mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-14 07:06:19 +09:00
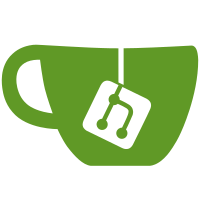
T104xrdb has several sleep management signals that are used for deep sleep. They are enabled by OS to enter deep sleep and should be disabled by u-boot when cores wake up. Signed-off-by: Tang Yuantian <Yuantian.Tang@freescale.com> Reviewed-by: York Sun <yorksun@freescale.com>
119 lines
2.3 KiB
C
119 lines
2.3 KiB
C
/*
|
|
* Copyright 2013 Freescale Semiconductor, Inc.
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <command.h>
|
|
#include <netdev.h>
|
|
#include <linux/compiler.h>
|
|
#include <asm/mmu.h>
|
|
#include <asm/processor.h>
|
|
#include <asm/cache.h>
|
|
#include <asm/immap_85xx.h>
|
|
#include <asm/fsl_law.h>
|
|
#include <asm/fsl_serdes.h>
|
|
#include <asm/fsl_portals.h>
|
|
#include <asm/fsl_liodn.h>
|
|
#include <fm_eth.h>
|
|
#include <asm/mpc85xx_gpio.h>
|
|
|
|
#include "t104xrdb.h"
|
|
#include "cpld.h"
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
int checkboard(void)
|
|
{
|
|
struct cpu_type *cpu = gd->arch.cpu;
|
|
u8 sw;
|
|
|
|
printf("Board: %sRDB\n", cpu->name);
|
|
printf("Board rev: 0x%02x CPLD ver: 0x%02x, ",
|
|
CPLD_READ(hw_ver), CPLD_READ(sw_ver));
|
|
|
|
sw = CPLD_READ(flash_ctl_status);
|
|
sw = ((sw & CPLD_LBMAP_MASK) >> CPLD_LBMAP_SHIFT);
|
|
|
|
if (sw <= 7)
|
|
printf("vBank: %d\n", sw);
|
|
else
|
|
printf("Unsupported Bank=%x\n", sw);
|
|
|
|
return 0;
|
|
}
|
|
|
|
int board_early_init_r(void)
|
|
{
|
|
#ifdef CONFIG_SYS_FLASH_BASE
|
|
const unsigned int flashbase = CONFIG_SYS_FLASH_BASE;
|
|
const u8 flash_esel = find_tlb_idx((void *)flashbase, 1);
|
|
|
|
/*
|
|
* Remap Boot flash region to caching-inhibited
|
|
* so that flash can be erased properly.
|
|
*/
|
|
|
|
/* Flush d-cache and invalidate i-cache of any FLASH data */
|
|
flush_dcache();
|
|
invalidate_icache();
|
|
|
|
/* invalidate existing TLB entry for flash */
|
|
disable_tlb(flash_esel);
|
|
|
|
set_tlb(1, flashbase, CONFIG_SYS_FLASH_BASE_PHYS,
|
|
MAS3_SX|MAS3_SW|MAS3_SR, MAS2_I|MAS2_G,
|
|
0, flash_esel, BOOKE_PAGESZ_256M, 1);
|
|
#endif
|
|
set_liodns();
|
|
#ifdef CONFIG_SYS_DPAA_QBMAN
|
|
setup_portals();
|
|
#endif
|
|
|
|
return 0;
|
|
}
|
|
|
|
int misc_init_r(void)
|
|
{
|
|
return 0;
|
|
}
|
|
|
|
void ft_board_setup(void *blob, bd_t *bd)
|
|
{
|
|
phys_addr_t base;
|
|
phys_size_t size;
|
|
|
|
ft_cpu_setup(blob, bd);
|
|
|
|
base = getenv_bootm_low();
|
|
size = getenv_bootm_size();
|
|
|
|
fdt_fixup_memory(blob, (u64)base, (u64)size);
|
|
|
|
#ifdef CONFIG_PCI
|
|
pci_of_setup(blob, bd);
|
|
#endif
|
|
|
|
fdt_fixup_liodn(blob);
|
|
|
|
#ifdef CONFIG_HAS_FSL_DR_USB
|
|
fdt_fixup_dr_usb(blob, bd);
|
|
#endif
|
|
|
|
#ifdef CONFIG_SYS_DPAA_FMAN
|
|
fdt_fixup_fman_ethernet(blob);
|
|
#endif
|
|
}
|
|
|
|
#ifdef CONFIG_DEEP_SLEEP
|
|
void board_mem_sleep_setup(void)
|
|
{
|
|
/* does not provide HW signals for power management */
|
|
CPLD_WRITE(misc_ctl_status, (CPLD_READ(misc_ctl_status) & ~0x40));
|
|
/* Disable MCKE isolation */
|
|
gpio_set_value(2, 0);
|
|
udelay(1);
|
|
}
|
|
#endif
|