mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
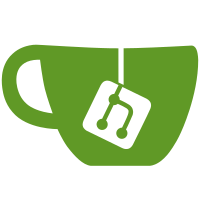
Some platforms don't have native code for dealing with their video hardware. In some cases they use a binary blob to set it up and perform required actions like setting the video mode. This approach is a hangover from the old PC days where a ROM was provided and executed during startup. Even now, these ROMs are supplied as a way to set up video. It avoids the code for every video chip needing to be provided in the boot loader. But it makes the video much less flexible - e.g. it is not possible to do anything else while the video init is happening (including waiting hundreds of milliseconds for display panels to start up). In any case, to deal with this sad state of affairs, provide an API for execution of x86 video ROMs, either natively or through emulation. Signed-off-by: Simon Glass <sjg@chromium.org>
105 lines
3.1 KiB
C
105 lines
3.1 KiB
C
/*
|
|
* (C) Copyright 1997-2002 ELTEC Elektronik AG
|
|
* Frank Gottschling <fgottschling@eltec.de>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
/*
|
|
* smiLynxEM.h
|
|
* Silicon Motion graphic interface for sm810/sm710/sm712 accelerator
|
|
*
|
|
*
|
|
* modification history
|
|
* --------------------
|
|
* 04-18-2002 Rewritten for U-Boot <fgottschling@eltec.de>.
|
|
*/
|
|
|
|
#ifndef _VIDEO_FB_H_
|
|
#define _VIDEO_FB_H_
|
|
|
|
#if defined(CONFIG_SYS_CONSOLE_FG_COL) && defined(CONFIG_SYS_CONSOLE_BG_COL)
|
|
#define CONSOLE_BG_COL CONFIG_SYS_CONSOLE_BG_COL
|
|
#define CONSOLE_FG_COL CONFIG_SYS_CONSOLE_FG_COL
|
|
#else
|
|
#define CONSOLE_BG_COL 0x00
|
|
#define CONSOLE_FG_COL 0xa0
|
|
#endif
|
|
|
|
/*
|
|
* Graphic Data Format (GDF) bits for VIDEO_DATA_FORMAT
|
|
*/
|
|
#define GDF__8BIT_INDEX 0
|
|
#define GDF_15BIT_555RGB 1
|
|
#define GDF_16BIT_565RGB 2
|
|
#define GDF_32BIT_X888RGB 3
|
|
#define GDF_24BIT_888RGB 4
|
|
#define GDF__8BIT_332RGB 5
|
|
|
|
/******************************************************************************/
|
|
/* Export Graphic Driver Control */
|
|
/******************************************************************************/
|
|
|
|
typedef struct graphic_device {
|
|
unsigned int isaBase;
|
|
unsigned int pciBase;
|
|
unsigned int dprBase;
|
|
unsigned int vprBase;
|
|
unsigned int cprBase;
|
|
unsigned int frameAdrs;
|
|
unsigned int memSize;
|
|
unsigned int mode;
|
|
unsigned int gdfIndex;
|
|
unsigned int gdfBytesPP;
|
|
unsigned int fg;
|
|
unsigned int bg;
|
|
unsigned int plnSizeX;
|
|
unsigned int plnSizeY;
|
|
unsigned int winSizeX;
|
|
unsigned int winSizeY;
|
|
char modeIdent[80];
|
|
} GraphicDevice;
|
|
|
|
|
|
/******************************************************************************/
|
|
/* Export Graphic Functions */
|
|
/******************************************************************************/
|
|
|
|
void *video_hw_init (void); /* returns GraphicDevice struct or NULL */
|
|
|
|
#ifdef VIDEO_HW_BITBLT
|
|
void video_hw_bitblt (
|
|
unsigned int bpp, /* bytes per pixel */
|
|
unsigned int src_x, /* source pos x */
|
|
unsigned int src_y, /* source pos y */
|
|
unsigned int dst_x, /* dest pos x */
|
|
unsigned int dst_y, /* dest pos y */
|
|
unsigned int dim_x, /* frame width */
|
|
unsigned int dim_y /* frame height */
|
|
);
|
|
#endif
|
|
|
|
#ifdef VIDEO_HW_RECTFILL
|
|
void video_hw_rectfill (
|
|
unsigned int bpp, /* bytes per pixel */
|
|
unsigned int dst_x, /* dest pos x */
|
|
unsigned int dst_y, /* dest pos y */
|
|
unsigned int dim_x, /* frame width */
|
|
unsigned int dim_y, /* frame height */
|
|
unsigned int color /* fill color */
|
|
);
|
|
#endif
|
|
|
|
void video_set_lut (
|
|
unsigned int index, /* color number */
|
|
unsigned char r, /* red */
|
|
unsigned char g, /* green */
|
|
unsigned char b /* blue */
|
|
);
|
|
#ifdef CONFIG_VIDEO_HW_CURSOR
|
|
void video_set_hw_cursor(int x, int y); /* x y in pixel */
|
|
void video_init_hw_cursor(int font_width, int font_height);
|
|
#endif
|
|
|
|
#endif /*_VIDEO_FB_H_ */
|