mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
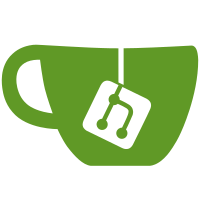
reset cause is a generic functionality based on the soc cru registers in rockchip. This can be used for printing the cause of reset in cpuinfo or some other place where reset cause is needed. Other than cpuinfo, reset cause can also be using during bootcount for checking the specific reset cause and glow the led based on the reset cause. So, let's separate the reset cause code from cpuinfo, and add a check to build it for rk3399, rk3288 since these two soc are supporting reset cause as of now. Tested-by: Suniel Mahesh <sunil@amarulasolutions.com> Signed-off-by: Jagan Teki <jagan@amarulasolutions.com> Reviewed-by: Kever Yang <kever.yang@rock-chips.com>
66 lines
1.3 KiB
C
66 lines
1.3 KiB
C
// SPDX-License-Identifier: (GPL-2.0+ OR MIT)
|
|
/*
|
|
* (C) Copyright 2019 Amarula Solutions(India)
|
|
* Author: Jagan Teki <jagan@amarulasolutions.com>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <env.h>
|
|
#include <init.h>
|
|
#include <asm/io.h>
|
|
#include <asm/arch-rockchip/clock.h>
|
|
#include <asm/arch-rockchip/cru.h>
|
|
#include <asm/arch-rockchip/hardware.h>
|
|
#include <linux/err.h>
|
|
|
|
char *get_reset_cause(void)
|
|
{
|
|
struct rockchip_cru *cru = rockchip_get_cru();
|
|
char *cause = NULL;
|
|
|
|
if (IS_ERR(cru))
|
|
return cause;
|
|
|
|
switch (cru->glb_rst_st) {
|
|
case GLB_POR_RST:
|
|
cause = "POR";
|
|
break;
|
|
case FST_GLB_RST_ST:
|
|
case SND_GLB_RST_ST:
|
|
cause = "RST";
|
|
break;
|
|
case FST_GLB_TSADC_RST_ST:
|
|
case SND_GLB_TSADC_RST_ST:
|
|
cause = "THERMAL";
|
|
break;
|
|
case FST_GLB_WDT_RST_ST:
|
|
case SND_GLB_WDT_RST_ST:
|
|
cause = "WDOG";
|
|
break;
|
|
default:
|
|
cause = "unknown reset";
|
|
}
|
|
|
|
return cause;
|
|
}
|
|
|
|
#if CONFIG_IS_ENABLED(DISPLAY_CPUINFO)
|
|
int print_cpuinfo(void)
|
|
{
|
|
char *cause = get_reset_cause();
|
|
|
|
printf("SoC: Rockchip %s\n", CONFIG_SYS_SOC);
|
|
printf("Reset cause: %s\n", cause);
|
|
|
|
/**
|
|
* reset_reason env is used by rk3288, due to special use case
|
|
* to figure it the boot behavior. so keep this as it is.
|
|
*/
|
|
env_set("reset_reason", cause);
|
|
|
|
/* TODO print operating temparature and clock */
|
|
|
|
return 0;
|
|
}
|
|
#endif
|