mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
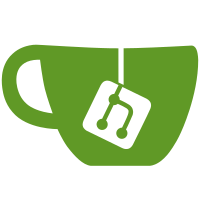
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
86 lines
2.1 KiB
C
86 lines
2.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* (C) Copyright 2015
|
|
* Texas Instruments Incorporated, <www.ti.com>
|
|
*/
|
|
|
|
#ifndef _DMA_H_
|
|
#define _DMA_H_
|
|
|
|
/*
|
|
* enum dma_direction - dma transfer direction indicator
|
|
* @DMA_MEM_TO_MEM: Memcpy mode
|
|
* @DMA_MEM_TO_DEV: From Memory to Device
|
|
* @DMA_DEV_TO_MEM: From Device to Memory
|
|
* @DMA_DEV_TO_DEV: From Device to Device
|
|
*/
|
|
enum dma_direction {
|
|
DMA_MEM_TO_MEM,
|
|
DMA_MEM_TO_DEV,
|
|
DMA_DEV_TO_MEM,
|
|
DMA_DEV_TO_DEV,
|
|
};
|
|
|
|
#define DMA_SUPPORTS_MEM_TO_MEM BIT(0)
|
|
#define DMA_SUPPORTS_MEM_TO_DEV BIT(1)
|
|
#define DMA_SUPPORTS_DEV_TO_MEM BIT(2)
|
|
#define DMA_SUPPORTS_DEV_TO_DEV BIT(3)
|
|
|
|
/*
|
|
* struct dma_ops - Driver model DMA operations
|
|
*
|
|
* The uclass interface is implemented by all DMA devices which use
|
|
* driver model.
|
|
*/
|
|
struct dma_ops {
|
|
/*
|
|
* Get the current timer count
|
|
*
|
|
* @dev: The DMA device
|
|
* @direction: direction of data transfer should be one from
|
|
enum dma_direction
|
|
* @dst: Destination pointer
|
|
* @src: Source pointer
|
|
* @len: Length of the data to be copied.
|
|
* @return: 0 if OK, -ve on error
|
|
*/
|
|
int (*transfer)(struct udevice *dev, int direction, void *dst,
|
|
void *src, size_t len);
|
|
};
|
|
|
|
/*
|
|
* struct dma_dev_priv - information about a device used by the uclass
|
|
*
|
|
* @supported: mode of transfers that DMA can support, should be
|
|
* one/multiple of DMA_SUPPORTS_*
|
|
*/
|
|
struct dma_dev_priv {
|
|
u32 supported;
|
|
};
|
|
|
|
/*
|
|
* dma_get_device - get a DMA device which supports transfer
|
|
* type of transfer_type
|
|
*
|
|
* @transfer_type - transfer type should be one/multiple of
|
|
* DMA_SUPPORTS_*
|
|
* @devp - udevice pointer to return the found device
|
|
* @return - will return on success and devp will hold the
|
|
* pointer to the device
|
|
*/
|
|
int dma_get_device(u32 transfer_type, struct udevice **devp);
|
|
|
|
/*
|
|
* dma_memcpy - try to use DMA to do a mem copy which will be
|
|
* much faster than CPU mem copy
|
|
*
|
|
* @dst - destination pointer
|
|
* @src - souce pointer
|
|
* @len - data length to be copied
|
|
* @return - on successful transfer returns no of bytes
|
|
transferred and on failure return error code.
|
|
*/
|
|
int dma_memcpy(void *dst, void *src, size_t len);
|
|
|
|
#endif /* _DMA_H_ */
|