mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
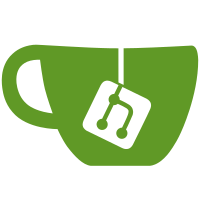
At present the panel can be turned on but not off, and the brightness cannot be controlled at run-time. Add a new API function to both the panel and backlight uclasses to handle this. Enhance the PWM backlight driver to deal with custom levels properly and allow the backlight to be turned on and off. Update the test to cover thes new features. Signed-off-by: Simon Glass <sjg@chromium.org>
120 lines
2.9 KiB
C
120 lines
2.9 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright (c) 2016 Google, Inc
|
|
* Written by Simon Glass <sjg@chromium.org>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <backlight.h>
|
|
#include <dm.h>
|
|
#include <panel.h>
|
|
#include <asm/gpio.h>
|
|
#include <power/regulator.h>
|
|
|
|
struct simple_panel_priv {
|
|
struct udevice *reg;
|
|
struct udevice *backlight;
|
|
struct gpio_desc enable;
|
|
};
|
|
|
|
static int simple_panel_enable_backlight(struct udevice *dev)
|
|
{
|
|
struct simple_panel_priv *priv = dev_get_priv(dev);
|
|
int ret;
|
|
|
|
debug("%s: start, backlight = '%s'\n", __func__, priv->backlight->name);
|
|
dm_gpio_set_value(&priv->enable, 1);
|
|
ret = backlight_enable(priv->backlight);
|
|
debug("%s: done, ret = %d\n", __func__, ret);
|
|
if (ret)
|
|
return ret;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int simple_panel_set_backlight(struct udevice *dev, int percent)
|
|
{
|
|
struct simple_panel_priv *priv = dev_get_priv(dev);
|
|
int ret;
|
|
|
|
debug("%s: start, backlight = '%s'\n", __func__, priv->backlight->name);
|
|
dm_gpio_set_value(&priv->enable, 1);
|
|
ret = backlight_set_brightness(priv->backlight, percent);
|
|
debug("%s: done, ret = %d\n", __func__, ret);
|
|
if (ret)
|
|
return ret;
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int simple_panel_ofdata_to_platdata(struct udevice *dev)
|
|
{
|
|
struct simple_panel_priv *priv = dev_get_priv(dev);
|
|
int ret;
|
|
|
|
if (IS_ENABLED(CONFIG_DM_REGULATOR)) {
|
|
ret = uclass_get_device_by_phandle(UCLASS_REGULATOR, dev,
|
|
"power-supply", &priv->reg);
|
|
if (ret) {
|
|
debug("%s: Warning: cannot get power supply: ret=%d\n",
|
|
__func__, ret);
|
|
if (ret != -ENOENT)
|
|
return ret;
|
|
}
|
|
}
|
|
ret = uclass_get_device_by_phandle(UCLASS_PANEL_BACKLIGHT, dev,
|
|
"backlight", &priv->backlight);
|
|
if (ret) {
|
|
debug("%s: Cannot get backlight: ret=%d\n", __func__, ret);
|
|
return log_ret(ret);
|
|
}
|
|
ret = gpio_request_by_name(dev, "enable-gpios", 0, &priv->enable,
|
|
GPIOD_IS_OUT);
|
|
if (ret) {
|
|
debug("%s: Warning: cannot get enable GPIO: ret=%d\n",
|
|
__func__, ret);
|
|
if (ret != -ENOENT)
|
|
return log_ret(ret);
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static int simple_panel_probe(struct udevice *dev)
|
|
{
|
|
struct simple_panel_priv *priv = dev_get_priv(dev);
|
|
int ret;
|
|
|
|
if (IS_ENABLED(CONFIG_DM_REGULATOR) && priv->reg) {
|
|
debug("%s: Enable regulator '%s'\n", __func__, priv->reg->name);
|
|
ret = regulator_set_enable(priv->reg, true);
|
|
if (ret)
|
|
return ret;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static const struct panel_ops simple_panel_ops = {
|
|
.enable_backlight = simple_panel_enable_backlight,
|
|
.set_backlight = simple_panel_set_backlight,
|
|
};
|
|
|
|
static const struct udevice_id simple_panel_ids[] = {
|
|
{ .compatible = "simple-panel" },
|
|
{ .compatible = "auo,b133xtn01" },
|
|
{ .compatible = "auo,b116xw03" },
|
|
{ .compatible = "auo,b133htn01" },
|
|
{ }
|
|
};
|
|
|
|
U_BOOT_DRIVER(simple_panel) = {
|
|
.name = "simple_panel",
|
|
.id = UCLASS_PANEL,
|
|
.of_match = simple_panel_ids,
|
|
.ops = &simple_panel_ops,
|
|
.ofdata_to_platdata = simple_panel_ofdata_to_platdata,
|
|
.probe = simple_panel_probe,
|
|
.priv_auto_alloc_size = sizeof(struct simple_panel_priv),
|
|
};
|