mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-26 21:03:44 +09:00
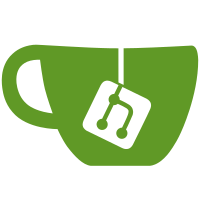
- Add new MESON_EE driver support for GXBB & AXG - Add support for Libretech-CC v2, Wetek Core2, Beelink GT-King/Pro boards - add driver for TDO tl070wsh30 panel driver - meson: isolate loading of socinfo - Add soc_rev to environment - Enable G12A support for saradc - Add correct mmcdev on VIM3(L) & Odroid-N2(C4) - Read MAC from fuses for VIM3 & VIM3L boards -----BEGIN PGP SIGNATURE----- iQIzBAABCgAdFiEEPVPGJshWBf4d9CyLd9zb2sjISdEFAl/9rV0ACgkQd9zb2sjI SdFE0xAAtXzW98pKmC4C3a1FWcGSuBZK2gzUgKc8oTqBWodMTVLSmDGNbIpaES/+ UySXSR0KJ5Qmu3jEZN2NYUKuABzylmIyT+5/sj1yN0zgxU2NB2//18uELNVKC4b+ wtLna+SSebl8ofGuVzfYYCfbBS0S2Y+n9g2+WVu3s+D03WG5wrqEZy5RqKRf4OMZ MoQiDoLFms1GS6iLoidg/RMNme6efODAOTOVklHre2zeN3rjGei2URHdVtIeXcdG G3Wz9GVwR96UWTGY6JuXEGVpI459vHc4AcswnVcKNnILS/50tpSA9Gc0e0lXaDwT 29DPehnoBkdbtIQ4szrAthGBITWhhgp2ksHaY5jyg38mVGF1/uu76kPUlCLxY5fL //3j/t+qaA3iwJq36tvZSKbprjA8sINN8Hsmi9+2Bzyv90sLfxY/x/DpeTVfWUQf UireG3iCYBkP5Mxr1kO+eJUoPAEe8BDJ+3AyVCjlMvKdomGFGCY0C/2LY54G8WS+ el2Sj8XkTgtZQA/HXuXg1y2Cw54FWN6SXMVFXqZW5bu/w2GmeSOMEkFs3aFieCB7 5UP+PiA3aM9Z6oXJReNnwc7iy+de8peZ/gpNCqWxr/jxg/C5d+Lg3kgbr961MyhU e97xab9p3f9raVOrYCAP7jVqAfTS39ZtAzSZlSJcK3Pls9wVxJI= =iIWq -----END PGP SIGNATURE----- Merge tag 'u-boot-amlogic-20210112' of https://gitlab.denx.de/u-boot/custodians/u-boot-amlogic - sync amlogic GX & AXG DT to Linux 5.10 - Add new MESON_EE driver support for GXBB & AXG - Add support for Libretech-CC v2, Wetek Core2, Beelink GT-King/Pro boards - add driver for TDO tl070wsh30 panel driver - meson: isolate loading of socinfo - Add soc_rev to environment - Enable G12A support for saradc - Add correct mmcdev on VIM3(L) & Odroid-N2(C4) - Read MAC from fuses for VIM3 & VIM3L boards
84 lines
1.6 KiB
C
84 lines
1.6 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
|
|
#include <common.h>
|
|
#include <log.h>
|
|
#include <asm/io.h>
|
|
#include <clk-uclass.h>
|
|
#include <dm.h>
|
|
#include <regmap.h>
|
|
#include <syscon.h>
|
|
#include <dt-bindings/clock/g12a-aoclkc.h>
|
|
|
|
#include "clk_meson.h"
|
|
|
|
struct meson_clk {
|
|
struct regmap *map;
|
|
};
|
|
|
|
#define AO_CLK_GATE0 0x4c
|
|
#define AO_SAR_CLK 0x90
|
|
|
|
static struct meson_gate gates[] = {
|
|
MESON_GATE(CLKID_AO_SAR_ADC, AO_CLK_GATE0, 8),
|
|
MESON_GATE(CLKID_AO_SAR_ADC_CLK, AO_SAR_CLK, 8),
|
|
};
|
|
|
|
static int meson_set_gate(struct clk *clk, bool on)
|
|
{
|
|
struct meson_clk *priv = dev_get_priv(clk->dev);
|
|
struct meson_gate *gate;
|
|
|
|
if (clk->id >= ARRAY_SIZE(gates))
|
|
return -ENOENT;
|
|
|
|
gate = &gates[clk->id];
|
|
|
|
if (gate->reg == 0)
|
|
return 0;
|
|
|
|
regmap_update_bits(priv->map, gate->reg,
|
|
BIT(gate->bit), on ? BIT(gate->bit) : 0);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int meson_clk_enable(struct clk *clk)
|
|
{
|
|
return meson_set_gate(clk, true);
|
|
}
|
|
|
|
static int meson_clk_disable(struct clk *clk)
|
|
{
|
|
return meson_set_gate(clk, false);
|
|
}
|
|
|
|
static int meson_clk_probe(struct udevice *dev)
|
|
{
|
|
struct meson_clk *priv = dev_get_priv(dev);
|
|
|
|
priv->map = syscon_node_to_regmap(dev_ofnode(dev_get_parent(dev)));
|
|
if (IS_ERR(priv->map))
|
|
return PTR_ERR(priv->map);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static struct clk_ops meson_clk_ops = {
|
|
.disable = meson_clk_disable,
|
|
.enable = meson_clk_enable,
|
|
};
|
|
|
|
static const struct udevice_id meson_clk_ids[] = {
|
|
{ .compatible = "amlogic,meson-g12a-aoclkc" },
|
|
{ }
|
|
};
|
|
|
|
U_BOOT_DRIVER(meson_clk_axg) = {
|
|
.name = "meson_clk_g12a_ao",
|
|
.id = UCLASS_CLK,
|
|
.of_match = meson_clk_ids,
|
|
.priv_auto = sizeof(struct meson_clk),
|
|
.ops = &meson_clk_ops,
|
|
.probe = meson_clk_probe,
|
|
};
|