mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-21 18:39:43 +09:00
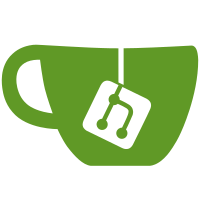
The gd will be cleared at first so we don't need to set arch.tbl to 0. In addition, the checks later against lastinc also work fine with an initial value of 0 here. This also brings us in line with sunxi code for example. Signed-off-by: Tom Rini <trini@ti.com> Reviewed-by: Simon Glass <sjg@chromium.org>
104 lines
2.3 KiB
C
104 lines
2.3 KiB
C
/*
|
|
* (C) Copyright 2008
|
|
* Texas Instruments
|
|
*
|
|
* Richard Woodruff <r-woodruff2@ti.com>
|
|
* Syed Moahmmed Khasim <khasim@ti.com>
|
|
*
|
|
* (C) Copyright 2002
|
|
* Sysgo Real-Time Solutions, GmbH <www.elinos.com>
|
|
* Marius Groeger <mgroeger@sysgo.de>
|
|
* Alex Zuepke <azu@sysgo.de>
|
|
*
|
|
* (C) Copyright 2002
|
|
* Gary Jennejohn, DENX Software Engineering, <garyj@denx.de>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <asm/io.h>
|
|
#include <asm/arch/cpu.h>
|
|
#include <asm/arch/clock.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
static struct gptimer *timer_base = (struct gptimer *)CONFIG_SYS_TIMERBASE;
|
|
|
|
/*
|
|
* Nothing really to do with interrupts, just starts up a counter.
|
|
*/
|
|
|
|
#define TIMER_CLOCK (V_SCLK / (2 << CONFIG_SYS_PTV))
|
|
#define TIMER_OVERFLOW_VAL 0xffffffff
|
|
#define TIMER_LOAD_VAL 0
|
|
|
|
int timer_init(void)
|
|
{
|
|
/* start the counter ticking up, reload value on overflow */
|
|
writel(TIMER_LOAD_VAL, &timer_base->tldr);
|
|
/* enable timer */
|
|
writel((CONFIG_SYS_PTV << 2) | TCLR_PRE | TCLR_AR | TCLR_ST,
|
|
&timer_base->tclr);
|
|
|
|
return 0;
|
|
}
|
|
|
|
/*
|
|
* timer without interrupts
|
|
*/
|
|
ulong get_timer(ulong base)
|
|
{
|
|
return get_timer_masked() - base;
|
|
}
|
|
|
|
/* delay x useconds */
|
|
void __udelay(unsigned long usec)
|
|
{
|
|
long tmo = usec * (TIMER_CLOCK / 1000) / 1000;
|
|
unsigned long now, last = readl(&timer_base->tcrr);
|
|
|
|
while (tmo > 0) {
|
|
now = readl(&timer_base->tcrr);
|
|
if (last > now) /* count up timer overflow */
|
|
tmo -= TIMER_OVERFLOW_VAL - last + now + 1;
|
|
else
|
|
tmo -= now - last;
|
|
last = now;
|
|
}
|
|
}
|
|
|
|
ulong get_timer_masked(void)
|
|
{
|
|
/* current tick value */
|
|
ulong now = readl(&timer_base->tcrr) / (TIMER_CLOCK / CONFIG_SYS_HZ);
|
|
|
|
if (now >= gd->arch.lastinc) { /* normal mode (non roll) */
|
|
/* move stamp fordward with absoulte diff ticks */
|
|
gd->arch.tbl += (now - gd->arch.lastinc);
|
|
} else { /* we have rollover of incrementer */
|
|
gd->arch.tbl += ((TIMER_LOAD_VAL / (TIMER_CLOCK /
|
|
CONFIG_SYS_HZ)) - gd->arch.lastinc) + now;
|
|
}
|
|
gd->arch.lastinc = now;
|
|
return gd->arch.tbl;
|
|
}
|
|
|
|
/*
|
|
* This function is derived from PowerPC code (read timebase as long long).
|
|
* On ARM it just returns the timer value.
|
|
*/
|
|
unsigned long long get_ticks(void)
|
|
{
|
|
return get_timer(0);
|
|
}
|
|
|
|
/*
|
|
* This function is derived from PowerPC code (timebase clock frequency).
|
|
* On ARM it returns the number of timer ticks per second.
|
|
*/
|
|
ulong get_tbclk(void)
|
|
{
|
|
return CONFIG_SYS_HZ;
|
|
}
|