mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
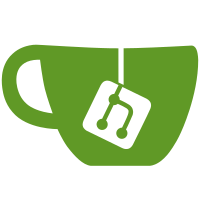
The problem is that timeout bits in WCR register were leaved unchanged. So previously set timeout value was applied and therefore 'reset' command takes any value up to two minutes, depending on previous watchdog settings, instead of minimal 0.5 seconds. Signed-off-by: Andrey Skvortsov <andrej.skvortzov@gmail.com>
56 lines
1.2 KiB
C
56 lines
1.2 KiB
C
/*
|
|
* watchdog.c - driver for i.mx on-chip watchdog
|
|
*
|
|
* Licensed under the GPL-2 or later.
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <asm/io.h>
|
|
#include <watchdog.h>
|
|
#include <asm/arch/imx-regs.h>
|
|
#include <fsl_wdog.h>
|
|
|
|
#ifdef CONFIG_IMX_WATCHDOG
|
|
void hw_watchdog_reset(void)
|
|
{
|
|
struct watchdog_regs *wdog = (struct watchdog_regs *)WDOG1_BASE_ADDR;
|
|
|
|
writew(0x5555, &wdog->wsr);
|
|
writew(0xaaaa, &wdog->wsr);
|
|
}
|
|
|
|
void hw_watchdog_init(void)
|
|
{
|
|
struct watchdog_regs *wdog = (struct watchdog_regs *)WDOG1_BASE_ADDR;
|
|
u16 timeout;
|
|
|
|
/*
|
|
* The timer watchdog can be set between
|
|
* 0.5 and 128 Seconds. If not defined
|
|
* in configuration file, sets 128 Seconds
|
|
*/
|
|
#ifndef CONFIG_WATCHDOG_TIMEOUT_MSECS
|
|
#define CONFIG_WATCHDOG_TIMEOUT_MSECS 128000
|
|
#endif
|
|
timeout = (CONFIG_WATCHDOG_TIMEOUT_MSECS / 500) - 1;
|
|
writew(WCR_WDZST | WCR_WDBG | WCR_WDE | WCR_WDT | WCR_SRS |
|
|
SET_WCR_WT(timeout), &wdog->wcr);
|
|
hw_watchdog_reset();
|
|
}
|
|
#endif
|
|
|
|
void reset_cpu(ulong addr)
|
|
{
|
|
struct watchdog_regs *wdog = (struct watchdog_regs *)WDOG1_BASE_ADDR;
|
|
|
|
clrsetbits_le16(&wdog->wcr, WCR_WT_MSK, WCR_WDE);
|
|
|
|
writew(0x5555, &wdog->wsr);
|
|
writew(0xaaaa, &wdog->wsr); /* load minimum 1/2 second timeout */
|
|
while (1) {
|
|
/*
|
|
* spin for .5 seconds before reset
|
|
*/
|
|
}
|
|
}
|