mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-26 21:03:44 +09:00
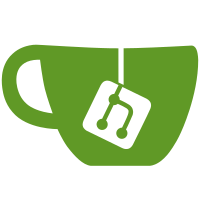
There was for long time no activity in the 8xx area. We need to go further and convert to Kconfig, but it turned out, nobody is interested anymore in 8xx, so remove it (with a heavy heart, knowing that I remove here the root of U-Boot). Signed-off-by: Heiko Schocher <hs@denx.de>
90 lines
2.6 KiB
C
90 lines
2.6 KiB
C
/*
|
|
* (C) Copyright 2007
|
|
* Gary Jennejohn, DENX Software Engineering, garyj@denx.de.
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
*/
|
|
/* sil680.c - ide support functions for the Sil0680A controller */
|
|
|
|
/*
|
|
* The following parameters must be defined in the configuration file
|
|
* of the target board:
|
|
*
|
|
* #define CONFIG_IDE_SIL680
|
|
*
|
|
* #define CONFIG_PCI_PNP
|
|
* NOTE it may also be necessary to define this if the default of 8 is
|
|
* incorrect for the target board (e.g. the sequoia board requires 0).
|
|
* #define CONFIG_SYS_PCI_CACHE_LINE_SIZE 0
|
|
*
|
|
* #define CONFIG_IDE
|
|
* #undef CONFIG_IDE_LED
|
|
* #undef CONFIG_IDE_RESET
|
|
* #define CONFIG_IDE_PREINIT
|
|
* #define CONFIG_SYS_IDE_MAXBUS 2 - modify to suit
|
|
* #define CONFIG_SYS_IDE_MAXDEVICE (CONFIG_SYS_IDE_MAXBUS*2) - modify to suit
|
|
* #define CONFIG_SYS_ATA_BASE_ADDR 0
|
|
* #define CONFIG_SYS_ATA_IDE0_OFFSET 0
|
|
* #define CONFIG_SYS_ATA_IDE1_OFFSET 0
|
|
* #define CONFIG_SYS_ATA_DATA_OFFSET 0
|
|
* #define CONFIG_SYS_ATA_REG_OFFSET 0
|
|
* #define CONFIG_SYS_ATA_ALT_OFFSET 0x0004
|
|
*
|
|
* The mapping for PCI IO-space.
|
|
* NOTE this is the value for the sequoia board. Modify to suit.
|
|
* #define CONFIG_SYS_PCI0_IO_SPACE 0xE8000000
|
|
*/
|
|
|
|
#include <common.h>
|
|
#include <ata.h>
|
|
#include <ide.h>
|
|
#include <pci.h>
|
|
|
|
extern ulong ide_bus_offset[CONFIG_SYS_IDE_MAXBUS];
|
|
|
|
int ide_preinit (void)
|
|
{
|
|
int status;
|
|
pci_dev_t devbusfn;
|
|
int l;
|
|
|
|
status = 1;
|
|
for (l = 0; l < CONFIG_SYS_IDE_MAXBUS; l++) {
|
|
ide_bus_offset[l] = -ATA_STATUS;
|
|
}
|
|
devbusfn = pci_find_device (0x1095, 0x0680, 0);
|
|
if (devbusfn != -1) {
|
|
status = 0;
|
|
|
|
pci_read_config_dword (devbusfn, PCI_BASE_ADDRESS_0,
|
|
(u32 *) &ide_bus_offset[0]);
|
|
ide_bus_offset[0] &= 0xfffffff8;
|
|
ide_bus_offset[0] += CONFIG_SYS_PCI0_IO_SPACE;
|
|
pci_read_config_dword (devbusfn, PCI_BASE_ADDRESS_2,
|
|
(u32 *) &ide_bus_offset[1]);
|
|
ide_bus_offset[1] &= 0xfffffff8;
|
|
ide_bus_offset[1] += CONFIG_SYS_PCI0_IO_SPACE;
|
|
/* init various things - taken from the Linux driver */
|
|
/* set PIO mode */
|
|
pci_write_config_byte(devbusfn, 0x80, 0x00);
|
|
pci_write_config_byte(devbusfn, 0x84, 0x00);
|
|
/* IDE0 */
|
|
pci_write_config_byte(devbusfn, 0xA1, 0x02);
|
|
pci_write_config_word(devbusfn, 0xA2, 0x328A);
|
|
pci_write_config_dword(devbusfn, 0xA4, 0x62DD62DD);
|
|
pci_write_config_dword(devbusfn, 0xA8, 0x43924392);
|
|
pci_write_config_dword(devbusfn, 0xAC, 0x40094009);
|
|
/* IDE1 */
|
|
pci_write_config_byte(devbusfn, 0xB1, 0x02);
|
|
pci_write_config_word(devbusfn, 0xB2, 0x328A);
|
|
pci_write_config_dword(devbusfn, 0xB4, 0x62DD62DD);
|
|
pci_write_config_dword(devbusfn, 0xB8, 0x43924392);
|
|
pci_write_config_dword(devbusfn, 0xBC, 0x40094009);
|
|
}
|
|
return (status);
|
|
}
|
|
|
|
void ide_set_reset (int flag) {
|
|
return;
|
|
}
|