mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-05 18:56:15 +09:00
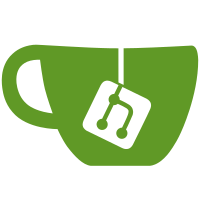
Linux booting protocol mandates that register "a0" contains the hartid. However, U-Boot can not pass the hartid via a0 during standard UEFI protocol. DT nodes are commonly used to pass such information to the OS. Add a DT node under chosen node to indicate the boot hartid. EFI stub in Linux kernel will parse this node and pass it to the real kernel in "a0" before jumping to it. Signed-off-by: Atish Patra <atish.patra@wdc.com> Reviewed-by: Rick Chen <rick@andestech.com> Reviewed-by: Bin Meng <bmeng.cn@gmail.com> Tested-by: Heinrich Schuchardt <xypron.glpk@gmx.de> Tested-by: Bin Meng <bmeng.cn@gmail.com>
162 lines
3.6 KiB
C
162 lines
3.6 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright (C) 2011 Andes Technology Corporation
|
|
* Shawn Lin, Andes Technology Corporation <nobuhiro@andestech.com>
|
|
* Macpaul Lin, Andes Technology Corporation <macpaul@andestech.com>
|
|
* Rick Chen, Andes Technology Corporation <rick@andestech.com>
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <command.h>
|
|
#include <dm.h>
|
|
#include <fdt_support.h>
|
|
#include <hang.h>
|
|
#include <dm/root.h>
|
|
#include <image.h>
|
|
#include <asm/byteorder.h>
|
|
#include <asm/csr.h>
|
|
#include <asm/smp.h>
|
|
#include <dm/device.h>
|
|
#include <dm/root.h>
|
|
#include <u-boot/zlib.h>
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
__weak void board_quiesce_devices(void)
|
|
{
|
|
}
|
|
|
|
int arch_fixup_fdt(void *blob)
|
|
{
|
|
#ifdef CONFIG_EFI_LOADER
|
|
int err;
|
|
u32 size;
|
|
int chosen_offset;
|
|
|
|
size = fdt_totalsize(blob);
|
|
err = fdt_open_into(blob, blob, size + 32);
|
|
if (err < 0) {
|
|
printf("Device Tree can't be expanded to accommodate new node");
|
|
return err;
|
|
}
|
|
chosen_offset = fdt_path_offset(blob, "/chosen");
|
|
if (chosen_offset < 0) {
|
|
err = fdt_add_subnode(blob, 0, "chosen");
|
|
if (err < 0) {
|
|
printf("chosen node can not be added\n");
|
|
return err;
|
|
}
|
|
}
|
|
/* Overwrite the boot-hartid as U-Boot is the last stage BL */
|
|
fdt_setprop_u32(blob, chosen_offset, "boot-hartid", gd->arch.boot_hart);
|
|
#endif
|
|
return 0;
|
|
}
|
|
|
|
/**
|
|
* announce_and_cleanup() - Print message and prepare for kernel boot
|
|
*
|
|
* @fake: non-zero to do everything except actually boot
|
|
*/
|
|
static void announce_and_cleanup(int fake)
|
|
{
|
|
printf("\nStarting kernel ...%s\n\n", fake ?
|
|
"(fake run for tracing)" : "");
|
|
bootstage_mark_name(BOOTSTAGE_ID_BOOTM_HANDOFF, "start_kernel");
|
|
#ifdef CONFIG_BOOTSTAGE_FDT
|
|
bootstage_fdt_add_report();
|
|
#endif
|
|
#ifdef CONFIG_BOOTSTAGE_REPORT
|
|
bootstage_report();
|
|
#endif
|
|
|
|
#ifdef CONFIG_USB_DEVICE
|
|
udc_disconnect();
|
|
#endif
|
|
|
|
board_quiesce_devices();
|
|
|
|
/*
|
|
* Call remove function of all devices with a removal flag set.
|
|
* This may be useful for last-stage operations, like cancelling
|
|
* of DMA operation or releasing device internal buffers.
|
|
*/
|
|
dm_remove_devices_flags(DM_REMOVE_ACTIVE_ALL);
|
|
|
|
cleanup_before_linux();
|
|
}
|
|
|
|
static void boot_prep_linux(bootm_headers_t *images)
|
|
{
|
|
if (IMAGE_ENABLE_OF_LIBFDT && images->ft_len) {
|
|
#ifdef CONFIG_OF_LIBFDT
|
|
debug("using: FDT\n");
|
|
if (image_setup_linux(images)) {
|
|
printf("FDT creation failed! hanging...");
|
|
hang();
|
|
}
|
|
#endif
|
|
} else {
|
|
printf("Device tree not found or missing FDT support\n");
|
|
hang();
|
|
}
|
|
}
|
|
|
|
static void boot_jump_linux(bootm_headers_t *images, int flag)
|
|
{
|
|
void (*kernel)(ulong hart, void *dtb);
|
|
int fake = (flag & BOOTM_STATE_OS_FAKE_GO);
|
|
#ifdef CONFIG_SMP
|
|
int ret;
|
|
#endif
|
|
|
|
kernel = (void (*)(ulong, void *))images->ep;
|
|
|
|
bootstage_mark(BOOTSTAGE_ID_RUN_OS);
|
|
|
|
debug("## Transferring control to kernel (at address %08lx) ...\n",
|
|
(ulong)kernel);
|
|
|
|
announce_and_cleanup(fake);
|
|
|
|
if (!fake) {
|
|
if (IMAGE_ENABLE_OF_LIBFDT && images->ft_len) {
|
|
#ifdef CONFIG_SMP
|
|
ret = smp_call_function(images->ep,
|
|
(ulong)images->ft_addr, 0, 0);
|
|
if (ret)
|
|
hang();
|
|
#endif
|
|
kernel(gd->arch.boot_hart, images->ft_addr);
|
|
}
|
|
}
|
|
}
|
|
|
|
int do_bootm_linux(int flag, int argc, char * const argv[],
|
|
bootm_headers_t *images)
|
|
{
|
|
/* No need for those on RISC-V */
|
|
if (flag & BOOTM_STATE_OS_BD_T || flag & BOOTM_STATE_OS_CMDLINE)
|
|
return -1;
|
|
|
|
if (flag & BOOTM_STATE_OS_PREP) {
|
|
boot_prep_linux(images);
|
|
return 0;
|
|
}
|
|
|
|
if (flag & (BOOTM_STATE_OS_GO | BOOTM_STATE_OS_FAKE_GO)) {
|
|
boot_jump_linux(images, flag);
|
|
return 0;
|
|
}
|
|
|
|
boot_prep_linux(images);
|
|
boot_jump_linux(images, flag);
|
|
return 0;
|
|
}
|
|
|
|
int do_bootm_vxworks(int flag, int argc, char * const argv[],
|
|
bootm_headers_t *images)
|
|
{
|
|
return do_bootm_linux(flag, argc, argv, images);
|
|
}
|