mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
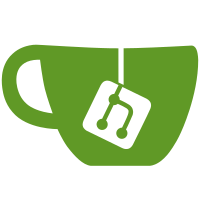
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
91 lines
2.5 KiB
C
91 lines
2.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* (C) Copyright 2002 ELTEC Elektronik AG
|
|
* Frank Gottschling <fgottschling@eltec.de>
|
|
*/
|
|
|
|
/* i8042.h - Intel 8042 keyboard driver header */
|
|
|
|
#ifndef _I8042_H_
|
|
#define _I8042_H_
|
|
|
|
/* defines */
|
|
|
|
#define I8042_DATA_REG 0x60 /* keyboard i/o buffer */
|
|
#define I8042_STS_REG 0x64 /* keyboard status read */
|
|
#define I8042_CMD_REG 0x64 /* keyboard ctrl write */
|
|
|
|
/* Status register bit defines */
|
|
#define STATUS_OBF (1 << 0)
|
|
#define STATUS_IBF (1 << 1)
|
|
|
|
/* Configuration byte bit defines */
|
|
#define CONFIG_KIRQ_EN (1 << 0)
|
|
#define CONFIG_MIRQ_EN (1 << 1)
|
|
#define CONFIG_SET_BIST (1 << 2)
|
|
#define CONFIG_KCLK_DIS (1 << 4)
|
|
#define CONFIG_MCLK_DIS (1 << 5)
|
|
#define CONFIG_AT_TRANS (1 << 6)
|
|
|
|
/* i8042 commands */
|
|
#define CMD_RD_CONFIG 0x20 /* read configuration byte */
|
|
#define CMD_WR_CONFIG 0x60 /* write configuration byte */
|
|
#define CMD_SELF_TEST 0xaa /* controller self-test */
|
|
#define CMD_KBD_DIS 0xad /* keyboard disable */
|
|
#define CMD_KBD_EN 0xae /* keyboard enable */
|
|
#define CMD_SET_KBD_LED 0xed /* set keyboard led */
|
|
#define CMD_DRAIN_OUTPUT 0xf4 /* drain output buffer */
|
|
#define CMD_RESET_KBD 0xff /* reset keyboard */
|
|
|
|
/* i8042 command result */
|
|
#define KBC_TEST_OK 0x55
|
|
#define KBD_ACK 0xfa
|
|
#define KBD_POR 0xaa
|
|
|
|
/* keyboard scan codes */
|
|
|
|
#define KBD_US 0 /* default US layout */
|
|
#define KBD_GER 1 /* german layout */
|
|
|
|
#define KBD_TIMEOUT 1000 /* 1 sec */
|
|
#define KBD_RESET_TRIES 3
|
|
|
|
#define AS 0 /* normal character index */
|
|
#define SH 1 /* shift index */
|
|
#define CN 2 /* control index */
|
|
#define NM 3 /* numeric lock index */
|
|
#define AK 4 /* right alt key */
|
|
#define CP 5 /* capslock index */
|
|
#define ST 6 /* stop output index */
|
|
#define EX 7 /* extended code index */
|
|
#define ES 8 /* escape and extended code index */
|
|
|
|
#define NORMAL 0x0000 /* normal key */
|
|
#define STP 0x0001 /* scroll lock stop output*/
|
|
#define NUM 0x0002 /* numeric lock */
|
|
#define CAPS 0x0004 /* capslock */
|
|
#define SHIFT 0x0008 /* shift */
|
|
#define CTRL 0x0010 /* control*/
|
|
#define EXT 0x0020 /* extended scan code 0xe0 */
|
|
#define ESC 0x0040 /* escape key press */
|
|
#define E1 0x0080 /* extended scan code 0xe1 */
|
|
#define BRK 0x0100 /* make break flag for keyboard */
|
|
#define ALT 0x0200 /* right alt */
|
|
|
|
/* exports */
|
|
|
|
/**
|
|
* Flush all buffer from keyboard controller to host.
|
|
*/
|
|
void i8042_flush(void);
|
|
|
|
/**
|
|
* Disables the keyboard so that key strokes no longer generate scancodes to
|
|
* the host.
|
|
*
|
|
* @return 0 if ok, -1 if keyboard input was found while disabling
|
|
*/
|
|
int i8042_disable(void);
|
|
|
|
#endif /* _I8042_H_ */
|