mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
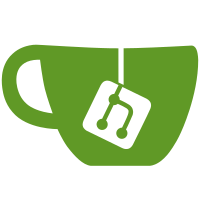
To use the PCIe driver, its controller memory and the PCIe regions need to get mapped in the MMU. Otherwise these areas can't be accessed. Signed-off-by: Stefan Roese <sr@denx.de> Cc: Nadav Haklai <nadavh@marvell.com> Cc: Neta Zur Hershkovits <neta@marvell.com> Cc: Kostya Porotchkin <kostap@marvell.com> Cc: Omri Itach <omrii@marvell.com> Cc: Igal Liberman <igall@marvell.com> Cc: Haim Boot <hayim@marvell.com> Cc: Hanna Hawa <hannah@marvell.com>
81 lines
1.8 KiB
C
81 lines
1.8 KiB
C
/*
|
|
* Copyright (C) 2016 Stefan Roese <sr@denx.de>
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <dm.h>
|
|
#include <fdtdec.h>
|
|
#include <libfdt.h>
|
|
#include <asm/io.h>
|
|
#include <asm/system.h>
|
|
#include <asm/arch/cpu.h>
|
|
#include <asm/arch/soc.h>
|
|
#include <asm/armv8/mmu.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
/* Armada 7k/8k */
|
|
#define MVEBU_RFU_BASE (MVEBU_REGISTER(0x6f0000))
|
|
#define RFU_GLOBAL_SW_RST (MVEBU_RFU_BASE + 0x84)
|
|
#define RFU_SW_RESET_OFFSET 0
|
|
|
|
static struct mm_region mvebu_mem_map[] = {
|
|
{
|
|
/* RAM */
|
|
.phys = 0x0UL,
|
|
.virt = 0x0UL,
|
|
.size = 0x80000000UL,
|
|
.attrs = PTE_BLOCK_MEMTYPE(MT_NORMAL) |
|
|
PTE_BLOCK_INNER_SHARE
|
|
},
|
|
{
|
|
/* SRAM, MMIO regions - AP806 region */
|
|
.phys = 0xf0000000UL,
|
|
.virt = 0xf0000000UL,
|
|
.size = 0x01000000UL, /* 16MiB internal registers */
|
|
.attrs = PTE_BLOCK_MEMTYPE(MT_DEVICE_NGNRNE) |
|
|
PTE_BLOCK_NON_SHARE
|
|
},
|
|
{
|
|
/* SRAM, MMIO regions - CP110 master region */
|
|
.phys = 0xf2000000UL,
|
|
.virt = 0xf2000000UL,
|
|
.size = 0x02000000UL, /* 32MiB internal registers */
|
|
.attrs = PTE_BLOCK_MEMTYPE(MT_DEVICE_NGNRNE) |
|
|
PTE_BLOCK_NON_SHARE
|
|
},
|
|
{
|
|
/* SRAM, MMIO regions - CP110 slave region */
|
|
.phys = 0xf4000000UL,
|
|
.virt = 0xf4000000UL,
|
|
.size = 0x02000000UL, /* 32MiB internal registers */
|
|
.attrs = PTE_BLOCK_MEMTYPE(MT_DEVICE_NGNRNE) |
|
|
PTE_BLOCK_NON_SHARE
|
|
},
|
|
{
|
|
/* PCI regions */
|
|
.phys = 0xf8000000UL,
|
|
.virt = 0xf8000000UL,
|
|
.size = 0x08000000UL, /* 128MiB PCI space (master & slave) */
|
|
.attrs = PTE_BLOCK_MEMTYPE(MT_DEVICE_NGNRNE) |
|
|
PTE_BLOCK_NON_SHARE
|
|
},
|
|
{
|
|
/* List terminator */
|
|
0,
|
|
}
|
|
};
|
|
|
|
struct mm_region *mem_map = mvebu_mem_map;
|
|
|
|
void reset_cpu(ulong ignored)
|
|
{
|
|
u32 reg;
|
|
|
|
reg = readl(RFU_GLOBAL_SW_RST);
|
|
reg &= ~(1 << RFU_SW_RESET_OFFSET);
|
|
writel(reg, RFU_GLOBAL_SW_RST);
|
|
}
|