mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-06 03:06:16 +09:00
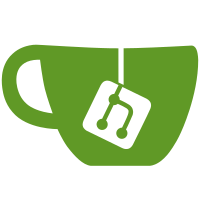
The Arria10 SPL is a complete mess of calls to functions which are called in the wrong context and it is surprise it works at all. This patch tries to clean that mess up by shuffling the function calls around and moving the calls into the correct context. Due to the delicate nature of the reordering, this is done in one huge patch. The following changes happen in this patch: - Security policy init and NIC301 happens first in board_init_f() - The clock init happens very early in board_init_f() in SPL only - arch_early_init_r() only registers the FPGA, just like on Gen5 - arch_early_init_r() is never called from any _f() function - Dedicated FPGA pins are inited in board_init_f() as on Gen5 Signed-off-by: Marek Vasut <marex@denx.de> Cc: Chin Liang See <chin.liang.see@intel.com> Cc: Dinh Nguyen <dinguyen@kernel.org> Cc: Ley Foon Tan <ley.foon.tan@intel.com>
89 lines
1.8 KiB
C
89 lines
1.8 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Altera SoCFPGA common board code
|
|
*
|
|
* Copyright (C) 2015 Marek Vasut <marex@denx.de>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <errno.h>
|
|
#include <fdtdec.h>
|
|
#include <asm/arch/reset_manager.h>
|
|
#include <asm/arch/clock_manager.h>
|
|
#include <asm/arch/misc.h>
|
|
#include <asm/io.h>
|
|
|
|
#include <usb.h>
|
|
#include <usb/dwc2_udc.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
void s_init(void) {
|
|
#ifndef CONFIG_ARM64
|
|
/*
|
|
* Preconfigure ACTLR and CPACR, make sure Write Full Line of Zeroes
|
|
* is disabled in ACTLR.
|
|
* This is optional on CycloneV / ArriaV.
|
|
* This is mandatory on Arria10, otherwise Linux refuses to boot.
|
|
*/
|
|
asm volatile(
|
|
"mcr p15, 0, %0, c1, c0, 1\n"
|
|
"mcr p15, 0, %0, c1, c0, 2\n"
|
|
"isb\n"
|
|
"dsb\n"
|
|
::"r"(0x0));
|
|
#endif
|
|
}
|
|
|
|
/*
|
|
* Miscellaneous platform dependent initialisations
|
|
*/
|
|
int board_init(void)
|
|
{
|
|
/* Address of boot parameters for ATAG (if ATAG is used) */
|
|
gd->bd->bi_boot_params = CONFIG_SYS_SDRAM_BASE + 0x100;
|
|
|
|
return 0;
|
|
}
|
|
|
|
int dram_init_banksize(void)
|
|
{
|
|
fdtdec_setup_memory_banksize();
|
|
|
|
return 0;
|
|
}
|
|
|
|
#ifdef CONFIG_USB_GADGET
|
|
struct dwc2_plat_otg_data socfpga_otg_data = {
|
|
.usb_gusbcfg = 0x1417,
|
|
};
|
|
|
|
int board_usb_init(int index, enum usb_init_type init)
|
|
{
|
|
int node[2], count;
|
|
fdt_addr_t addr;
|
|
|
|
count = fdtdec_find_aliases_for_id(gd->fdt_blob, "udc",
|
|
COMPAT_ALTERA_SOCFPGA_DWC2USB,
|
|
node, 2);
|
|
if (count <= 0) /* No controller found. */
|
|
return 0;
|
|
|
|
addr = fdtdec_get_addr(gd->fdt_blob, node[0], "reg");
|
|
if (addr == FDT_ADDR_T_NONE) {
|
|
printf("UDC Controller has no 'reg' property!\n");
|
|
return -EINVAL;
|
|
}
|
|
|
|
/* Patch the address from OF into the controller pdata. */
|
|
socfpga_otg_data.regs_otg = addr;
|
|
|
|
return dwc2_udc_probe(&socfpga_otg_data);
|
|
}
|
|
|
|
int g_dnl_board_usb_cable_connected(void)
|
|
{
|
|
return 1;
|
|
}
|
|
#endif
|