mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-11 21:56:21 +09:00
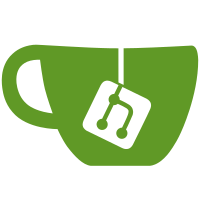
The Xtensa processor architecture is a configurable, extensible, and synthesizable 32-bit RISC processor core provided by Tensilica, inc. This is the second part of the basic architecture port, adding the 'arch/xtensa' directory and a readme file. Signed-off-by: Chris Zankel <chris@zankel.net> Signed-off-by: Max Filippov <jcmvbkbc@gmail.com> Reviewed-by: Simon Glass <sjg@chromium.org> Reviewed-by: Tom Rini <trini@konsulko.com>
56 lines
919 B
C
56 lines
919 B
C
/*
|
|
* Copyright (C) 2016 Cadence Design Systems Inc.
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#ifndef _XTENSA_ATOMIC_H
|
|
#define _XTENSA_ATOMIC_H
|
|
|
|
#include <asm/system.h>
|
|
|
|
typedef struct { volatile int counter; } atomic_t;
|
|
|
|
#define ATOMIC_INIT(i) { (i) }
|
|
|
|
#define atomic_read(v) ((v)->counter)
|
|
#define atomic_set(v, i) ((v)->counter = (i))
|
|
|
|
static inline void atomic_add(int i, atomic_t *v)
|
|
{
|
|
unsigned long flags;
|
|
|
|
local_irq_save(flags);
|
|
v->counter += i;
|
|
local_irq_restore(flags);
|
|
}
|
|
|
|
static inline void atomic_sub(int i, atomic_t *v)
|
|
{
|
|
unsigned long flags;
|
|
|
|
local_irq_save(flags);
|
|
v->counter -= i;
|
|
local_irq_restore(flags);
|
|
}
|
|
|
|
static inline void atomic_inc(atomic_t *v)
|
|
{
|
|
unsigned long flags;
|
|
|
|
local_irq_save(flags);
|
|
++v->counter;
|
|
local_irq_restore(flags);
|
|
}
|
|
|
|
static inline void atomic_dec(atomic_t *v)
|
|
{
|
|
unsigned long flags;
|
|
|
|
local_irq_save(flags);
|
|
--v->counter;
|
|
local_irq_restore(flags);
|
|
}
|
|
|
|
#endif
|