mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-10-01 17:10:42 +09:00
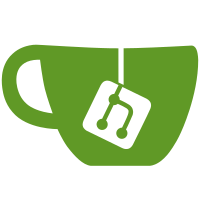
According to the I2C specification device address 0 is the "general call address", ie a broadcast address. The I2C specification states that the format of a general call uses at least 2 bytes, which U-Boot's probing routine does not adhere to. Not probing device address 0 will prevent possible issues with devices that accept general calls. Additionally, this change shouldn't reduce POST coverage since each I2C device should still be accessed via its own, unique address. Signed-off-by: Peter Tyser <ptyser@xes-inc.com> Acked-by: Heiko Schocher <hs@denx.de> Acked-by: Wolfgang Denk <wd@denx.de> Signed-off-by: Kumar Gala <galak@kernel.crashing.org>
89 lines
2.2 KiB
C
89 lines
2.2 KiB
C
/*
|
|
* (C) Copyright 2002
|
|
* Wolfgang Denk, DENX Software Engineering, wd@denx.de.
|
|
*
|
|
* See file CREDITS for list of people who contributed to this
|
|
* project.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston,
|
|
* MA 02111-1307 USA
|
|
*/
|
|
|
|
/*
|
|
* I2C test
|
|
*
|
|
* For verifying the I2C bus, a full I2C bus scanning is performed.
|
|
*
|
|
* #ifdef I2C_ADDR_LIST
|
|
* The test is considered as passed if all the devices and
|
|
* only the devices in the list are found.
|
|
* #else [ ! I2C_ADDR_LIST ]
|
|
* The test is considered as passed if any I2C device is found.
|
|
* #endif
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <post.h>
|
|
#include <i2c.h>
|
|
|
|
#if CONFIG_POST & CONFIG_SYS_POST_I2C
|
|
|
|
int i2c_post_test (int flags)
|
|
{
|
|
unsigned int i;
|
|
#ifndef I2C_ADDR_LIST
|
|
/* Start at address 1, address 0 is the general call address */
|
|
for (i = 1; i < 128; i++)
|
|
if (i2c_probe (i) == 0)
|
|
return 0;
|
|
|
|
/* No devices found */
|
|
return -1;
|
|
#else
|
|
unsigned int ret = 0;
|
|
int j;
|
|
const unsigned char i2c_addr_list[] = I2C_ADDR_LIST;
|
|
|
|
/* Start at address 1, address 0 is the general call address */
|
|
for (i = 1; i < 128; i++) {
|
|
if (i2c_probe(i) != 0)
|
|
continue;
|
|
|
|
for (j = 0; j < sizeof(i2c_addr_list); ++j) {
|
|
if (i == i2c_addr_list[j]) {
|
|
i2c_addr_list[j] = 0xff;
|
|
break;
|
|
}
|
|
}
|
|
|
|
if (j == sizeof(i2c_addr_list)) {
|
|
ret = -1;
|
|
post_log("I2C: addr %02x not expected\n", i);
|
|
}
|
|
}
|
|
|
|
for (i = 0; i < sizeof(i2c_addr_list); ++i) {
|
|
if (i2c_addr_list[i] == 0xff)
|
|
continue;
|
|
post_log("I2C: addr %02x did not respond\n", i2c_addr_list[i]);
|
|
ret = -1;
|
|
}
|
|
|
|
return ret;
|
|
#endif
|
|
}
|
|
|
|
#endif /* CONFIG_POST & CONFIG_SYS_POST_I2C */
|