mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 15:26:03 +09:00
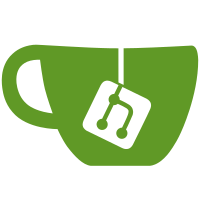
This function is actually intended to read a string rather than a property. All of its current callers use it that way. Also there is no way to return the length of the property from this function. Rename it to better indicate its purpose, using ofnode_read as the prefix since this matches most other functions. Also add some tests which are missing for these functions. Signed-off-by: Simon Glass <sjg@chromium.org>
70 lines
1.5 KiB
C
70 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* (C) Copyright 2014 - 2019 Xilinx, Inc.
|
|
* Michal Simek <michal.simek@xilinx.com>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <asm/sections.h>
|
|
#include <dm/uclass.h>
|
|
#include <i2c.h>
|
|
|
|
int zynq_board_read_rom_ethaddr(unsigned char *ethaddr)
|
|
{
|
|
int ret = -EINVAL;
|
|
|
|
#if defined(CONFIG_ZYNQ_GEM_I2C_MAC_OFFSET)
|
|
struct udevice *dev;
|
|
ofnode eeprom;
|
|
|
|
eeprom = ofnode_get_chosen_node("xlnx,eeprom");
|
|
if (!ofnode_valid(eeprom))
|
|
return -ENODEV;
|
|
|
|
debug("%s: Path to EEPROM %s\n", __func__,
|
|
ofnode_read_chosen_string("xlnx,eeprom"));
|
|
|
|
ret = uclass_get_device_by_ofnode(UCLASS_I2C_EEPROM, eeprom, &dev);
|
|
if (ret)
|
|
return ret;
|
|
|
|
ret = dm_i2c_read(dev, CONFIG_ZYNQ_GEM_I2C_MAC_OFFSET, ethaddr, 6);
|
|
if (ret)
|
|
debug("%s: I2C EEPROM MAC address read failed\n", __func__);
|
|
else
|
|
debug("%s: I2C EEPROM MAC %pM\n", __func__, ethaddr);
|
|
#endif
|
|
|
|
return ret;
|
|
}
|
|
|
|
#if defined(CONFIG_OF_BOARD) || defined(CONFIG_OF_SEPARATE)
|
|
void *board_fdt_blob_setup(void)
|
|
{
|
|
static void *fdt_blob = (void *)CONFIG_XILINX_OF_BOARD_DTB_ADDR;
|
|
|
|
if (fdt_magic(fdt_blob) == FDT_MAGIC)
|
|
return fdt_blob;
|
|
|
|
debug("DTB is not passed via %p\n", fdt_blob);
|
|
|
|
#ifdef CONFIG_SPL_BUILD
|
|
/* FDT is at end of BSS unless it is in a different memory region */
|
|
if (IS_ENABLED(CONFIG_SPL_SEPARATE_BSS))
|
|
fdt_blob = (ulong *)&_image_binary_end;
|
|
else
|
|
fdt_blob = (ulong *)&__bss_end;
|
|
#else
|
|
/* FDT is at end of image */
|
|
fdt_blob = (ulong *)&_end;
|
|
#endif
|
|
|
|
if (fdt_magic(fdt_blob) == FDT_MAGIC)
|
|
return fdt_blob;
|
|
|
|
debug("DTB is also not passed via %p\n", fdt_blob);
|
|
|
|
return NULL;
|
|
}
|
|
#endif
|