mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-06-09 23:36:03 +09:00
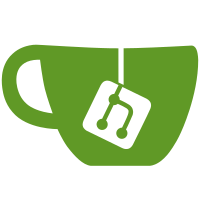
When U-Boot started using SPDX tags we were among the early adopters and there weren't a lot of other examples to borrow from. So we picked the area of the file that usually had a full license text and replaced it with an appropriate SPDX-License-Identifier: entry. Since then, the Linux Kernel has adopted SPDX tags and they place it as the very first line in a file (except where shebangs are used, then it's second line) and with slightly different comment styles than us. In part due to community overlap, in part due to better tag visibility and in part for other minor reasons, switch over to that style. This commit changes all instances where we have a single declared license in the tag as both the before and after are identical in tag contents. There's also a few places where I found we did not have a tag and have introduced one. Signed-off-by: Tom Rini <trini@konsulko.com>
118 lines
2.6 KiB
C
118 lines
2.6 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* Copyright (c) 2013 Google, Inc
|
|
*/
|
|
|
|
#ifndef __SANDBOX_SDL_H
|
|
#define __SANDBOX_SDL_H
|
|
|
|
#include <errno.h>
|
|
|
|
#ifdef CONFIG_SANDBOX_SDL
|
|
|
|
/**
|
|
* sandbox_sdl_init_display() - Set up SDL video ready for use
|
|
*
|
|
* @width: Window width in pixels
|
|
* @height Window height in pixels
|
|
* @log2_bpp: Log to base 2 of the number of bits per pixel. So a 32bpp
|
|
* display will pass 5, since 2*5 = 32
|
|
* @return 0 if OK, -ENODEV if no device, -EIO if SDL failed to initialize
|
|
* and -EPERM if the video failed to come up.
|
|
*/
|
|
int sandbox_sdl_init_display(int width, int height, int log2_bpp);
|
|
|
|
/**
|
|
* sandbox_sdl_sync() - Sync current U-Boot LCD frame buffer to SDL
|
|
*
|
|
* This must be called periodically to update the screen for SDL so that the
|
|
* user can see it.
|
|
*
|
|
* @lcd_base: Base of frame buffer
|
|
* @return 0 if screen was updated, -ENODEV is there is no screen.
|
|
*/
|
|
int sandbox_sdl_sync(void *lcd_base);
|
|
|
|
/**
|
|
* sandbox_sdl_scan_keys() - scan for pressed keys
|
|
*
|
|
* Works out which keys are pressed and returns a list
|
|
*
|
|
* @key: Array to receive keycodes
|
|
* @max_keys: Size of array
|
|
* @return number of keycodes found, 0 if none, -ENODEV if no keyboard
|
|
*/
|
|
int sandbox_sdl_scan_keys(int key[], int max_keys);
|
|
|
|
/**
|
|
* sandbox_sdl_key_pressed() - check if a particular key is pressed
|
|
*
|
|
* @keycode: Keycode to check (KEY_... - see include/linux/input.h
|
|
* @return 0 if pressed, -ENOENT if not pressed. -ENODEV if keybord not
|
|
* available,
|
|
*/
|
|
int sandbox_sdl_key_pressed(int keycode);
|
|
|
|
/**
|
|
* sandbox_sdl_sound_start() - start playing a sound
|
|
*
|
|
* @frequency: Frequency of sounds in Hertz
|
|
* @return 0 if OK, -ENODEV if no sound is available
|
|
*/
|
|
int sandbox_sdl_sound_start(uint frequency);
|
|
|
|
/**
|
|
* sandbox_sdl_sound_stop() - stop playing a sound
|
|
*
|
|
* @return 0 if OK, -ENODEV if no sound is available
|
|
*/
|
|
int sandbox_sdl_sound_stop(void);
|
|
|
|
/**
|
|
* sandbox_sdl_sound_init() - set up the sound system
|
|
*
|
|
* @return 0 if OK, -ENODEV if no sound is available
|
|
*/
|
|
int sandbox_sdl_sound_init(void);
|
|
|
|
#else
|
|
static inline int sandbox_sdl_init_display(int width, int height,
|
|
int log2_bpp)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int sandbox_sdl_sync(void *lcd_base)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int sandbox_sdl_scan_keys(int key[], int max_keys)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int sandbox_sdl_key_pressed(int keycode)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int sandbox_sdl_sound_start(uint frequency)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int sandbox_sdl_sound_stop(void)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
static inline int sandbox_sdl_sound_init(void)
|
|
{
|
|
return -ENODEV;
|
|
}
|
|
|
|
#endif
|
|
|
|
#endif
|