mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
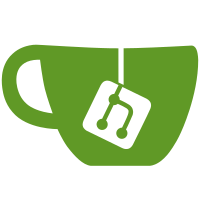
This patch adds generic support for the Samsung s3c2440 processor. Global s3c24x0 changes to struct members converting from upper case to lower case. Signed-off-by: Craig Nauman <cnauman@diagraph.com> Cc: kevin.morfitt@fearnside-systems.co.uk Signed-off-by: Minkyu Kang <mk7.kang@samsung.com>
194 lines
4.4 KiB
C
194 lines
4.4 KiB
C
/*
|
|
* (C) Copyright 2002
|
|
* Sysgo Real-Time Solutions, GmbH <www.elinos.com>
|
|
* Marius Groeger <mgroeger@sysgo.de>
|
|
*
|
|
* (C) Copyright 2002
|
|
* David Mueller, ELSOFT AG, <d.mueller@elsoft.ch>
|
|
*
|
|
* (C) Copyright 2005
|
|
* JinHua Luo, GuangDong Linux Center, <luo.jinhua@gd-linux.com>
|
|
*
|
|
* See file CREDITS for list of people who contributed to this
|
|
* project.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation; either version 2 of
|
|
* the License, or (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston,
|
|
* MA 02111-1307 USA
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <netdev.h>
|
|
#include <asm/arch/s3c24x0_cpu.h>
|
|
|
|
#if defined(CONFIG_CMD_NAND)
|
|
#include <linux/mtd/nand.h>
|
|
#endif
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
#define FCLK_SPEED 1
|
|
|
|
#if FCLK_SPEED==0 /* Fout = 203MHz, Fin = 12MHz for Audio */
|
|
#define M_MDIV 0xC3
|
|
#define M_PDIV 0x4
|
|
#define M_SDIV 0x1
|
|
#elif FCLK_SPEED==1 /* Fout = 202.8MHz */
|
|
#define M_MDIV 0x5c
|
|
#define M_PDIV 0x4
|
|
#define M_SDIV 0x0
|
|
#endif
|
|
|
|
#define USB_CLOCK 1
|
|
|
|
#if USB_CLOCK==0
|
|
#define U_M_MDIV 0xA1
|
|
#define U_M_PDIV 0x3
|
|
#define U_M_SDIV 0x1
|
|
#elif USB_CLOCK==1
|
|
#define U_M_MDIV 0x48
|
|
#define U_M_PDIV 0x3
|
|
#define U_M_SDIV 0x2
|
|
#endif
|
|
|
|
static inline void delay (unsigned long loops)
|
|
{
|
|
__asm__ volatile ("1:\n"
|
|
"subs %0, %1, #1\n"
|
|
"bne 1b":"=r" (loops):"0" (loops));
|
|
}
|
|
|
|
/*
|
|
* Miscellaneous platform dependent initialisations
|
|
*/
|
|
|
|
int board_init (void)
|
|
{
|
|
struct s3c24x0_clock_power * const clk_power =
|
|
s3c24x0_get_base_clock_power();
|
|
struct s3c24x0_gpio * const gpio = s3c24x0_get_base_gpio();
|
|
|
|
/* to reduce PLL lock time, adjust the LOCKTIME register */
|
|
clk_power->locktime = 0xFFFFFF;
|
|
|
|
/* configure MPLL */
|
|
clk_power->mpllcon = ((M_MDIV << 12) + (M_PDIV << 4) + M_SDIV);
|
|
|
|
/* some delay between MPLL and UPLL */
|
|
delay (4000);
|
|
|
|
/* configure UPLL */
|
|
clk_power->upllcon = ((U_M_MDIV << 12) + (U_M_PDIV << 4) + U_M_SDIV);
|
|
|
|
/* some delay between MPLL and UPLL */
|
|
delay (8000);
|
|
|
|
/* set up the I/O ports */
|
|
gpio->gpacon = 0x007FFFFF;
|
|
gpio->gpbcon = 0x00044556;
|
|
gpio->gpbup = 0x000007FF;
|
|
gpio->gpccon = 0xAAAAAAAA;
|
|
gpio->gpcup = 0x0000FFFF;
|
|
gpio->gpdcon = 0xAAAAAAAA;
|
|
gpio->gpdup = 0x0000FFFF;
|
|
gpio->gpecon = 0xAAAAAAAA;
|
|
gpio->gpeup = 0x0000FFFF;
|
|
gpio->gpfcon = 0x000055AA;
|
|
gpio->gpfup = 0x000000FF;
|
|
gpio->gpgcon = 0xFF95FF3A;
|
|
gpio->gpgup = 0x0000FFFF;
|
|
gpio->gphcon = 0x0016FAAA;
|
|
gpio->gphup = 0x000007FF;
|
|
|
|
gpio->extint0 = 0x22222222;
|
|
gpio->extint1 = 0x22222222;
|
|
gpio->extint2 = 0x22222222;
|
|
|
|
/* arch number of SMDK2410-Board */
|
|
gd->bd->bi_arch_number = MACH_TYPE_SMDK2410;
|
|
|
|
/* adress of boot parameters */
|
|
gd->bd->bi_boot_params = 0x30000100;
|
|
|
|
icache_enable();
|
|
dcache_enable();
|
|
|
|
return 0;
|
|
}
|
|
|
|
int dram_init (void)
|
|
{
|
|
gd->bd->bi_dram[0].start = PHYS_SDRAM_1;
|
|
gd->bd->bi_dram[0].size = PHYS_SDRAM_1_SIZE;
|
|
|
|
return 0;
|
|
}
|
|
|
|
#if defined(CONFIG_CMD_NAND)
|
|
extern ulong nand_probe(ulong physadr);
|
|
|
|
static inline void NF_Reset(void)
|
|
{
|
|
int i;
|
|
|
|
NF_SetCE(NFCE_LOW);
|
|
NF_Cmd(0xFF); /* reset command */
|
|
for(i = 0; i < 10; i++); /* tWB = 100ns. */
|
|
NF_WaitRB(); /* wait 200~500us; */
|
|
NF_SetCE(NFCE_HIGH);
|
|
}
|
|
|
|
static inline void NF_Init(void)
|
|
{
|
|
#if 1
|
|
#define TACLS 0
|
|
#define TWRPH0 3
|
|
#define TWRPH1 0
|
|
#else
|
|
#define TACLS 0
|
|
#define TWRPH0 4
|
|
#define TWRPH1 2
|
|
#endif
|
|
|
|
NF_Conf((1<<15)|(0<<14)|(0<<13)|(1<<12)|(1<<11)|(TACLS<<8)|(TWRPH0<<4)|(TWRPH1<<0));
|
|
/*nand->NFCONF = (1<<15)|(1<<14)|(1<<13)|(1<<12)|(1<<11)|(TACLS<<8)|(TWRPH0<<4)|(TWRPH1<<0); */
|
|
/* 1 1 1 1, 1 xxx, r xxx, r xxx */
|
|
/* En 512B 4step ECCR nFCE=H tACLS tWRPH0 tWRPH1 */
|
|
|
|
NF_Reset();
|
|
}
|
|
|
|
void nand_init(void)
|
|
{
|
|
struct s3c2410_nand * const nand = s3c2410_get_base_nand();
|
|
|
|
NF_Init();
|
|
#ifdef DEBUG
|
|
printf("NAND flash probing at 0x%.8lX\n", (ulong)nand);
|
|
#endif
|
|
printf ("%4lu MB\n", nand_probe((ulong)nand) >> 20);
|
|
}
|
|
#endif
|
|
|
|
#ifdef CONFIG_CMD_NET
|
|
int board_eth_init(bd_t *bis)
|
|
{
|
|
int rc = 0;
|
|
#ifdef CONFIG_CS8900
|
|
rc = cs8900_initialize(0, CONFIG_CS8900_BASE);
|
|
#endif
|
|
return rc;
|
|
}
|
|
#endif
|