mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-29 14:23:44 +09:00
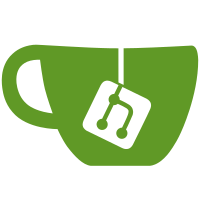
This patch brings the files from Linux kernel (linux-stable/linux-5.1.y SHA1: 5752b50477da)to provide clocks support as it is used on the Linux kernel with Common Clock Framework [CCF] setup. The directory structure has been preserved. The ported code only supports reading information from PLL, MUX, Divider, etc and enabling/disabling the clocks USDHCx/ECSPIx depending on used bus. Moreover, it is agnostic to the alias numbering as the information about the clock is read from the device tree. One needs to pay attention to the comments indicating necessary for U-Boot's driver model changes. If needed, the code can be extended to support the "set" part of the clock management. Signed-off-by: Lukasz Majewski <lukma@denx.de>
70 lines
1.9 KiB
C
70 lines
1.9 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright (C) 2019 DENX Software Engineering
|
|
* Lukasz Majewski, DENX Software Engineering, lukma@denx.de
|
|
*/
|
|
#ifndef __MACH_IMX_CLK_H
|
|
#define __MACH_IMX_CLK_H
|
|
|
|
#include <linux/clk-provider.h>
|
|
|
|
enum imx_pllv3_type {
|
|
IMX_PLLV3_GENERIC,
|
|
IMX_PLLV3_SYS,
|
|
IMX_PLLV3_USB,
|
|
IMX_PLLV3_USB_VF610,
|
|
IMX_PLLV3_AV,
|
|
IMX_PLLV3_ENET,
|
|
IMX_PLLV3_ENET_IMX7,
|
|
IMX_PLLV3_SYS_VF610,
|
|
IMX_PLLV3_DDR_IMX7,
|
|
};
|
|
|
|
struct clk *clk_register_gate2(struct device *dev, const char *name,
|
|
const char *parent_name, unsigned long flags,
|
|
void __iomem *reg, u8 bit_idx, u8 cgr_val,
|
|
u8 clk_gate_flags);
|
|
|
|
struct clk *imx_clk_pllv3(enum imx_pllv3_type type, const char *name,
|
|
const char *parent_name, void __iomem *base,
|
|
u32 div_mask);
|
|
|
|
static inline struct clk *imx_clk_gate2(const char *name, const char *parent,
|
|
void __iomem *reg, u8 shift)
|
|
{
|
|
return clk_register_gate2(NULL, name, parent, CLK_SET_RATE_PARENT, reg,
|
|
shift, 0x3, 0);
|
|
}
|
|
|
|
static inline struct clk *imx_clk_fixed_factor(const char *name,
|
|
const char *parent, unsigned int mult, unsigned int div)
|
|
{
|
|
return clk_register_fixed_factor(NULL, name, parent,
|
|
CLK_SET_RATE_PARENT, mult, div);
|
|
}
|
|
|
|
static inline struct clk *imx_clk_divider(const char *name, const char *parent,
|
|
void __iomem *reg, u8 shift, u8 width)
|
|
{
|
|
return clk_register_divider(NULL, name, parent, CLK_SET_RATE_PARENT,
|
|
reg, shift, width, 0);
|
|
}
|
|
|
|
struct clk *imx_clk_pfd(const char *name, const char *parent_name,
|
|
void __iomem *reg, u8 idx);
|
|
|
|
struct clk *imx_clk_fixup_mux(const char *name, void __iomem *reg,
|
|
u8 shift, u8 width, const char * const *parents,
|
|
int num_parents, void (*fixup)(u32 *val));
|
|
|
|
static inline struct clk *imx_clk_mux(const char *name, void __iomem *reg,
|
|
u8 shift, u8 width, const char * const *parents,
|
|
int num_parents)
|
|
{
|
|
return clk_register_mux(NULL, name, parents, num_parents,
|
|
CLK_SET_RATE_NO_REPARENT, reg, shift,
|
|
width, 0);
|
|
}
|
|
|
|
#endif /* __MACH_IMX_CLK_H */
|