mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-16 16:16:16 +09:00
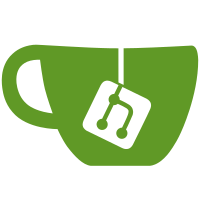
In axs103 v1.1 procedure to kick-start slave cores has changed quite a bit compared t previous implementation. In particular: * We used to have a generic START bit for all cores selected by CORE_SEL mask. But now we don't touch CORE_SEL at all because we have a dedicated START bit for each core: bit 0: Core 0 (master) bit 1: Core 1 (slave) * Now there's no need to select "manual" mode of core start Additional challenge for us is how to tell which axs103 firmware we're dealing with. For now we'll rely on ARC core version which was bumped from 2.1c to 3.0. Signed-off-by: Alexey Brodkin <abrodkin@synopsys.com>
94 lines
2.0 KiB
C
94 lines
2.0 KiB
C
/*
|
|
* Copyright (C) 2013-2014 Synopsys, Inc. All rights reserved.
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <dwmmc.h>
|
|
#include <malloc.h>
|
|
#include <asm/arcregs.h>
|
|
#include "axs10x.h"
|
|
|
|
DECLARE_GLOBAL_DATA_PTR;
|
|
|
|
int board_mmc_init(bd_t *bis)
|
|
{
|
|
struct dwmci_host *host = NULL;
|
|
|
|
host = malloc(sizeof(struct dwmci_host));
|
|
if (!host) {
|
|
printf("dwmci_host malloc fail!\n");
|
|
return 1;
|
|
}
|
|
|
|
memset(host, 0, sizeof(struct dwmci_host));
|
|
host->name = "Synopsys Mobile storage";
|
|
host->ioaddr = (void *)ARC_DWMMC_BASE;
|
|
host->buswidth = 4;
|
|
host->dev_index = 0;
|
|
host->bus_hz = 50000000;
|
|
|
|
add_dwmci(host, host->bus_hz / 2, 400000);
|
|
|
|
return 0;
|
|
}
|
|
|
|
#define AXS_MB_CREG 0xE0011000
|
|
|
|
int board_early_init_f(void)
|
|
{
|
|
if (readl((void __iomem *)AXS_MB_CREG + 0x234) & (1 << 28))
|
|
gd->board_type = AXS_MB_V3;
|
|
else
|
|
gd->board_type = AXS_MB_V2;
|
|
|
|
return 0;
|
|
}
|
|
|
|
#ifdef CONFIG_ISA_ARCV2
|
|
#define RESET_VECTOR_ADDR 0x0
|
|
|
|
void smp_set_core_boot_addr(unsigned long addr, int corenr)
|
|
{
|
|
/* All cores have reset vector pointing to 0 */
|
|
writel(addr, (void __iomem *)RESET_VECTOR_ADDR);
|
|
|
|
/* Make sure other cores see written value in memory */
|
|
flush_dcache_all();
|
|
}
|
|
|
|
void smp_kick_all_cpus(void)
|
|
{
|
|
/* CPU start CREG */
|
|
#define AXC003_CREG_CPU_START 0xF0001400
|
|
/* Bits positions in CPU start CREG */
|
|
#define BITS_START 0
|
|
#define BITS_START_MODE 4
|
|
#define BITS_CORE_SEL 9
|
|
|
|
/*
|
|
* In axs103 v1.1 START bits semantics has changed quite a bit.
|
|
* We used to have a generic START bit for all cores selected by CORE_SEL mask.
|
|
* But now we don't touch CORE_SEL at all because we have a dedicated START bit
|
|
* for each core:
|
|
* bit 0: Core 0 (master)
|
|
* bit 1: Core 1 (slave)
|
|
*/
|
|
#define BITS_START_CORE1 1
|
|
|
|
#define ARCVER_HS38_3_0 0x53
|
|
|
|
int core_family = read_aux_reg(ARC_AUX_IDENTITY) & 0xff;
|
|
int cmd = readl((void __iomem *)AXC003_CREG_CPU_START);
|
|
|
|
if (core_family < ARCVER_HS38_3_0) {
|
|
cmd |= (1 << BITS_CORE_SEL) | (1 << BITS_START);
|
|
cmd &= ~(1 << BITS_START_MODE);
|
|
} else {
|
|
cmd |= (1 << BITS_START_CORE1);
|
|
}
|
|
writel(cmd, (void __iomem *)AXC003_CREG_CPU_START);
|
|
}
|
|
#endif
|