
|
|
|
|
/* use CONFIG_SYS_TEXT_BASE as temporary storage area */
|
|
header = (struct image_header *)(CONFIG_SYS_TEXT_BASE);
|
|
|
|
#ifdef CONFIG_SPL_OS_BOOT
|
|
if (spl_start_uboot() || spi_load_image_os(flash, header))
|
|
#endif
|
|
{
|
|
/* Load u-boot, mkimage header is 64 bytes. */
|
|
err = spi_flash_read(flash, CONFIG_SYS_SPI_U_BOOT_OFFS, 0x40,
|
|
(void *)header);
|
|
if (err)
|
|
return err;
|
|
|
|
spl_parse_image_header(header);
|
|
err = spi_flash_read(flash, CONFIG_SYS_SPI_U_BOOT_OFFS,
|
|
spl_image.size, (void *)spl_image.load_addr);
|
|
}
|
|
|
|
return err;
|
|
}
|