mirror of
https://github.com/brain-hackers/u-boot-brain
synced 2024-07-06 03:06:16 +09:00
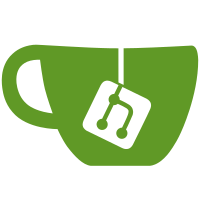
This commit adds functions issuing calls to secure monitor or hypervisore. This allows using services such as Power State Coordination Interface (PSCI) provided by firmware, e.g. ARM Trusted Firmware (ATF) The SMC call can destroy all registers declared temporary by the calling conventions. The clobber list is "x0..x17" because of this Signed-off-by: Sergey Temerkhanov <s.temerkhanov@gmail.com> Signed-off-by: Corey Minyard <cminyard@mvista.com> Signed-off-by: Radha Mohan Chintakuntla <rchintakuntla@cavium.com> Reviewed-by: Simon Glass <sjg@chromium.org> Tested-by: Mateusz Kulikowski <mateusz.kulikowski@gmail.com>
76 lines
1.5 KiB
C
76 lines
1.5 KiB
C
/**
|
|
* (C) Copyright 2014, Cavium Inc.
|
|
*
|
|
* SPDX-License-Identifier: GPL-2.0+
|
|
**/
|
|
|
|
#include <asm-offsets.h>
|
|
#include <config.h>
|
|
#include <version.h>
|
|
#include <asm/macro.h>
|
|
#include <asm/system.h>
|
|
|
|
/*
|
|
* Issue the hypervisor call
|
|
*
|
|
* x0~x7: input arguments
|
|
* x0~x3: output arguments
|
|
*/
|
|
void hvc_call(struct pt_regs *args)
|
|
{
|
|
asm volatile(
|
|
"ldr x0, %0\n"
|
|
"ldr x1, %1\n"
|
|
"ldr x2, %2\n"
|
|
"ldr x3, %3\n"
|
|
"ldr x4, %4\n"
|
|
"ldr x5, %5\n"
|
|
"ldr x6, %6\n"
|
|
"ldr x7, %7\n"
|
|
"hvc #0\n"
|
|
"str x0, %0\n"
|
|
"str x1, %1\n"
|
|
"str x2, %2\n"
|
|
"str x3, %3\n"
|
|
: "+m" (args->regs[0]), "+m" (args->regs[1]),
|
|
"+m" (args->regs[2]), "+m" (args->regs[3])
|
|
: "m" (args->regs[4]), "m" (args->regs[5]),
|
|
"m" (args->regs[6]), "m" (args->regs[7])
|
|
: "x0", "x1", "x2", "x3", "x4", "x5", "x6", "x7",
|
|
"x8", "x9", "x10", "x11", "x12", "x13", "x14", "x15",
|
|
"x16", "x17");
|
|
}
|
|
|
|
/*
|
|
* void smc_call(arg0, arg1...arg7)
|
|
*
|
|
* issue the secure monitor call
|
|
*
|
|
* x0~x7: input arguments
|
|
* x0~x3: output arguments
|
|
*/
|
|
|
|
void smc_call(struct pt_regs *args)
|
|
{
|
|
asm volatile(
|
|
"ldr x0, %0\n"
|
|
"ldr x1, %1\n"
|
|
"ldr x2, %2\n"
|
|
"ldr x3, %3\n"
|
|
"ldr x4, %4\n"
|
|
"ldr x5, %5\n"
|
|
"ldr x6, %6\n"
|
|
"smc #0\n"
|
|
"str x0, %0\n"
|
|
"str x1, %1\n"
|
|
"str x2, %2\n"
|
|
"str x3, %3\n"
|
|
: "+m" (args->regs[0]), "+m" (args->regs[1]),
|
|
"+m" (args->regs[2]), "+m" (args->regs[3])
|
|
: "m" (args->regs[4]), "m" (args->regs[5]),
|
|
"m" (args->regs[6])
|
|
: "x0", "x1", "x2", "x3", "x4", "x5", "x6", "x7",
|
|
"x8", "x9", "x10", "x11", "x12", "x13", "x14", "x15",
|
|
"x16", "x17");
|
|
}
|