mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-06 03:06:16 +09:00
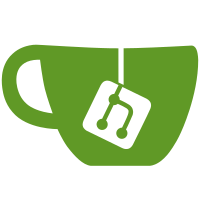
All callers of malloc should already do error checking, and may even be able to continue without the alloc succeeding. Moreover, common/malloc_simple.c is the only user of .rodata.str1.1 in common/built-in.o when building the SPL, triggering this gcc bug: https://gcc.gnu.org/bugzilla/show_bug.cgi?id=54303 Causing .rodata to grow with e.g. 0xc21 bytes, nullifying all benefits of using malloc_simple in the first place. Signed-off-by: Hans de Goede <hdegoede@redhat.com> Acked-by: Simon Glass <sjg@chromium.org>
40 lines
675 B
C
40 lines
675 B
C
/*
|
|
* Simple malloc implementation
|
|
*
|
|
* Copyright (c) 2014 Google, Inc
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <malloc.h>
|
|
#include <asm/io.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
void *malloc_simple(size_t bytes)
|
|
{
|
|
ulong new_ptr;
|
|
void *ptr;
|
|
|
|
new_ptr = gd->malloc_ptr + bytes;
|
|
if (new_ptr > gd->malloc_limit)
|
|
return NULL;
|
|
ptr = map_sysmem(gd->malloc_base + gd->malloc_ptr, bytes);
|
|
gd->malloc_ptr = ALIGN(new_ptr, sizeof(new_ptr));
|
|
return ptr;
|
|
}
|
|
|
|
#ifdef CONFIG_SYS_MALLOC_SIMPLE
|
|
void *calloc(size_t nmemb, size_t elem_size)
|
|
{
|
|
size_t size = nmemb * elem_size;
|
|
void *ptr;
|
|
|
|
ptr = malloc(size);
|
|
memset(ptr, '\0', size);
|
|
|
|
return ptr;
|
|
}
|
|
#endif
|