mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
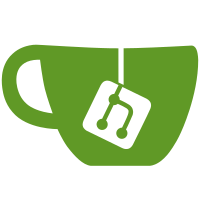
At present we go through various contortions to store the SPI slave's chip select in its private data. This only exists when the slave is active so must be set up when it is probed. Until the device is probed we don't actually know what chip select it will appear on. However, now that we can support per-child platform data, we can use that instead. This allows us to set up the chip select when the child is bound, and avoid the messy contortions. Unfortunately this is a fairly large change and it seems to be difficult to break it down further. Signed-off-by: Simon Glass <sjg@chromium.org>
128 lines
3.9 KiB
C
128 lines
3.9 KiB
C
/*
|
|
* Copyright (C) 2013 Google, Inc
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <dm.h>
|
|
#include <fdtdec.h>
|
|
#include <spi.h>
|
|
#include <spi_flash.h>
|
|
#include <dm/device-internal.h>
|
|
#include <dm/test.h>
|
|
#include <dm/uclass-internal.h>
|
|
#include <dm/ut.h>
|
|
#include <dm/util.h>
|
|
#include <asm/state.h>
|
|
|
|
/* Test that we can find buses and chip-selects */
|
|
static int dm_test_spi_find(struct dm_test_state *dms)
|
|
{
|
|
struct sandbox_state *state = state_get_current();
|
|
struct spi_slave *slave;
|
|
struct udevice *bus, *dev;
|
|
const int busnum = 0, cs = 0, mode = 0, speed = 1000000, cs_b = 1;
|
|
struct spi_cs_info info;
|
|
int of_offset;
|
|
|
|
ut_asserteq(-ENODEV, uclass_find_device_by_seq(UCLASS_SPI, busnum,
|
|
false, &bus));
|
|
|
|
/*
|
|
* spi_post_bind() will bind devices to chip selects. Check this then
|
|
* remove the emulation and the slave device.
|
|
*/
|
|
ut_asserteq(0, uclass_get_device_by_seq(UCLASS_SPI, busnum, &bus));
|
|
ut_assertok(spi_cs_info(bus, cs, &info));
|
|
of_offset = info.dev->of_offset;
|
|
device_remove(info.dev);
|
|
device_unbind(info.dev);
|
|
|
|
/*
|
|
* Even though the device is gone, the sandbox SPI drivers always
|
|
* reports that CS 0 is present
|
|
*/
|
|
ut_assertok(spi_cs_info(bus, cs, &info));
|
|
ut_asserteq_ptr(NULL, info.dev);
|
|
|
|
/* This finds nothing because we removed the device */
|
|
ut_asserteq(-ENODEV, spi_find_bus_and_cs(busnum, cs, &bus, &dev));
|
|
ut_asserteq(-ENODEV, spi_get_bus_and_cs(busnum, cs, speed, mode,
|
|
NULL, 0, &bus, &slave));
|
|
|
|
/*
|
|
* This forces the device to be re-added, but there is no emulation
|
|
* connected so the probe will fail. We require that bus is left
|
|
* alone on failure, and that the spi_get_bus_and_cs() does not add
|
|
* a 'partially-inited' device.
|
|
*/
|
|
ut_asserteq(-ENODEV, spi_find_bus_and_cs(busnum, cs, &bus, &dev));
|
|
ut_asserteq(-ENOENT, spi_get_bus_and_cs(busnum, cs, speed, mode,
|
|
"spi_flash_std", "name", &bus,
|
|
&slave));
|
|
sandbox_sf_unbind_emul(state_get_current(), busnum, cs);
|
|
ut_assertok(spi_cs_info(bus, cs, &info));
|
|
ut_asserteq_ptr(NULL, info.dev);
|
|
|
|
/* Add the emulation and try again */
|
|
ut_assertok(sandbox_sf_bind_emul(state, busnum, cs, bus, of_offset,
|
|
"name"));
|
|
ut_assertok(spi_find_bus_and_cs(busnum, cs, &bus, &dev));
|
|
ut_assertok(spi_get_bus_and_cs(busnum, cs, speed, mode,
|
|
"spi_flash_std", "name", &bus, &slave));
|
|
|
|
ut_assertok(spi_cs_info(bus, cs, &info));
|
|
ut_asserteq_ptr(info.dev, slave->dev);
|
|
|
|
/* We should be able to add something to another chip select */
|
|
ut_assertok(sandbox_sf_bind_emul(state, busnum, cs_b, bus, of_offset,
|
|
"name"));
|
|
ut_assertok(spi_get_bus_and_cs(busnum, cs_b, speed, mode,
|
|
"spi_flash_std", "name", &bus, &slave));
|
|
ut_assertok(spi_cs_info(bus, cs_b, &info));
|
|
ut_asserteq_ptr(info.dev, slave->dev);
|
|
|
|
/*
|
|
* Since we are about to destroy all devices, we must tell sandbox
|
|
* to forget the emulation device
|
|
*/
|
|
sandbox_sf_unbind_emul(state_get_current(), busnum, cs);
|
|
sandbox_sf_unbind_emul(state_get_current(), busnum, cs_b);
|
|
|
|
return 0;
|
|
}
|
|
DM_TEST(dm_test_spi_find, DM_TESTF_SCAN_PDATA | DM_TESTF_SCAN_FDT);
|
|
|
|
/* Test that sandbox SPI works correctly */
|
|
static int dm_test_spi_xfer(struct dm_test_state *dms)
|
|
{
|
|
struct spi_slave *slave;
|
|
struct udevice *bus;
|
|
const int busnum = 0, cs = 0, mode = 0;
|
|
const char dout[5] = {0x9f};
|
|
unsigned char din[5];
|
|
|
|
ut_assertok(spi_get_bus_and_cs(busnum, cs, 1000000, mode, NULL, 0,
|
|
&bus, &slave));
|
|
ut_assertok(spi_claim_bus(slave));
|
|
ut_assertok(spi_xfer(slave, 40, dout, din,
|
|
SPI_XFER_BEGIN | SPI_XFER_END));
|
|
ut_asserteq(0xff, din[0]);
|
|
ut_asserteq(0x20, din[1]);
|
|
ut_asserteq(0x20, din[2]);
|
|
ut_asserteq(0x15, din[3]);
|
|
spi_release_bus(slave);
|
|
|
|
/*
|
|
* Since we are about to destroy all devices, we must tell sandbox
|
|
* to forget the emulation device
|
|
*/
|
|
#ifdef CONFIG_DM_SPI_FLASH
|
|
sandbox_sf_unbind_emul(state_get_current(), busnum, cs);
|
|
#endif
|
|
|
|
return 0;
|
|
}
|
|
DM_TEST(dm_test_spi_xfer, DM_TESTF_SCAN_PDATA | DM_TESTF_SCAN_FDT);
|