mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-09 04:36:16 +09:00
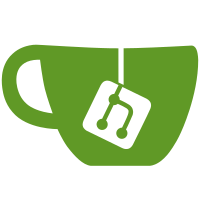
Define timer_get_boot_us() which returns the number of microseconds since boot. If undefined then we use get_timer() * 1000. We can fit this in a 32-bit register which keeps everyone happy on the efficiency side. It will wrap around after about an hour. If we are still looking at it after an hour then we had better not be timing the boot. Signed-off-by: Simon Glass <sjg@chromium.org>
67 lines
1.7 KiB
C
67 lines
1.7 KiB
C
/*
|
|
* (C) Copyright 2000-2009
|
|
* Wolfgang Denk, DENX Software Engineering, wd@denx.de.
|
|
*
|
|
* See file CREDITS for list of people who contributed to this
|
|
* project.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston,
|
|
* MA 02111-1307 USA
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <watchdog.h>
|
|
|
|
#ifndef CONFIG_WD_PERIOD
|
|
# define CONFIG_WD_PERIOD (10 * 1000 * 1000) /* 10 seconds default*/
|
|
#endif
|
|
|
|
/* ------------------------------------------------------------------------- */
|
|
|
|
void udelay(unsigned long usec)
|
|
{
|
|
ulong kv;
|
|
|
|
do {
|
|
WATCHDOG_RESET();
|
|
kv = usec > CONFIG_WD_PERIOD ? CONFIG_WD_PERIOD : usec;
|
|
__udelay (kv);
|
|
usec -= kv;
|
|
} while(usec);
|
|
}
|
|
|
|
void mdelay(unsigned long msec)
|
|
{
|
|
while (msec--)
|
|
udelay(1000);
|
|
}
|
|
|
|
ulong __timer_get_boot_us(void)
|
|
{
|
|
static ulong base_time;
|
|
|
|
/*
|
|
* We can't implement this properly. Return 0 on the first call and
|
|
* larger values after that.
|
|
*/
|
|
if (base_time)
|
|
return get_timer(base_time) * 1000;
|
|
base_time = get_timer(0);
|
|
return 0;
|
|
}
|
|
|
|
ulong timer_get_boot_us(void)
|
|
__attribute__((weak, alias("__timer_get_boot_us")));
|