mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-06 19:26:16 +09:00
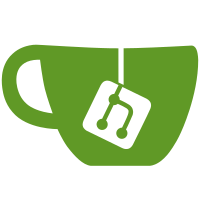
A hook is installed to configure PCI bus bridges as they encountered by u-boot. The hook extracts the secondary bus number from the bridge's config space and then recursively scans that bus. On Coreboot, the PCI bus address space has identity mapping with the physical address space, so declare it as such to ensure that the "pci_map_bar" function used by some PCI drivers is behaving properly. This fixes the EHCI PCI driver initialization on Stumpy. This was tested as follows: Ran the PCI command on Alex, saw devices on bus 0, the OXPCIe 952 on bus 1, and empty busses 2 through 5. This matches the bridges reported on bus 0 and the PCI configuration output from coreboot. Signed-off-by: Gabe Black <gabeblack@chromium.org> Signed-off-by: Vincent Palatin <vpalatin@chromium.org> Signed-off-by: Stefan Reinauer <reinauer@chromium.org> Signed-off-by: Simon Glass <sjg@chromium.org>
66 lines
1.9 KiB
C
66 lines
1.9 KiB
C
/*
|
|
* Copyright (c) 2011 The Chromium OS Authors.
|
|
* (C) Copyright 2008,2009
|
|
* Graeme Russ, <graeme.russ@gmail.com>
|
|
*
|
|
* (C) Copyright 2002
|
|
* Daniel Engström, Omicron Ceti AB, <daniel@omicron.se>
|
|
*
|
|
* See file CREDITS for list of people who contributed to this
|
|
* project.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston,
|
|
* MA 02111-1307 USA
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <pci.h>
|
|
#include <asm/pci.h>
|
|
|
|
static struct pci_controller coreboot_hose;
|
|
|
|
static void config_pci_bridge(struct pci_controller *hose, pci_dev_t dev,
|
|
struct pci_config_table *table)
|
|
{
|
|
u8 secondary;
|
|
hose->read_byte(hose, dev, PCI_SECONDARY_BUS, &secondary);
|
|
hose->last_busno = max(hose->last_busno, secondary);
|
|
pci_hose_scan_bus(hose, secondary);
|
|
}
|
|
|
|
static struct pci_config_table pci_coreboot_config_table[] = {
|
|
/* vendor, device, class, bus, dev, func */
|
|
{ PCI_ANY_ID, PCI_ANY_ID, PCI_CLASS_BRIDGE_PCI,
|
|
PCI_ANY_ID, PCI_ANY_ID, PCI_ANY_ID, &config_pci_bridge },
|
|
{}
|
|
};
|
|
|
|
void pci_init_board(void)
|
|
{
|
|
coreboot_hose.config_table = pci_coreboot_config_table;
|
|
coreboot_hose.first_busno = 0;
|
|
coreboot_hose.last_busno = 0;
|
|
|
|
pci_set_region(coreboot_hose.regions + 0, 0x0, 0x0, 0xffffffff,
|
|
PCI_REGION_MEM);
|
|
coreboot_hose.region_count = 1;
|
|
|
|
pci_setup_type1(&coreboot_hose);
|
|
|
|
pci_register_hose(&coreboot_hose);
|
|
|
|
pci_hose_scan(&coreboot_hose);
|
|
}
|